High-performance database search practice sharing driven by Java technology
Sharing of high-performance database search practices driven by Java technology
Abstract: As the amount of data continues to grow, high-performance database search is becoming more and more important. This article will introduce how to use Java technology to implement high-performance database search, and provide specific code examples to help readers better understand and apply.
Introduction: In the era of modern information technology, the importance of data is self-evident. Massive amounts of data are stored in databases, and efficient search in the database is a core technology that developers are concerned about. This article will introduce how to use Java technology to implement high-performance database search, and conduct specific operations through examples.
1. Use Java to connect to the database
In Java, you can use JDBC (Java Database Connectivity) technology to connect and operate the database. The following is a simple code example for using JDBC to connect to the database:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.SQLException; public class DatabaseConnection { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydb"; // 数据库地址 String username = "root"; // 用户名 String password = "password"; // 密码 Connection connection = null; try { connection = DriverManager.getConnection(url, username, password); System.out.println("Connect to database successfully!"); } catch (SQLException e) { System.out.println("Connect to database failed!"); e.printStackTrace(); } finally { if (connection != null) { try { connection.close(); } catch (SQLException e) { e.printStackTrace(); } } } } }
2. Using Java technology to implement database search
The core of database search is to write efficient SQL query statements and use indexes to improve search performance . The following is a simple code example using Java for database search:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class DatabaseSearch { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/mydb"; String username = "root"; String password = "password"; String searchKeyword = "Java"; // 搜索关键词 Connection connection = null; try { connection = DriverManager.getConnection(url, username, password); String sql = "SELECT * FROM books WHERE title LIKE ?"; PreparedStatement statement = connection.prepareStatement(sql); statement.setString(1, "%" + searchKeyword + "%"); ResultSet resultSet = statement.executeQuery(); while (resultSet.next()) { String bookTitle = resultSet.getString("title"); String bookAuthor = resultSet.getString("author"); System.out.println("Book Title: " + bookTitle); System.out.println("Book Author: " + bookAuthor); } } catch (SQLException e) { e.printStackTrace(); } finally { if (connection != null) { try { connection.close(); } catch (SQLException e) { e.printStackTrace(); } } } } }
The above code implements a simple book search function. By entering a keyword, the program will find books containing the keyword from the database and for output.
Conclusion: This article introduces how to use Java technology to implement high-performance database search, and provides specific code examples through which readers can better understand and apply this technology. In actual projects, database search is one of the common functions. Therefore, improving the performance of database search is very important for the overall efficiency and user experience of the system. I hope this article can be helpful to readers and provide some ideas and inspiration. Thanks for reading!
The above is the detailed content of High-performance database search practice sharing driven by Java technology. For more information, please follow other related articles on the PHP Chinese website!
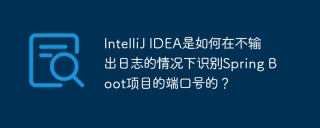
Start Spring using IntelliJIDEAUltimate version...
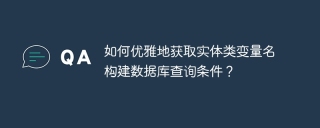
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
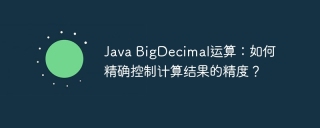
Java...
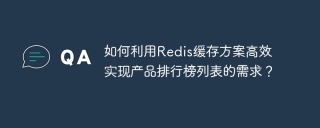
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
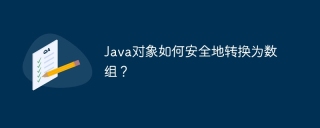
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
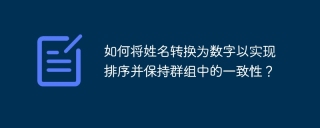
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
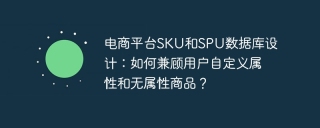
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
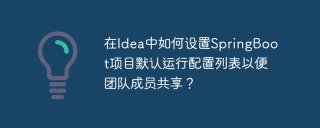
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
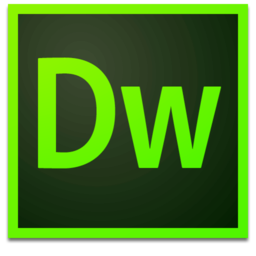
Dreamweaver Mac version
Visual web development tools
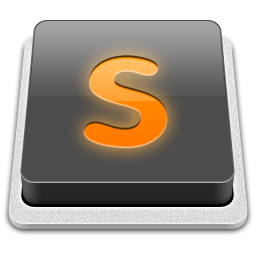
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
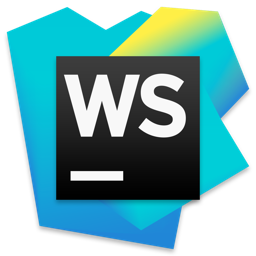
WebStorm Mac version
Useful JavaScript development tools