In Python, we can use a third-party Python library called openpyxl to replace one word in Excel with another word. Microsoft Excel is a useful tool for managing and analyzing data. Using Python, we can automate some Excel data management tasks. In this article, we will learn how to replace a word in Excel using Python.
Install openpyxl
Before replacing Word in Excel, we need to use the Python package manager to install the openpyxl library in the system. To install openpyxl, enter the following command in the terminal or command prompt.
Pip install openpyxl
grammar
openpyxl.load_workbook(‘your_excel_file’)
Here, the openpyxl.load_workbook() function loads the Excel file from the system. Once the file is loaded, you can perform operations on the worksheet.
Load Excel Spreadsheet
To load an Excel table, we first need to import openpyxl, then use the load_workbook() function to load the spreadsheet, and use the workbook.active property to select the active table.
Example
The code to load the workbook is as follows:
import openpyxl # Load the Excel spreadsheet workbook = openpyxl.load_workbook('example.xlsx') # Select the active worksheet worksheet = workbook.active Print("Workbook loaded")
Output
Workbook loaded
Replace a word
To replace a specific word in Excel, we need to iterate through each cell of the active Excel workbook, check if the word in the cell matches the word we want to replace, and then insert the new word in that cell.
Example
The code to replace old words with new words is shown below.
import openpyxl # Load the Excel spreadsheet workbook = openpyxl.load_workbook('testing.xlsx') # Select the active worksheet worksheet = workbook.active # Replace the word 'old_word' with 'new_word' for row in worksheet.iter_rows(): for cell in row: if cell.value == 'old_word': print("Word found") cell.value = 'new_word' print("word replaced") else: # Save the changes workbook.save('testing.xlsx')
Output
Word found word replaced
Replace multiple words
If we want to replace multiple words in an Excel spreadsheet, we can modify the previous code to use a dictionary instead of a single word. The keys in the dictionary represent the words to be replaced, and the values represent the words to be replaced.
Example
The following code demonstrates how to replace multiple words in an Excel spreadsheet -
import openpyxl # Load the Excel spreadsheet workbook = openpyxl.load_workbook('example.xlsx') # Select the active worksheet worksheet = workbook.active # Define the words to be replaced and their replacements replacements = { 'old_word_1': 'new_word_1', 'old_word_2': 'new_word_2', 'old_word_3': 'new_word_3' } # Replace the words for row in worksheet.iter_rows(): for cell in row: if cell.value in replacements: print("Word found") cell.value = replacements[cell.value] print("word replaced") else: print("word not found") # Save the changes workbook.save('example.xlsx')
Output
Word found word replaced
in conclusion
In this article, we discussed how to replace words in Excel using Python’s openpyxl library. openpyxl provides functionality to open a spreadsheet workbook and iterate over the cells of the workbook. We can also replace multiple words in the spreadsheet, as shown in one of the examples in this article.
The above is the detailed content of How to replace a word in Excel using Python?. For more information, please follow other related articles on the PHP Chinese website!
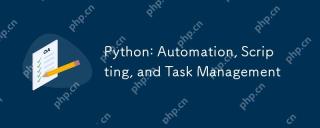
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
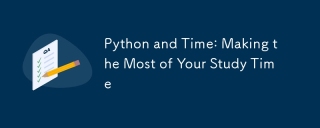
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
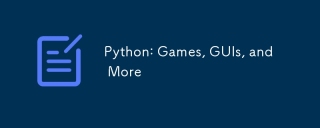
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
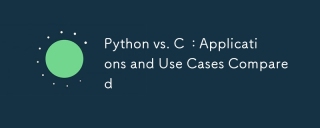
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
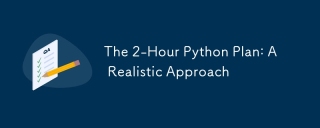
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
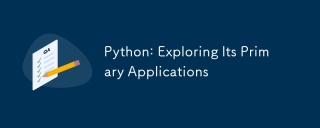
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
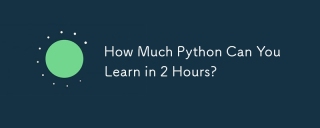
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
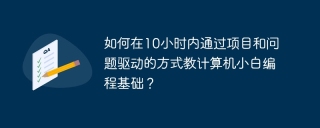
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
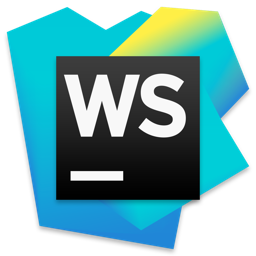
WebStorm Mac version
Useful JavaScript development tools
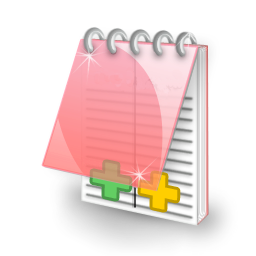
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
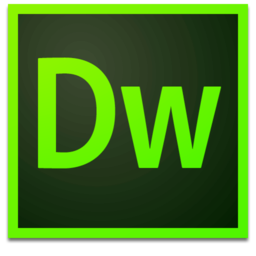
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.