How to use Vue to implement gesture sliding effects
How to use Vue to implement gesture sliding effects
Introduction: With the popularity of mobile devices, users have higher and higher requirements for interactive experience. As a common interaction method, gesture sliding effects have become a standard feature of many applications. This article is based on the Vue framework. Through specific code examples, we will introduce how to use Vue to achieve gesture sliding effects.
1. Introduction to Vue framework
Vue is a progressive framework for building user interfaces. Its core library only focuses on the view layer and is easy to integrate with other libraries or existing projects. Vue provides a complete set of tool libraries that enable us to quickly complete complex interactive effects through simple and easy-to-understand syntax.
2. Introducing the gesture sliding library
Before using Vue to implement gesture sliding effects, we need to introduce a gesture sliding library to facilitate our implementation of gesture operations. Here we choose to use the Hammer.js library. Hammer.js is a flexible and multi-functional touch gesture library that can support various gesture operations, including sliding, zooming, rotating, etc.
First, install the Hammer.js library in the Vue project:
npm install hammerjs
Then, in the entry file of the Vue component (usually main.js), introduce the Hammer.js library:
import Hammer from 'hammerjs' Vue.prototype.$hammer = Hammer
3. Realizing the special effects of gesture sliding
Next, we start to realize the special effects of gesture sliding. First, in the template of the Vue component, create a sliding container and bind a unique id:
<template> <div id="slider" ref="slider"> <!-- 滑动内容 --> </div> </template>
Then, in the script of the Vue component, add the following code:
export default { mounted() { const slider = this.$refs.slider const hammer = new this.$hammer.Manager(slider) const swipe = new this.$hammer.Swipe() hammer.add(swipe) hammer.on('swipeleft', () => { // 向左滑动操作 }) hammer.on('swiperight', () => { // 向右滑动操作 }) } }
Pass In the above code, we create a Hammer.js Manager object and bind it to the sliding container. Then, we created a Swipe object and added it to the Manager object through the add method. Finally, we can listen to the swipeleft and swiperight events through the on method and perform the corresponding operations in the event callback function.
So far, we have completed the implementation of gesture sliding effects.
4. Supplementary instructions
- You can add more gesture operations according to actual needs, such as swipeup and swipedown, etc.
- It should be noted that the Hammer.js library needs to be bound to specific DOM elements. In this example, we bind it to the slider container.
- You can perform some animation effects or page jumps in the event callback function to increase the user experience.
Conclusion: Through the introduction of this article, we have learned how to use the Vue framework combined with the Hammer.js library to achieve gesture sliding effects. I hope the content of this article will be helpful to everyone, and I also hope that everyone can flexibly use gesture sliding effects in actual projects to improve user experience.
The above is the detailed content of How to use Vue to implement gesture sliding effects. For more information, please follow other related articles on the PHP Chinese website!

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.

Vue.js is suitable for fast development and small projects, while React is more suitable for large and complex projects. 1.Vue.js is simple and easy to learn, suitable for rapid development and small projects. 2.React is powerful and suitable for large and complex projects. 3. The progressive features of Vue.js are suitable for gradually introducing functions. 4. React's componentized and virtual DOM performs well when dealing with complex UI and data-intensive applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
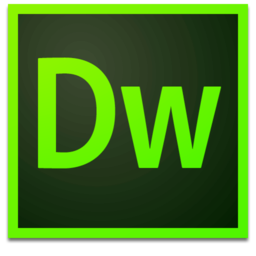
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
