In Python, a tuple is an immutable sequence type typically used to store a collection of items.
Python tuples are very similar to Python lists in terms of nesting, indexing, and repetition, but one difference is that tuples are immutable, while lists are mutable, which means we can change the elements of the list, but not Do the same with tuples. Another difference is that lists are declared using square brackets [] while tuples are declared using simple brackets ().
In this article, we will discuss how to create tuples in Python using tuple literals. We'll introduce the syntax for defining tuples and provide examples of creating tuples using elements of different types.
We can declare a tuple in the following way -
tup1 = ("this" , "is" , “a” , "tuple") tup2 = (1, 2, 3, 4, 5 ) tup3 = ("a", "b", "c", "d")
There are many ways to create tuples, but in this article, we will see how to create tuples using tuple literals.
Use tuple literals
Before using tuple literals to create tuple literals, let us first understand the meaning of literals in Python. The data we assign to variables and constants when we write code is called literals.
The values of these literals will not change during the entire execution of the program. There are many types of literal in Python, but if we have to refer to a collection type object, such as a list, tuple or dictionary, then we have to use a so-called "Literal Collection".
A tuple literal is an example of a collection of literals that can be used to create a tuple object in Python.
Example
In the following example, we will create a tuple using parentheses and comma declarations.
tuple1 = ( 1 , 2 , 3 , 4 , 5 , 6 , 7 ) print("the tuple is", tuple1 )
Output
The output of the above code is as follows -
the tuple is ( 1 , 2 , 3 , 4 , 5 , 6 , 7 )
Use another list
We will see how to create a tuple using another list. This method is also called type conversion. Essentially, what we do is create an object of type list and convert it to an object of tuple type. Using this method is an unconventional way to create a list, but is mentioned in this article as an alternative in case you need it.
Example
In the example below, we will create a list from another list. The tuple created will have the same elements as the list, but cannot be changed. We can do this by using the tuple() method, which is a built-in method provided by python to convert the data type in it to a tuple.
List1 = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 ] tuple1 = tuple(List1) print("the tuple is", tuple1 )
Output
The output of the above program is as follows -
the tuple is ( 1 , 2 , 3 , 4 , 5 , 6 , 7 )
Create tuple from string
We will see how to create tuples using form strings . With this approach, all characters of the string will be distinct elements of the created tuple. We can do this by using the tuple() function, which will convert the data type in it to a tuple.
Example
In the example below, we will create a list from strings . With this method, all characters in String will become elements of the tuple in the order in which they appear in String.
string1 = "This is a tuple" tuple1 = tuple(string1) print("the tuple is" , tuple1 )
Output
The output of the above program is as follows -
the tuple is ( ‘T’ , ‘h’ , ‘i’ , ‘s’ , ‘ ’ , ‘i’ , ‘s’ , ‘ ’ , ‘a’ , ‘ ’ , ‘t’ , ‘u’ , ‘p’ , ‘l’ , ‘e’ )
in conclusion
In this article, we discussed how tuples are created and their properties. We saw 3 different ways of creating tuples. In the first method, we create a tuple using parentheses with commas separating the elements. In the second method, we create a tuple from the list, in this method all the elements of the tuple are the same as the elements of the list from which it was created. In the third method, we create tuples from strings. All characters of a string are elements of a tuple.
The above is the detailed content of Create a Python program using tuple literals. For more information, please follow other related articles on the PHP Chinese website!
