


PHP development skills: How to implement data table association and query functions
PHP development skills: implementing data table association and query functions
In PHP development, it is often necessary to handle database-related operations, including associations between data tables and query. This article will introduce how to use PHP to implement the correlation and query functions of data tables, and provide specific code examples.
1. The concept of data table association
Data table association refers to connecting the records in two or more data tables through certain rules to obtain the data information of the associated table. Common data table association methods include one-to-one association, one-to-many association and many-to-many association.
- One-to-one association: There is a unique correspondence between two data tables, that is, one record in one data table only corresponds to one record in another data table. You can combine the two To achieve the primary key association of a data table.
- One-to-many association: One record in a data table has multiple corresponding records, which can be achieved by associating the primary keys and foreign keys of the two data tables.
- Many-to-many association: There is a many-to-many relationship between two data tables, which needs to be implemented through additional association tables. The records in the association table contain the primary keys of the two data tables and are used to establish the association between the two data tables.
2. Implementation method of data table association
- Use SQL statement for association query
You can combine two or more data tables through the JOIN operation of SQL statement Associate them to obtain the data information of the associated table.
For example, assuming there are two data tables user and order, which record user and order information respectively, you can implement a one-to-many related query through the following SQL statement:
SELECT user.name, order.order_no
FROM user
INNER JOIN order
ON user.id = order.user_id
Code example:
<?php $mysqli = new mysqli("localhost", "username", "password", "database"); // 检查连接是否成功 if ($mysqli->connect_errno) { echo "Failed to connect to MySQL: " . $mysqli->connect_error; exit(); } $sql = "SELECT user.name, order.order_no FROM user INNER JOIN order ON user.id = order.user_id"; $result = $mysqli->query($sql); if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { echo "User: " . $row["name"] . ", Order: " . $row["order_no"] . "<br>"; } } else { echo "No results found."; } $mysqli->close(); ?>
- Use ORM library for related queries
ORM (Object-Relational Mapping) is a technology that maps the database table structure to the object model. By using the ORM library, data table related queries can be more conveniently performed.
For example, you can use a commonly used ORM library in PHP such as Laravel's Eloquent to implement one-to-many related queries:
<?php use IlluminateDatabaseEloquentModel; class User extends Model { public function orders() { return $this->hasMany('AppOrder'); } } class Order extends Model { public function user() { return $this->belongsTo('AppUser'); } } // 查询用户及其订单信息 $user = User::with('orders')->find(1); foreach ($user->orders as $order) { echo "User: " . $user->name . ", Order: " . $order->order_no . "<br>"; } ?>
3. Summary
By studying this article , we learned about the concepts of data table associations and queries, and provided specific code examples. In PHP development, association and query functions between data tables can be easily realized through SQL statements and ORM libraries. Mastering these skills can improve the efficiency and flexibility of PHP development.
The above is the detailed content of PHP development skills: How to implement data table association and query functions. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
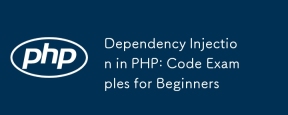
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
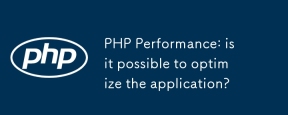
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
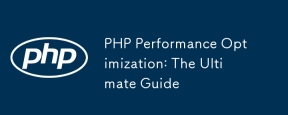
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
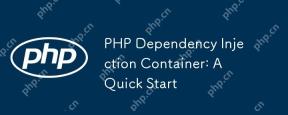
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
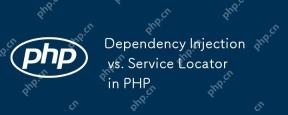
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
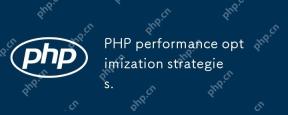
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
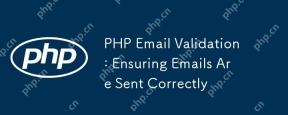
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
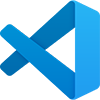
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
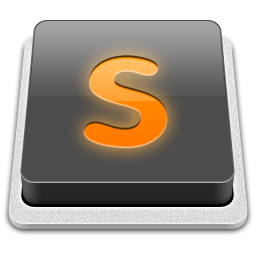
SublimeText3 Mac version
God-level code editing software (SublimeText3)
