How to use the backtracking algorithm in C++
How to use the backtracking algorithm in C
The backtracking algorithm is an algorithm that solves a problem by trying all possible solutions. It is often used to solve combinatorial problems, where the goal is to find all possible combinations that satisfy a set of constraints.
Take the permutation problem as an example. Suppose there is an array nums, and we need to find all possible permutations in it. The following will introduce how to use the backtracking algorithm to solve this problem through a C code example.
#include <iostream> #include <vector> using namespace std; void backtrack(vector<int>& nums, vector<vector<int>>& res, vector<bool>& visited, vector<int>& permutation) { // 如果当前排列的长度等于数组长度,说明已经找到一种解法 if (permutation.size() == nums.size()) { res.push_back(permutation); return; } for (int i = 0; i < nums.size(); i++) { // 如果当前数字已经被访问过,跳过继续下一次尝试 if (visited[i]) { continue; } // 将当前数字添加到排列中 permutation.push_back(nums[i]); visited[i] = true; // 继续尝试下一个数字 backtrack(nums, res, visited, permutation); // 回溯,从排列中移除当前数字,重新标记为未访问状态 permutation.pop_back(); visited[i] = false; } } vector<vector<int>> permute(vector<int>& nums) { vector<vector<int>> res; vector<bool> visited(nums.size(), false); vector<int> permutation; backtrack(nums, res, visited, permutation); return res; } int main() { vector<int> nums = {1, 2, 3}; vector<vector<int>> res = permute(nums); // 打印结果 for (vector<int> permutation : res) { for (int num : permutation) { cout << num << " "; } cout << endl; } return 0; }
Run the above code, the output result is:
1 2 3 1 3 2 2 1 3 2 3 1 3 1 2 3 2 1
In the above code, we use the backtracking algorithm to solve the arrangement problem. The backtracking function backtrack
tries all possible permutations through a depth-first search, while using the visited
array to mark the visited numbers to avoid duplication. When the length of the permutation is equal to the length of the array, the current permutation is added to the result.
Through this example, we can see that the core idea of the backtracking algorithm is to try all possible solutions and backtrack when the solution does not meet the conditions. In actual applications, some optimizations can be performed according to the requirements of specific problems, such as pruning operations to reduce unnecessary attempts. The backtracking algorithm has powerful solving capabilities in many combinatorial problems. For beginners, mastering the backtracking algorithm is very beneficial.
The above is the detailed content of How to use the backtracking algorithm in C++. For more information, please follow other related articles on the PHP Chinese website!
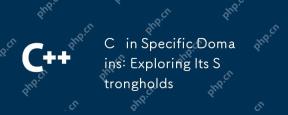
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
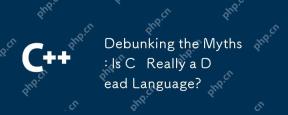
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
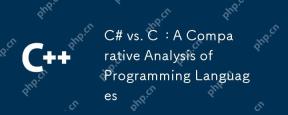
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
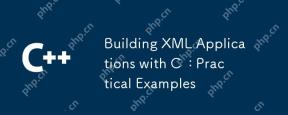
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
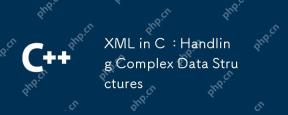
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
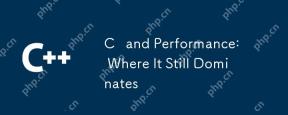
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
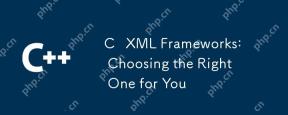
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
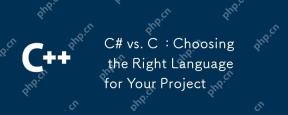
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
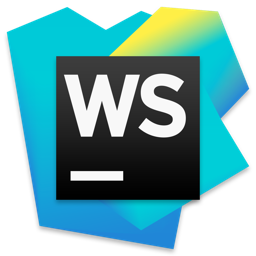
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use
