OpenCV Hough circle transformation implemented in Java
You can use Hough circle transform to detect circles in a given image. You can apply the Hough Circle Transform using the HoughCircles() method, which accepts the following parameters -
- A Mat object representing the input image.
Mat object used to store the output vector of the found circle.
Integer variable representing the detection method.
Two double variables representing the inverse ratio of the accumulator resolution to the image resolution and the minimum distance between the detected circle centers.
Example
import org.opencv.core.Core; import org.opencv.core.Mat; import org.opencv.core.Point; import org.opencv.core.Scalar; import org.opencv.highgui.HighGui; import org.opencv.imgcodecs.Imgcodecs; import org.opencv.imgproc.Imgproc; import java.awt.Image; import java.awt.image.BufferedImage; import java.io.IOException; import javafx.application.Application; import javafx.embed.swing.SwingFXUtils; import javafx.scene.Group; import javafx.scene.Scene; import javafx.scene.image.ImageView; import javafx.scene.image.WritableImage; import javafx.stage.Stage; public class HoughCircleTransform extends Application { public void start(Stage stage) throws IOException { //Loading the OpenCV core library System.loadLibrary( Core.NATIVE_LIBRARY_NAME ); String file ="D:\Images\compass.jpg"; Mat src = Imgcodecs.imread(file); //Converting the image to Gray Mat gray = new Mat(); Imgproc.cvtColor(src, gray, Imgproc.COLOR_RGBA2GRAY); //Blurring the image Mat blur = new Mat(); Imgproc.medianBlur(gray, blur, 5); //Detecting the Hough Circles Mat circles = new Mat(); Imgproc.HoughCircles(blur, circles, Imgproc.HOUGH_GRADIENT, Math.PI/180, 150); for (int i = 0; i < circles.cols(); i++ ) { double[] data = circles.get(0, i); Point center = new Point(Math.round(data[0]), Math.round(data[1])); // circle center Imgproc.circle(src, center, 1, new Scalar(0, 0, 255), 3, 8, 0 ); // circle outline int radius = (int) Math.round(data[2]); Imgproc.circle(src, center, radius, new Scalar(0,0,255), 3, 8, 0 ); } //Converting matrix to JavaFX writable image Image img = HighGui.toBufferedImage(src); WritableImage writableImage= SwingFXUtils.toFXImage((BufferedImage) img, null); //Setting the image view ImageView imageView = new ImageView(writableImage); imageView.setX(10); imageView.setY(10); imageView.setFitWidth(575); imageView.setPreserveRatio(true); //Setting the Scene object Group root = new Group(imageView); Scene scene = new Scene(root, 595, 400); stage.setTitle("Hough Circle Transform"); stage.setScene(scene); stage.show(); } public static void main(String args[]) { launch(args); } }
Input picture
Output
When executed, the above will produce the following output-
The above is the detailed content of OpenCV Hough circle transformation implemented in Java. For more information, please follow other related articles on the PHP Chinese website!
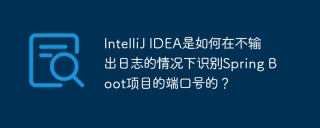
Start Spring using IntelliJIDEAUltimate version...
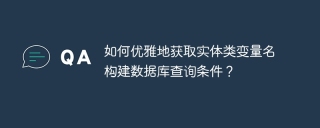
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
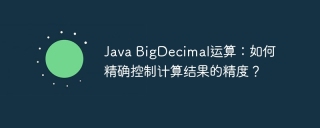
Java...
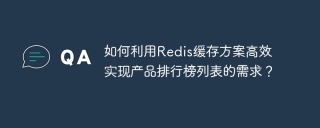
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
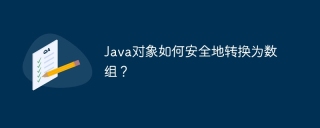
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
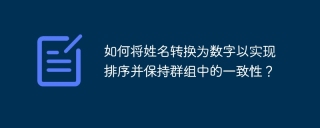
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
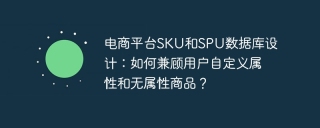
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
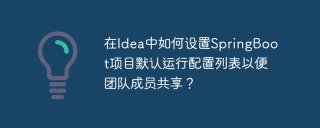
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
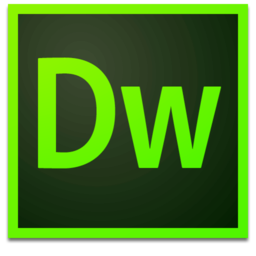
Dreamweaver Mac version
Visual web development tools
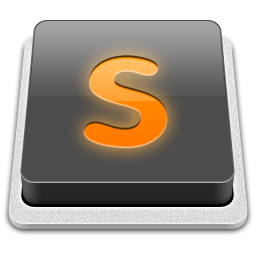
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
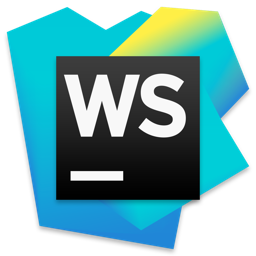
WebStorm Mac version
Useful JavaScript development tools