Step by step: Vue3+Django4 full stack project implementation steps
Step by step: Vue3 Django4 full-stack project implementation steps
With the continuous development of web development, full-stack development has gradually become a trend. Vue and Django are one of the most popular technology stacks in front-end and back-end development, and their combination is also very powerful. In this article, we will introduce step by step how to implement a full stack project using Vue3 and Django4.
- Preparation
First, we need to install Node.js and Python locally, as well as the corresponding package managers npm and pip. At the same time, we also need an integrated development environment (IDE), such as VS Code. -
Create Django project
Open the terminal, enter the directory where you want to create the project, and execute the following command to create the Django project:django-admin startproject myproject
-
Create Django application
Enter the project directory and execute the following command to create a Django application:cd myproject python3 manage.py startapp myapp
-
Configure Django database
Open the settings.py file and configure the database information, such as using SQLite:DATABASES = { 'default': { 'ENGINE': 'django.db.backends.sqlite3', 'NAME': BASE_DIR / 'db.sqlite3', } }
-
Create database model
Define your database model in the models.py file. For example, we create a User model:from django.db import models class User(models.Model): name = models.CharField(max_length=100) email = models.EmailField() password = models.CharField(max_length=100)
-
Perform database migration
Run the following command to perform database migration:python3 manage.py makemigrations python3 manage.py migrate
-
Create Django Views
Write your Django view functions in the views.py file. For example, we create a view function to get all users:from django.shortcuts import render from django.http import JsonResponse from .models import User def get_users(request): users = User.objects.all() data = [{'name': user.name, 'email': user.email} for user in users] return JsonResponse({'users': data})
-
Create Vue project
In the terminal, enter the directory where you want to create a Vue project, and execute the following command to create Vue Project:vue create myproject
-
Configure Vue proxy
Configure the Vue proxy in the myproject/vue.config.js file and proxy the request to the Django backend:module.exports = { devServer: { proxy: { '^/api': { target: 'http://localhost:8000', changeOrigin: true } } } }
-
Create Vue component
Create a Users.vue component in the src/views directory to display the user list:<template> <div> <ul> <li v-for="user in users" :key="user.name"> {{ user.name }} - {{ user.email }} </li> </ul> </div> </template> <script> export default { data() { return { users: [] } }, created() { this.getUsers() }, methods: { getUsers() { axios.get('/api/users') .then(response => { this.users = response.data.users }) } } } </script>
-
Configure Vue routing
Configure Vue's routing in the src/router/index.js file and use the Users.vue component as a route:import Vue from 'vue' import VueRouter from 'vue-router' import Users from '../views/Users.vue' Vue.use(VueRouter) const routes = [ { path: '/', name: 'Users', component: Users } ] const router = new VueRouter({ mode: 'history', base: process.env.BASE_URL, routes }) export default router
-
Run the project
At the root of Django and Vue respectively Execute the following command in the directory to run the project:python3 manage.py runserver npm run serve
Now, you can visit http://localhost:8080 in the browser to view your full-stack project. The Users component will get the data from the Django backend and display it on the page.
Summary:
Through the above steps, we successfully implemented a full-stack project using Vue3 and Django4. By integrating front-end and back-end development, we can develop powerful web applications more efficiently. I hope this article will be helpful to you and make you better familiar with the full-stack development process of Vue and Django.
The above is the detailed content of Step by step: Vue3+Django4 full stack project implementation steps. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is loved by developers because it is easy to use and powerful. 1) Its responsive data binding system automatically updates the view. 2) The component system improves the reusability and maintainability of the code. 3) Computing properties and listeners enhance the readability and performance of the code. 4) Using VueDevtools and checking for console errors are common debugging techniques. 5) Performance optimization includes the use of key attributes, computed attributes and keep-alive components. 6) Best practices include clear component naming, the use of single-file components and the rational use of life cycle hooks.

Vue.js is a progressive JavaScript framework suitable for building efficient and maintainable front-end applications. Its key features include: 1. Responsive data binding, 2. Component development, 3. Virtual DOM. Through these features, Vue.js simplifies the development process, improves application performance and maintainability, making it very popular in modern web development.

Vue.js and React each have their own advantages and disadvantages, and the choice depends on project requirements and team conditions. 1) Vue.js is suitable for small projects and beginners because of its simplicity and easy to use; 2) React is suitable for large projects and complex UIs because of its rich ecosystem and component design.

Vue.js improves user experience through multiple functions: 1. Responsive system realizes real-time data feedback; 2. Component development improves code reusability; 3. VueRouter provides smooth navigation; 4. Dynamic data binding and transition animation enhance interaction effect; 5. Error processing mechanism ensures user feedback; 6. Performance optimization and best practices improve application performance.

Vue.js' role in web development is to act as a progressive JavaScript framework that simplifies the development process and improves efficiency. 1) It enables developers to focus on business logic through responsive data binding and component development. 2) The working principle of Vue.js relies on responsive systems and virtual DOM to optimize performance. 3) In actual projects, it is common practice to use Vuex to manage global state and optimize data responsiveness.

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
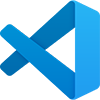
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor