How to get the build version number of an Android application?
Getting the build number of your Android application is easy. To do this, users should first launch the app on their Android device or emulator and then navigate to its settings or options menu. Next, they should look for the "About" or "Information" section, as this is where the build number usually lives.
Once found, click on it and it will display the necessary details, including a combination of numbers and/or letters, such as "1.2.3" or "v1.0.0". Since both developers and users require this information to identify different versions and updates, obtaining build version numbers is critical for effective management of Android applications.
Build version
The build number in Android is a unique identifier that distinguishes different versions of an application or operating system. This ID helps track and distinguish updates, ensuring seamless functionality in the Android platform.
Developers rely on build numbers to effectively manage and communicate critical software releases. This numbering system enables users to easily identify and monitor the exact application or Android OS version installed. Additionally, this approach can help developers pinpoint and resolve user-reported issues by quickly identifying which specific version is involved.
method
Let’s discuss ways to quickly find the build number in Android.
Use PackageManager
Use BuildConfig
Use package manager
The Android PackageManager class allows you to programmatically easily obtain the build version number of your application. Accessing package information is done using the getPackageManager() method, which allows you to get details not only of your application, but also of other installed applications. Another useful method involves calling getPackageInfo() to retrieve a PackageInfo object by specifying the application's package name.
The application object contains basic information such as version name and code. The version name represents a human-readable string, while the version code represents an integer used to compare versions programmatically. These values can be used for a variety of purposes, including displaying important information and implementing functionality specific to each version of the application.
algorithm
Get the package manager instance.
Package information can be retrieved using the getPackageInfo() method. Just pass in the name of the package and any necessary flags as arguments.
Retrieve version information from the PackageInfo object.
Use packageInfo.versionName to get the version name.
Use packageInfo.versionCode to get the version code.
Use version name and version code according to your needs.
Example
import android.content.pm.PackageInfo; import android.content.pm.PackageManager; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.widget.TextView; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Get the package manager instance PackageManager packageManager = getPackageManager(); try { // Get the package information PackageInfo packageInfo = packageManager.getPackageInfo(getPackageName(), 0); // Retrieve the version information String versionName = packageInfo.versionName; int versionCode = packageInfo.versionCode; // Use the version information TextView versionNameTextView = findViewById(R.id.versionNameTextView); versionNameTextView.setText("Version Name: " + versionName); TextView versionCodeTextView = findViewById(R.id.versionCodeTextView); versionCodeTextView.setText("Version Code: " + versionCode); } catch (PackageManager.NameNotFoundException e) { e.printStackTrace(); } } }
Output
Version Name: 1.2.3 Version Code: 123
Use build configuration
The BuildConfig class allows programmatic and efficient retrieval of a program's version information in an Android application. This class is automatically generated during the build process and contains several fields used to configure and support the build version of the program, such as version code and version name.
By appropriately accessing the various Build Configuration fields, it is possible to retrieve a human-readable string called a version name that is typically provided to the user and a unique integer value called a version code that is used for programmatic comparisons.
Developers can use these values in their applications for a variety of purposes, including displaying version information and implementing version-specific functionality. The BuildConfig method provides a direct and convenient way to access the build number in Android application code.
algorithm
Access BuildConfig class.
Retrieve the version name from BuildConfig.VERSION_NAME.
Retrieve the version code from BuildConfig.VERSION_CODE.
Use the version name and version code as needed in your application.
Example
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.widget.TextView; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Retrieve the version name from BuildConfig String versionName = BuildConfig.VERSION_NAME; // Retrieve the version code from BuildConfig int versionCode = BuildConfig.VERSION_CODE; // Use the version information TextView versionNameTextView = findViewById(R.id.versionNameTextView); versionNameTextView.setText("Version Name: " + versionName); TextView versionCodeTextView = findViewById(R.id.versionCodeTextView); versionCodeTextView.setText("Version Code: " + versionCode); } }
Output
Version Name: 1.2.3 Version Code: 123
in conclusion
In this case, there are two ways to retrieve the build number of the Android application. The first method involves using the PackageManager class to access package information and retrieve the version name and code, while the second method provides direct access to configuration fields for version-related data through the BuildConfig class. Both methods are efficient and programming-friendly ways to obtain important version information.
