How to use PHP to develop a simple online conference system
With the rapid development of the Internet, online conferencing has become important for more and more companies and individuals to communicate and collaborate. tool. In order to meet the needs of users, it is necessary to develop a simple and easy-to-use online conference system. This article will introduce how to use PHP to develop a simple online meeting system and provide specific code examples.
1. Requirements analysis and function sorting
Before starting development, we need to conduct needs analysis and function sorting first. A simple online conference system should have the following functions:
- User registration and login: Users can register an account and log in to the system.
- Create a meeting: Users can create a new meeting and fill in the basic information of the meeting, such as meeting title, start time, end time, location, etc.
- Invite participants: Users can invite other users to participate in the meeting.
- View meeting schedule: Users can view the meeting schedule they have participated in.
- Modify and delete meetings: Users can modify and delete meetings created by themselves.
- Participate in a meeting: Users can accept meeting invitations from others and join the meeting.
- Meeting Reminder: The system can send meeting reminders to users.
2. Database design and creation
The online conference system needs to use a database to store user information, meeting information and other data. We can use MySQL as the database and design the following table structure:
- User table (users): stores basic information of users, including user ID, user name, password and other fields.
- Meeting table (meetings): stores the basic information of the meeting, including meeting ID, meeting title, start time, end time, location and other fields.
- Participants table (participants): stores participant information, including participant ID, conference ID, participant ID and other fields.
3. Create PHP files and pages
-
Create a database connection file (db_connect.php) to connect to the MySQL database.
Code example:<?php $servername = "localhost"; $username = "root"; $password = "password"; $dbname = "meeting_system"; // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检测连接 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } ?>
-
Create a registration page (register.php) to implement the user registration function.
Code example:<?php include 'db_connect.php'; if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $password = $_POST["password"]; $sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')"; if ($conn->query($sql) === TRUE) { echo "注册成功"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } } $conn->close(); ?>
-
Create a login page (login.php) to implement the user login function.
Code example:<?php session_start(); include 'db_connect.php'; if ($_SERVER["REQUEST_METHOD"] == "POST") { $username = $_POST["username"]; $password = $_POST["password"]; $sql = "SELECT * FROM users WHERE username='$username' AND password='$password'"; $result = $conn->query($sql); if ($result->num_rows > 0) { $_SESSION["username"] = $username; header("Location: dashboard.php"); } else { echo "用户名或密码错误"; } } $conn->close(); ?>
-
Create a dashboard page (dashboard.php) to display the user's meeting schedule and other operations.
Code sample:<?php session_start(); include 'db_connect.php'; if (!isset($_SESSION["username"])) { header("Location: login.php"); exit; } $username = $_SESSION["username"]; $sql = "SELECT * FROM meetings WHERE username='$username'"; $result = $conn->query($sql); ?> <!DOCTYPE html> <html> <head> <title>在线会议系统</title> </head> <body> <h1 id="欢迎-php-echo-username">欢迎<?php echo $username; ?></h1> <h2 id="会议日程">会议日程</h2> <table> <tr> <th>会议标题</th> <th>开始时间</th> <th>结束时间</th> <th>地点</th> </tr> <?php while ($row = $result->fetch_assoc()) { echo "<tr>"; echo "<td>" . $row["title"] . "</td>"; echo "<td>" . $row["start_time"] . "</td>"; echo "<td>" . $row["end_time"] . "</td>"; echo "<td>" . $row["location"] . "</td>"; echo "</tr>"; } ?> </table> </body> </html>
The above is an example of a simple online conference system developed using PHP. Developers can continue to improve and expand functions on this basis, such as inviting participants, modifying meetings, etc. Hope this article is helpful to you.
The above is the detailed content of How to develop a simple online conference system using PHP. For more information, please follow other related articles on the PHP Chinese website!
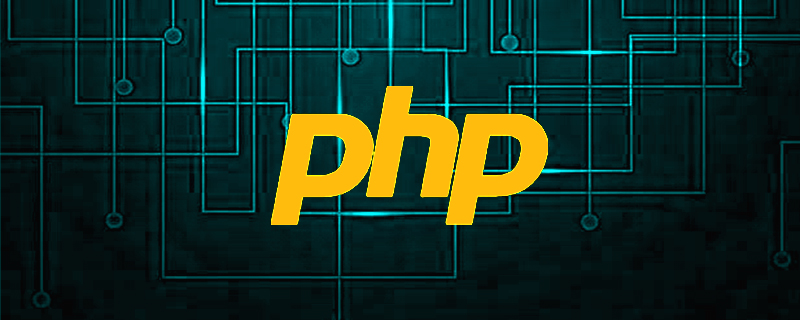
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
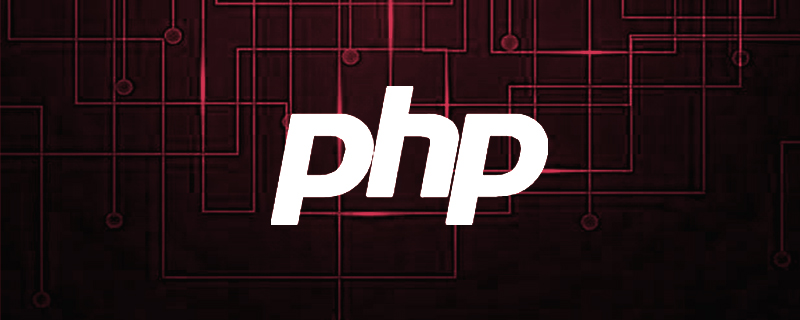
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
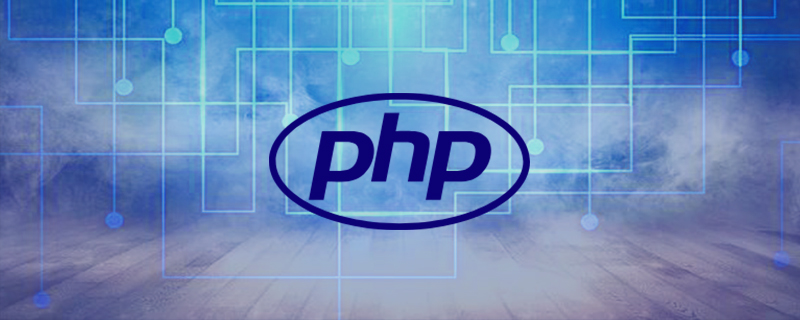
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
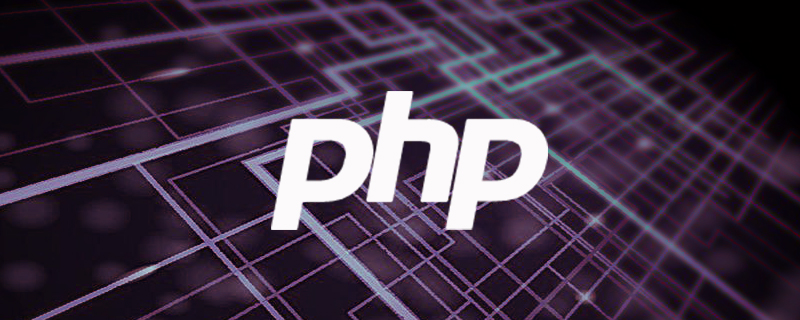
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
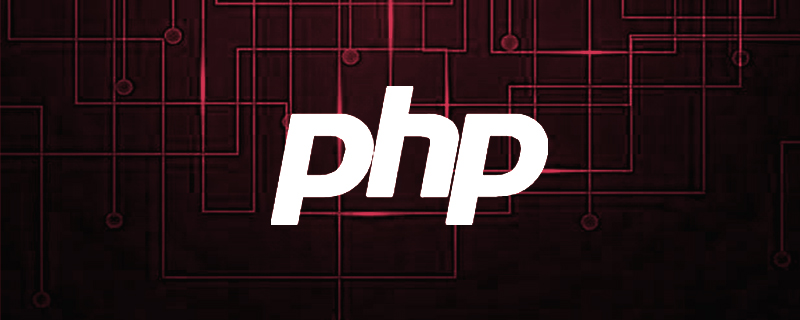
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
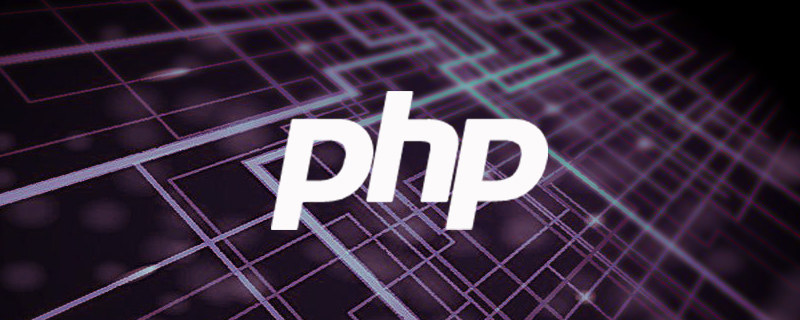
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
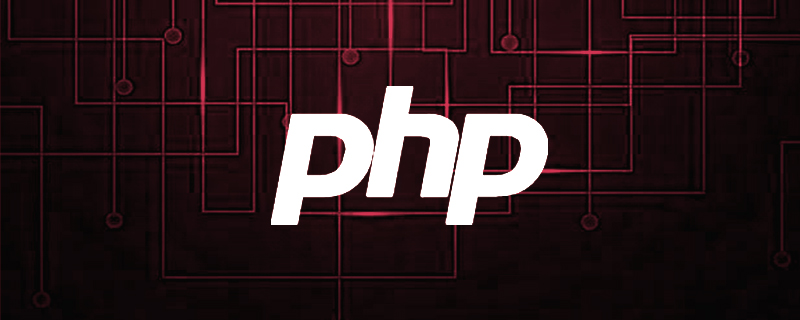
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
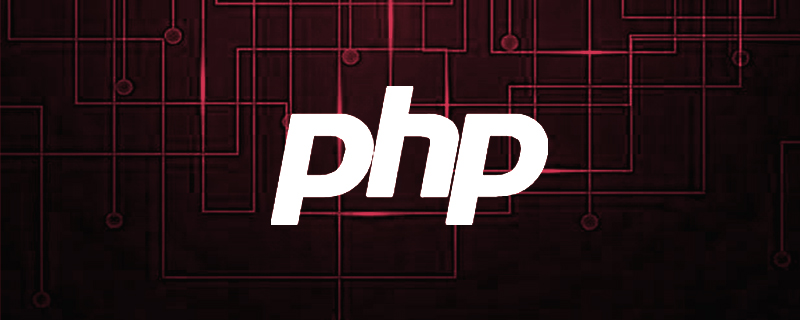
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
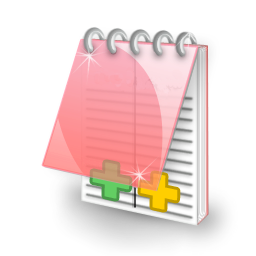
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
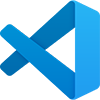
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
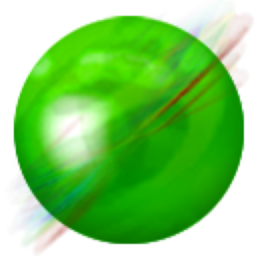
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
