Java program to alternately merge two or more files into a third file
Suppose we have three files -
output1.txt
Hello how are you
output2.txt
Welcome to Tutorialspoint
output3.txt
We provide simply easy learning
Example
The following Java example alternately merges the contents of the above three files into one file-
import java.util.Scanner; public class MergingFiles { public static void main(String args[]) throws IOException { Scanner sc1 = new Scanner(new File("D://input1.txt")); Scanner sc2 = new Scanner(new File("D://input2.txt")); Scanner sc3 = new Scanner(new File("D://input3.txt")); FileWriter writer = new FileWriter("D://result.txt"); String str[] = new String[3]; while (sc1.hasNextLine()||sc2.hasNextLine()||sc3.hasNextLine()) { str[0] = sc1.nextLine(); str[1] = sc2.nextLine(); str[2] = sc3.nextLine(); } writer.append(str[0]+"\n"); writer.append(str[1]+"\n"); writer.append(str[2]+"\n"); writer.flush(); System.out.println("Contents added "); } }
Output
Contents added
If the above three files are directly in the same file, you can rewrite the example program as -
Example
import java.io.File; import java.io.FileWriter; import java.io.IOException; import java.util.Scanner; public class MergingFiles { public static void main(String args[]) throws IOException { //Creating a File object for directory File directoryPath = new File("D:\example"); //List of all files and directories File filesList[] = directoryPath.listFiles(); Scanner sc = null; FileWriter writer = new FileWriter("D://output.txt"); for(File file : filesList) { sc = new Scanner(file); String input; StringBuffer sb = new StringBuffer(); while (sc.hasNextLine()) { input = sc.nextLine(); writer.append(input+"\n"); } writer.flush(); } System.out.println("Contents added "); } }
Output
Contents added
The above is the detailed content of Java program to alternately merge two or more files into a third file. For more information, please follow other related articles on the PHP Chinese website!
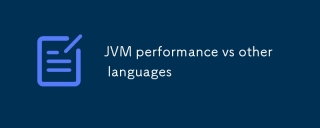
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
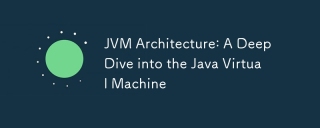
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
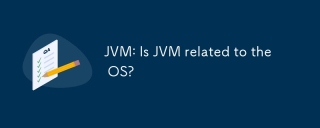
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
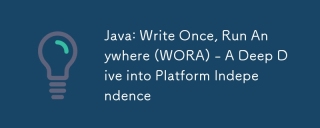
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
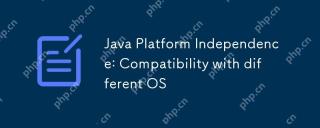
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
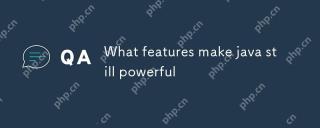
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
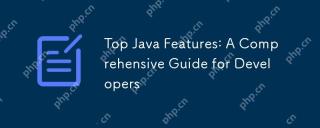
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
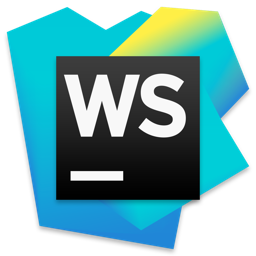
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
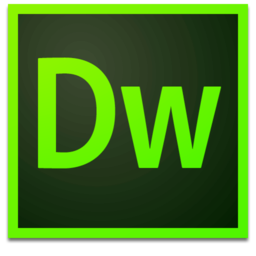
Dreamweaver Mac version
Visual web development tools
