


Data consistency guarantee measures in PHP instant killing system
Data consistency guarantee measures in PHP flash sale system
Abstract: With the rapid development of the Internet, flash sales in e-commerce activities are becoming more and more popular. . As an efficient and easy-to-develop open source programming language, PHP is widely used to develop various types of websites and systems. This article will introduce how to ensure data consistency in the PHP flash sale system, and give specific code examples to illustrate.
1. Background introduction
Flash sale activity refers to a marketing strategy for e-commerce websites to sell a certain product in limited quantities within a specific period of time. Since this activity involves a large number of users rushing to purchase the same product at the same time, it can easily lead to problems such as excessive system load, overselling, and dirty data. Therefore, it is very important to ensure data consistency in the PHP flash sale system to ensure order processing and data accuracy.
2. Optimistic locking mechanism
Optimistic locking is implemented through version number or timestamp. In the flash sale system, the inventory of each product can be used as the version number, the product inventory is updated after each rush, and the version number is compared to determine whether the rush is successful. The following is a sample code using the optimistic locking mechanism:
// 获取商品信息和库存 $sql = "SELECT stock FROM goods WHERE id = 1"; $result = $db->query($sql); $row = $result->fetch_assoc(); $stock = $row['stock']; // 判断库存是否足够 if ($stock > 0) { // 生成订单并更新库存 $sql = "INSERT INTO orders (goods_id) VALUES (1)"; $db->query($sql); $sql = "UPDATE goods SET stock = stock - 1 WHERE id = 1 AND stock = $stock"; $db->query($sql); if ($db->affected_rows == 0) { // 更新失败,抢购失败 } else { // 抢购成功 } } else { // 库存不足,抢购失败 }
By using the optimistic locking mechanism, the database pressure can be reduced while ensuring data consistency.
3. Distributed lock mechanism
Distributed lock is a mechanism designed to prevent multiple requests from operating shared resources at the same time. In the PHP flash sale system, distributed locks can be used to ensure that only one request can perform the snap-up operation at the same time. The following is a sample code that uses Redis to implement distributed locks:
// 加锁 $redis = new Redis(); $redis->connect('127.0.0.1', 6379); $lockKey = 'goods:1:lock'; $timeout = 10; $expire = time() + $timeout; while (true) { $isLock = $redis->setnx($lockKey, $expire); if ($isLock || ($redis->get($lockKey) < time() && $redis->getSet($lockKey, $expire) < time())) { // 加锁成功,执行抢购操作 // ... // 释放锁 $redis->del($lockKey); break; } }
By using the distributed lock mechanism, multiple requests can be prevented from rushing to purchase the same product at the same time, thus avoiding resource competition and data inconsistency.
4. Message Queue
The message queue is an asynchronous communication mechanism that can decouple the request processing process and the return results, avoiding the peak pressure of the system. In the PHP flash sale system, snap-up requests can be processed through message queues. The following is a sample code that uses RabbitMQ to implement a message queue:
// 生产者端代码 $exchangeName = 'seckill_exchange'; $queueName = 'seckill_queue'; $routingKey = 'seckill_key'; $connection = new AMQPStreamConnection('127.0.0.1', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->exchange_declare($exchangeName, 'direct', false, true, false); $channel->queue_declare($queueName, false, true, false, false); $channel->queue_bind($queueName, $exchangeName, $routingKey); $msgBody = 'User A wants to buy Goods 1'; $msg = new AMQPMessage($msgBody, ['delivery_mode' => AMQPMessage::DELIVERY_MODE_PERSISTENT]); $channel->basic_publish($msg, $exchangeName, $routingKey); $channel->close(); $connection->close(); // 消费者端代码 $callback = function ($msg) { // 处理抢购请求 // ... $msg->ack(); }; $connection = new AMQPStreamConnection('127.0.0.1', 5672, 'guest', 'guest'); $channel = $connection->channel(); $channel->queue_declare($queueName, false, true, false, false); $channel->basic_qos(null, 1, null); $channel->basic_consume($queueName, '', false, false, false, false, $callback); while (count($channel->callbacks)) { $channel->wait(); } $channel->close(); $connection->close();
By using the message queue, rush purchase requests can be processed asynchronously, reducing system load, and ensuring data consistency.
5. Summary
Data consistency in the PHP flash sale system is an important issue, involving order processing and data correctness. This article introduces three measures to ensure data consistency: optimistic locking, distributed locking and message queues, and gives specific code examples. In actual development, appropriate measures can be selected based on specific needs and business scenarios to ensure the stability and reliability of the flash sale system.
The above is the detailed content of Data consistency guarantee measures in PHP instant killing system. For more information, please follow other related articles on the PHP Chinese website!
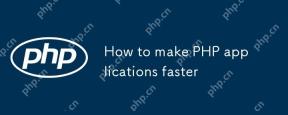
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
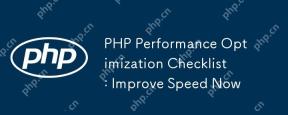
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
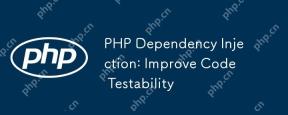
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
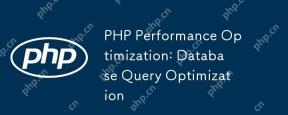
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi
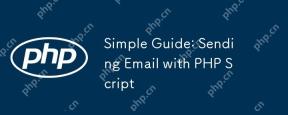
PHPisusedforsendingemailsduetoitsbuilt-inmail()functionandsupportivelibrarieslikePHPMailerandSwiftMailer.1)Usethemail()functionforbasicemails,butithaslimitations.2)EmployPHPMailerforadvancedfeatureslikeHTMLemailsandattachments.3)Improvedeliverability
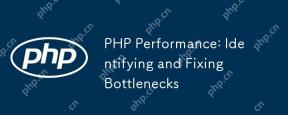
PHP performance bottlenecks can be solved through the following steps: 1) Use Xdebug or Blackfire for performance analysis to find out the problem; 2) Optimize database queries and use caches, such as APCu; 3) Use efficient functions such as array_filter to optimize array operations; 4) Configure OPcache for bytecode cache; 5) Optimize the front-end, such as reducing HTTP requests and optimizing pictures; 6) Continuously monitor and optimize performance. Through these methods, the performance of PHP applications can be significantly improved.
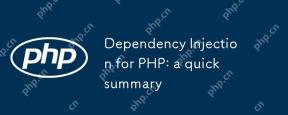
DependencyInjection(DI)inPHPisadesignpatternthatmanagesandreducesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itallowspassingdependencieslikedatabaseconnectionstoclassesasparameters,facilitatingeasiertestingandscalability.
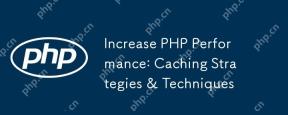
CachingimprovesPHPperformancebystoringresultsofcomputationsorqueriesforquickretrieval,reducingserverloadandenhancingresponsetimes.Effectivestrategiesinclude:1)Opcodecaching,whichstorescompiledPHPscriptsinmemorytoskipcompilation;2)DatacachingusingMemc


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
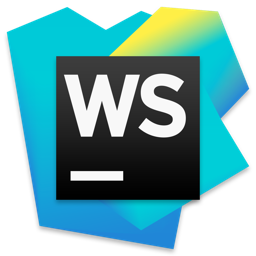
WebStorm Mac version
Useful JavaScript development tools
