How to implement the merge sort algorithm in C#
How to implement the merge sort algorithm in C
#Merge sort is a classic sorting algorithm based on the divide and conquer idea, which divides a large problem into multiple small problems, then gradually solve the small problems and merge the results to complete the ranking. The following will introduce how to implement the merge sort algorithm in C# and provide specific code examples.
The basic idea of merge sort is to split the sequence to be sorted into multiple subsequences, sort them separately, and then merge the sorted subsequences into an ordered sequence. The key to this algorithm is to implement the splitting and merging operations of subsequences.
First, we need to write a recursive function to implement the split operation, divide the original sequence into two subsequences, and recursively call the merge sort algorithm to sort the subsequences. The specific code is as follows:
static void MergeSort(int[] array, int left, int right) { if (left < right) { int middle = (left + right) / 2; MergeSort(array, left, middle); MergeSort(array, middle + 1, right); Merge(array, left, middle, right); } }
Next, we need to write a merge function to merge two ordered subsequences into one ordered sequence. The key to the merge operation is to compare the elements in the two subsequences and put them into an auxiliary array in order of size. The specific code is as follows:
static void Merge(int[] array, int left, int middle, int right) { int[] temp = new int[array.Length]; int i = left; int j = middle + 1; int k = left; while (i <= middle && j <= right) { if (array[i] <= array[j]) { temp[k] = array[i]; i++; } else { temp[k] = array[j]; j++; } k++; } while (i <= middle) { temp[k] = array[i]; i++; k++; } while (j <= right) { temp[k] = array[j]; j++; k++; } for (int l = left; l <= right; l++) { array[l] = temp[l]; } }
Finally, we can sort the array to be sorted by calling the MergeSort function. The specific code is as follows:
static void Main(string[] args) { int[] array = { 5, 3, 8, 4, 2, 1, 9, 7, 6 }; MergeSort(array, 0, array.Length - 1); Console.WriteLine("排序后的数组:"); for (int i = 0; i < array.Length; i++) { Console.Write(array[i] + " "); } Console.ReadLine(); }
The above are the detailed steps to implement the merge sort algorithm in C# and code examples. By recursively splitting the sequence, sorting the subsequences, and merging the results, we can efficiently sort sequences of any size. The time complexity of merge sort is O(nlogn), which is a relatively fast sorting algorithm.
The above is the detailed content of How to implement the merge sort algorithm in C#. For more information, please follow other related articles on the PHP Chinese website!
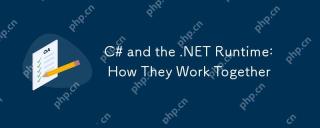
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
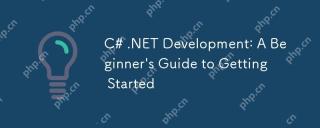
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
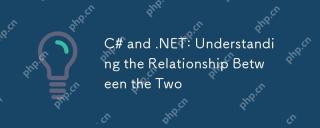
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
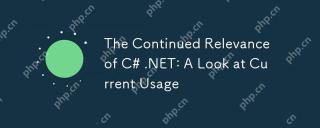
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
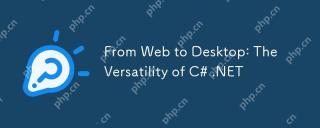
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
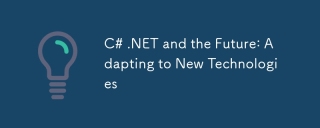
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
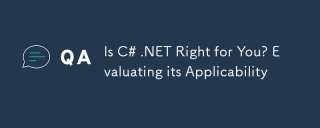
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
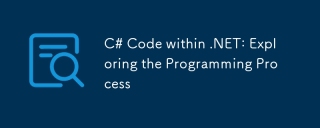
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
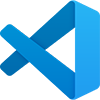
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
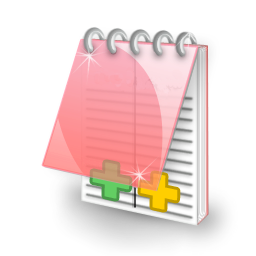
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use