How to use Vue to implement scrolling listening effects
How to use Vue to implement scrolling listening effects
Introduction:
Scrolling listening is one of the commonly used special effects in web development. It allows us to scroll the page when we scroll. , triggering corresponding animation, loading data or other interactive behaviors based on the scroll position. As a popular JavaScript framework, Vue provides a wealth of tools and functions that can help us implement scrolling monitoring effects. In this article, we will learn how to use Vue to implement scrolling listening effects and provide detailed code examples.
Step 1: Create Vue projects and components
First, we need to create a Vue project and create a component in it to implement scrolling listening effects. You can use Vue CLI to quickly build a Vue project. The command is as follows:
$ vue create scroll-listen-demo
After the creation is successful, enter the project directory and install the relevant dependencies:
$ cd scroll-listen-demo $ npm install
Then, create a project named ScrollListen
component file ScrollListen.vue
, and write the basic code in it:
<template> <div class="scroll-listen"> <!-- 在此处编写滚动监听特效的HTML代码 --> </div> </template> <script> export default { name: 'ScrollListen', data() { return { // 在此处定义状态等等 } }, mounted() { // 在此处编写滚动监听特效的代码 }, } </script> <style scoped> .scroll-listen { // 在此处编写滚动监听特效的样式 } </style>
Step 2: Use the vue-scrollama library to implement scrolling listening
In order to simplify scrolling monitoring For implementation, we can use the vue-scrollama
library. Execute the following command in the terminal to install:
$ npm install vue-scrollama
After the installation is completed, introduce the relevant code of vue-scrollama
into the ScrollListen.vue
component:
<template> <div class="scroll-listen"> <div v-for="(section, index) in sections" :key="index" class="section" > <h2 id="section-title">{{ section.title }}</h2> <p>{{ section.content }}</p> </div> </div> </template> <script> import { Scrollama, Step } from 'vue-scrollama'; export default { name: 'ScrollListen', components: { Scrollama, Step, }, data() { return { sections: [ { title: 'Section 1', content: 'Section 1 Content' }, { title: 'Section 2', content: 'Section 2 Content' }, { title: 'Section 3', content: 'Section 3 Content' }, ], }; }, mounted() { // 在此处编写滚动监听特效的代码 }, } </script> <style scoped> .scroll-listen { // 在此处编写滚动监听特效的样式 } .section { height: 100vh; } </style>
Next, we need to write the scroll monitoring code in the mounted
life cycle hook. First, introduce the Scrollama
component and initialize the Scrollama
instance in the mounted
method:
import { Scrollama, Step } from 'vue-scrollama'; export default { // ... mounted() { this.initScrollama(); }, methods: { initScrollama() { const scroller = new Scrollama(); scroller .onStepEnter(({ index }) => { // 在这里触发滚动进入某个步骤后的动作 }) .onStepExit(({ index }) => { // 在这里触发滚动离开某个步骤后的动作 }) .setup({ step: '.section', }); }, }, }
in the initScrollama
method , we created a Scrollama
instance and specified the callback functions when scrolling in and out through the onStepEnter
and onStepExit
methods. You can write corresponding logic in these two callback functions according to actual needs, such as displaying animations, loading data, etc.
Step 3: Use scrolling listening effects
The specific implementation steps of the scrolling listening effects have been completed. Now we can use the scrolling listening effects in the ScrollListen.vue
component. Add more sections in the sections
array and add the class name section
on each section
element:
<template> <div class="scroll-listen"> <div v-for="(section, index) in sections" :key="index" class="section" > <h2 id="section-title">{{ section.title }}</h2> <p>{{ section.content }}</p> </div> </div> </template> <script> // ... data() { return { sections: [ { title: 'Section 1', content: 'Section 1 Content' }, { title: 'Section 2', content: 'Section 2 Content' }, { title: 'Section 3', content: 'Section 3 Content' }, // 可以继续添加更多的section ], }; }, // ... </script>
Next, We can write the corresponding logic in the onStepEnter
and onStepExit
callback functions. For example, in the onStepEnter
callback function, we can modify the style of a section
based on the value of index
to achieve animation effects:
// ... methods: { // ... initScrollama() { const scroller = new Scrollama(); scroller .onStepEnter(({ index }) => { const activeSection = document.querySelectorAll('.section')[index]; activeSection.style.backgroundColor = 'red'; // 修改背景色为红色 }) .onStepExit(({ index }) => { const activeSection = document.querySelectorAll('.section')[index]; activeSection.style.backgroundColor = ''; // 恢复背景色 }) .setup({ step: '.section', }); }, }, // ... </script>
In this way, we can trigger corresponding animations, style changes or other interactive behaviors based on the scroll position.
Summary:
In this article, we learned how to use Vue to implement scrolling listening effects. By using the vue-scrollama
library, we can simplify the implementation process of scroll monitoring and implement scroll entry and scroll exit by writing onStepEnter
and onStepExit
callback functions. Actions. I hope this article will be helpful for you to learn Vue to implement scrolling listening effects. If you have any questions, please feel free to leave a message.
The above is the detailed content of How to use Vue to implement scrolling listening effects. For more information, please follow other related articles on the PHP Chinese website!

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
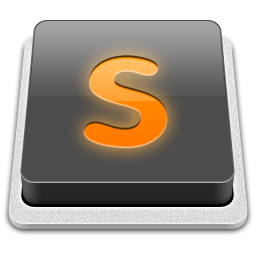
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
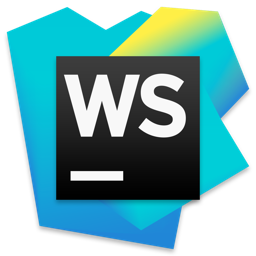
WebStorm Mac version
Useful JavaScript development tools