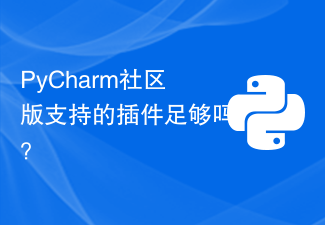
PyCharm社区版支持的插件足够吗?需要具体代码示例随着Python语言在软件开发领域的应用越来越广泛,PyCharm作为一款专业的Python集成开发环境(IDE),备受开发者青睐。PyCharm分为专业版和社区版两个版本,其中社区版是免费提供的,但其插件支持相对专业版有所限制。那么问题来了,PyCharm社区版支持的插件足够吗?本文将通过具体的代码示例
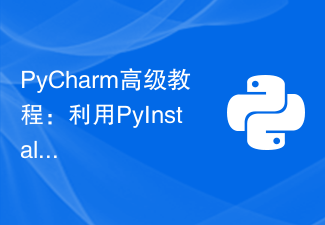
PyCharm是一款功能强大的Python集成开发环境,提供了丰富的功能和工具来帮助开发者提高效率。其中,PyInstaller是一个常用的工具,可以将Python代码打包为可执行文件(EXE格式),方便在没有Python环境的机器上运行。在本篇文章中,我们将介绍如何在PyCharm中使用PyInstaller将Python代码打包为EXE格式,并提供具体的
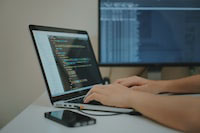
python元编程是一种强大的技术,它允许你对Python语言本身进行操作,赋予你编程超能力。元编程可以通过使用元类和装饰器来实现。元类是一种特殊的类,它负责创建其他类。装饰器是一种函数,它可以修改另一个函数的行为。元编程的一个常见用途是创建自定义的类。例如,你可以创建一个元类,它可以生成具有特定属性和方法的类。元编程还可以用于修改类的方法行为。例如,你可以创建一个装饰器,它可以对函数的输入和输出进行验证。元编程是一项强大的技术,它可以让你做很多有趣和有用的事情。如果你想成为一名更强大的Pyth
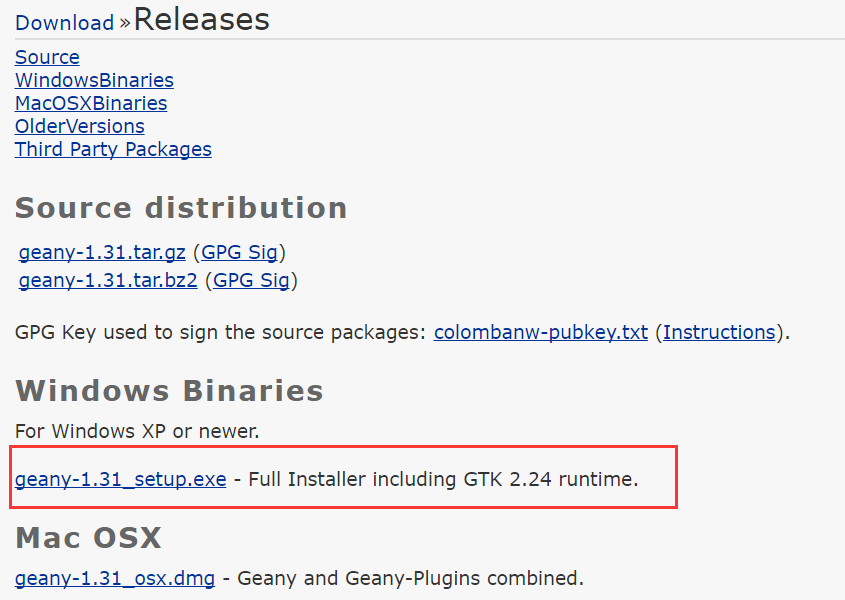
我们来了解一下吧:Geany是一个小巧的使用GTK+2开发的跨平台的开源集成开发环境,以GPL许可证分发源代码,是免费的自由软件。当前版本:1.31。该软件小巧、启动迅速,界面简洁,功能简单。它支持基本的语法高亮、代码自动完成、调用提示、插件扩展。支持文件类型:C,CPP,Java,Python,PHP,HTML,DocBook,Perl,LateX和Bash脚本。对于写多种语言的程序员来说,拥有Geany可以说是非常的方便了。知道了这么多,是不是迫不及待想要去尝试一下呢?下面让我们开始进行下载
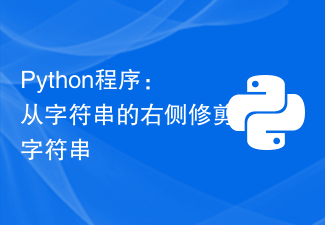
