How to write a linear search algorithm in Python?
Linear search is one of the simplest search algorithms, also known as sequential search. Its principle is very simple, that is, it traverses the data set to be searched from beginning to end, and compares the search target with the elements in the data set one by one.
Below we will introduce how to use Python to write a linear search algorithm and give specific code examples.
-
Algorithm implementation steps:
- Traverse the data set to be found and compare targets and elements one by one.
- If the target is found, return the index position of the element.
- If the target is not found after traversing all elements, -1 will be returned.
-
Code example:
def linear_search(arr, target): for i in range(len(arr)): if arr[i] == target: return i return -1 # 测试代码 arr = [1, 2, 3, 4, 5] target = 3 result = linear_search(arr, target) if result != -1: print("目标元素在索引位置:", result) else: print("未找到目标元素")
The above code implements a simple linear search algorithm. First define a linear_search
function, which accepts two parameters: one is the data set to be found arr
, and the other is the target element target
.
Next, iterate through each element in arr
through a for
loop and compare it with target
. If the target element is found, the index position of the element is returned. If the target element is not found after the traversal is completed, -1 is returned.
In the test code section, we define a sample data collection arr
and the target element target
, and then call the linear_search
function to search. Finally, the corresponding prompt information is output according to the return result of the function.
Please note that the time complexity of the linear search algorithm is O(n), where n is the size of the data set to be found. Linear search algorithms may be less efficient when the data collection is large because all elements need to be compared one by one.
Summary:
It is very simple to write a linear search algorithm in Python. You only need to traverse the data set to be found and compare it with the target element one by one. With the above code examples, we can easily understand and implement the linear search algorithm.
The above is the detailed content of How to write a linear search algorithm in Python?. For more information, please follow other related articles on the PHP Chinese website!
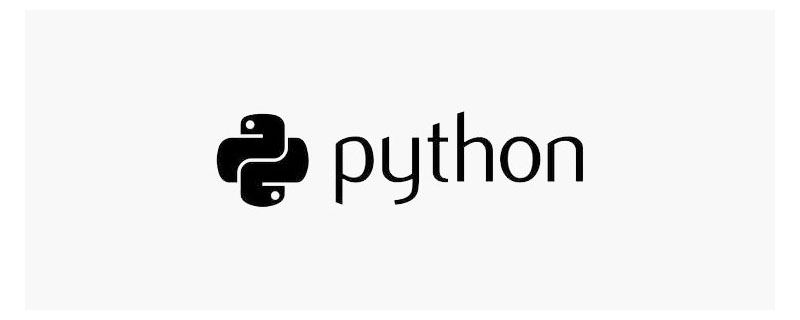
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
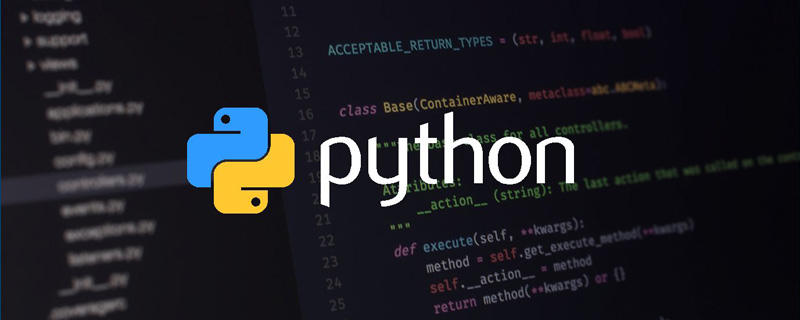
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
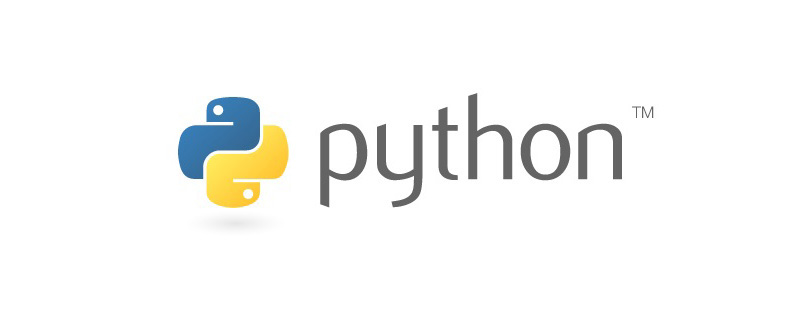
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
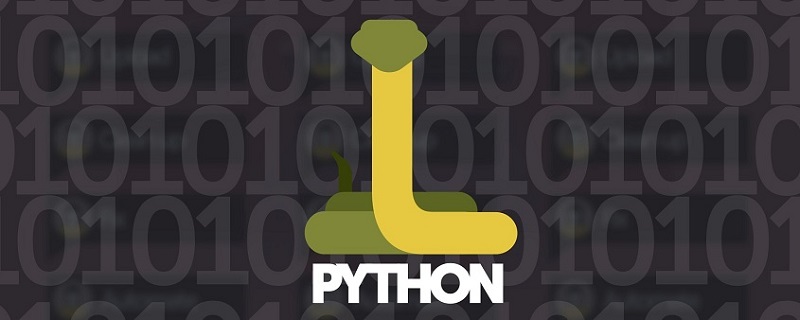
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
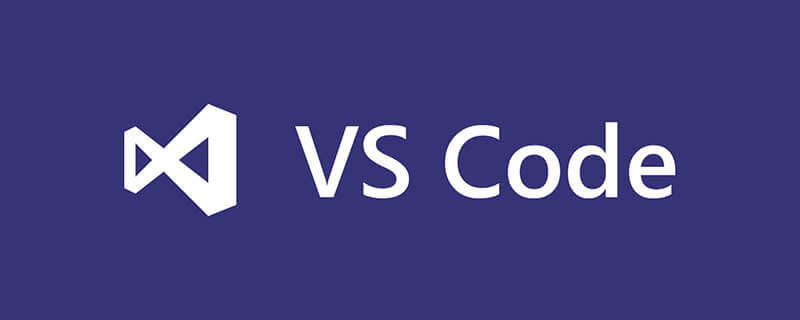
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
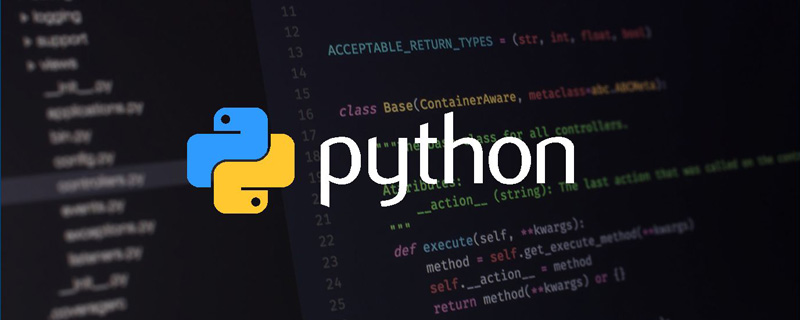
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
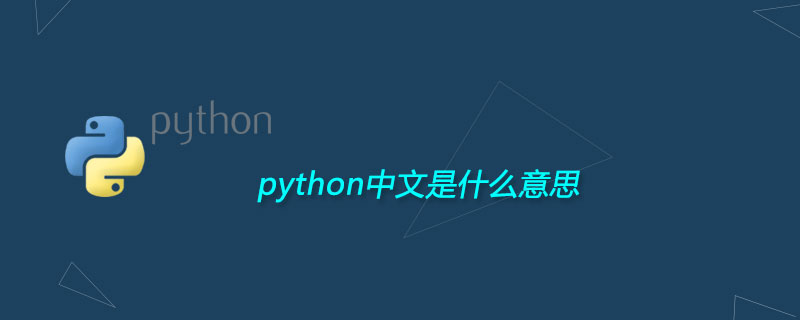
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
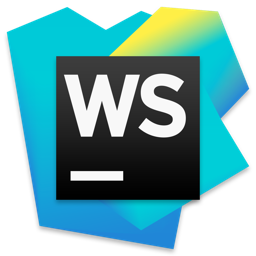
WebStorm Mac version
Useful JavaScript development tools
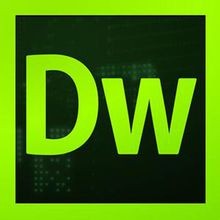
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