By merging these methods, developers can easily display build version numbers and implement version-specific functionality in their Android apps. Additionally, knowing the version of your application allows you to perform other tasks. Developers should choose the appropriate method based on their needs and use version names and version codes accordingly.
The above is the detailed content of How to get the build version number of an Android application?. For more information, please follow other related articles on the PHP Chinese website!
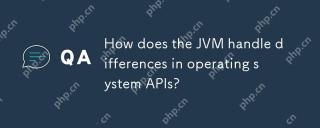
JVM handles operating system API differences through JavaNativeInterface (JNI) and Java standard library: 1. JNI allows Java code to call local code and directly interact with the operating system API. 2. The Java standard library provides a unified API, which is internally mapped to different operating system APIs to ensure that the code runs across platforms.
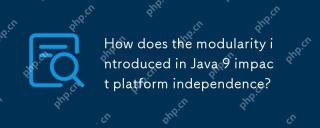
modularitydoesnotdirectlyaffectJava'splatformindependence.Java'splatformindependenceismaintainedbytheJVM,butmodularityinfluencesapplicationstructureandmanagement,indirectlyimpactingplatformindependence.1)Deploymentanddistributionbecomemoreefficientwi
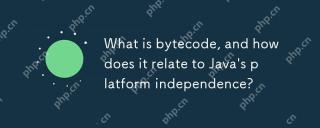
BytecodeinJavaistheintermediaterepresentationthatenablesplatformindependence.1)Javacodeiscompiledintobytecodestoredin.classfiles.2)TheJVMinterpretsorcompilesthisbytecodeintomachinecodeatruntime,allowingthesamebytecodetorunonanydevicewithaJVM,thusfulf
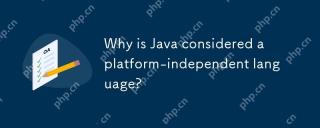
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),whichexecutesbytecodeonanydevicewithaJVM.1)Javacodeiscompiledintobytecode.2)TheJVMinterpretsandexecutesthisbytecodeintomachine-specificinstructions,allowingthesamecodetorunondifferentp
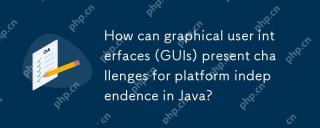
Platform independence in JavaGUI development faces challenges, but can be dealt with by using Swing, JavaFX, unifying appearance, performance optimization, third-party libraries and cross-platform testing. JavaGUI development relies on AWT and Swing, which aims to provide cross-platform consistency, but the actual effect varies from operating system to operating system. Solutions include: 1) using Swing and JavaFX as GUI toolkits; 2) Unify the appearance through UIManager.setLookAndFeel(); 3) Optimize performance to suit different platforms; 4) using third-party libraries such as ApachePivot or SWT; 5) conduct cross-platform testing to ensure consistency.
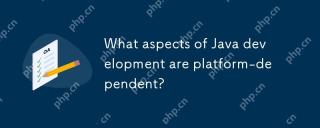
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
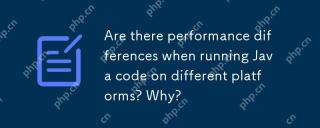
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
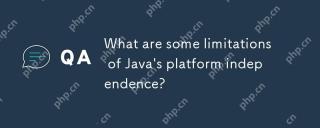
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
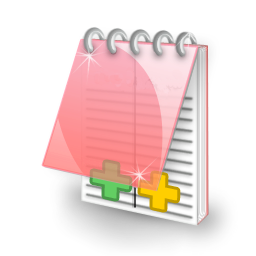
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