在Python中,我们有一个预定义的函数rstrip()来删除右侧的字符。这意味着它将删除字符串右侧的空格。让我们举一个例子来理解如何从字符串的左侧修剪。在给定的字符串“WIRELESS”中移除右侧字符串LESS并将结果值得到为“WIRE”。在给定的字符串“kingdom”中,删除右侧的字符串dom,得到结果值为“king”。语法以下示例中使用的语法为−isspace()这是Python中预定义的方法,用于允许字符中的空白、换行符或空格。rstrip("parameterasastri
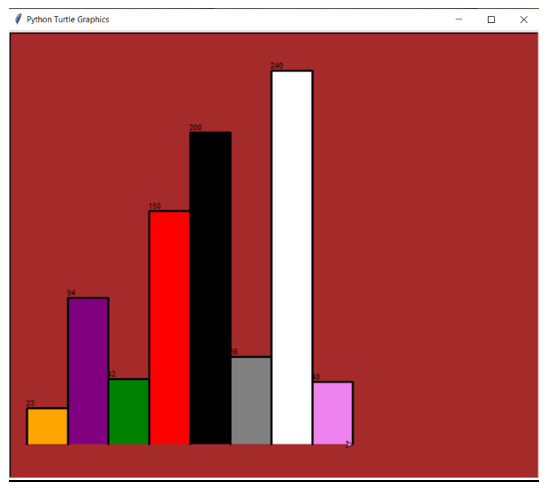
数据的图形表示提供了对数据复杂子结构的增强理解,帮助我们轻松解释隐藏的模式和趋势。想象一下,如果我们可以通过编程绘制类似的关系,那将是多么方便?Python提供了一个丰富的模块,专门用于执行此类操作,它被称为“turtle”。“turtle”模块是Python内置的库,允许我们在“turtle图形屏幕”上绘制图形。在本文中,我们将使用这个turtle模块创建一个条形图。理解Turtle模块Theturtlemoduleusesavirtualturtleobjecttocreategraphic
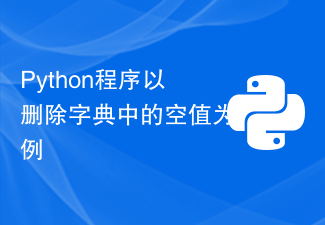
字典被称为集合数据类型。它们以键值对的形式存储数据。它们是有序的且可变的,即它们遵循特定的顺序并被索引。我们可以更改键的值,因此它是可操纵的或可更改的。字典不支持数据重复。每个键可以有多个与其关联的值,但单个值不能有多个键。我们可以使用字典来执行许多操作。整个机制取决于存储的值。在本文中,我们将讨论可用于从字典中删除“空值”的技术。在开始主要操作之前,我们必须对字典中的值处理有一个深入的了解。让我们快速浏览一下本文的概述。本文分为两部分-第1st部分将重点介绍“空值”的概念及其意义。在第2nd部
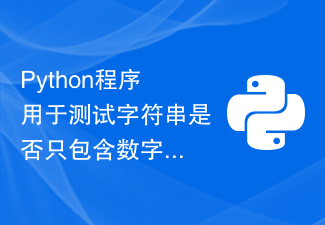
在使用Python处理字符串时,经常需要验证一个字符串是否只包含数字和字母,或者是否包含其他特殊字符。字符串验证在各种场景中都非常重要,比如输入验证、数据处理和过滤。在本文中,我们将探讨一个Python程序,用于测试给定的字符串是否仅包含字母数字字符。我们将讨论有效字符串的标准,提供有效和无效字符串的示例,并介绍使用内置字符串方法解决此问题的高效方法。理解问题在我们开始解决问题之前,让我们先定义一个只包含数字和字母的有效字符串的标准-字符串不应包含任何空格或特殊字符。字符串应由字母数字字符(a-


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
