JavaScript program for minimum product subset of an array is a common problem that arises in the fields of computer science and programming. The problem statement requires us to find the smallest product that can be obtained from any subset of the given arrays.
The minimum product subset of an array is the subset of array elements that yields the smallest possible product. There are several algorithms that can be used to identify this subset, including dynamic programming, greedy algorithms, and branch-and-bound. The choice of algorithm depends on the specific constraints and specifications of the problem at hand.
In this tutorial, we will discuss various ways to solve this problem using the JavaScript programming language. We will introduce basic algorithmic methods and their implementation using JavaScript code snippets. By the end of this tutorial, readers will have a clear understanding of the problem statement and the various ways to solve it using JavaScript.
Problem Statement
Given an array of integers, we need to find the minimum product subset of the array. The product subset of an array is defined as the product of any subset of the array.
For example,
Let us consider the array [2, 3, -1, 4, -2].
The product subset of this array is
[2], [3], [-1], [4], [-2], [2, 3], [2, -1], [2, 4], [2, -2], [3, -1], [3, 4], [3, -2], [-1, 4], [-1, -2], [4, -2], [2, 3, -1], [2, 3, 4], [2, 3, -2], [2, -1, 4], [2, -1, -2], [2, 4, -2], [3, -1, 4], [3, -1, -2], [3, 4, -2], [-1, 4, -2], and [2, 3, -1, 4, -2].
The minimum product subset of this array is [-2].
Now let us discuss the various algorithmic approaches to solving this problem statement and select the most suitable algorithm.
algorithm
The choice of algorithm depends on the specific constraints and prerequisites of the problem.
Greedy Algorithm - The greedy algorithm is a common method for finding the minimum product subset of an array. The basic concept is to start with an initial array element and only append the next element to the subset when a smaller product is generated. Although the greedy algorithm is easy to implement and simple, it does not necessarily provide an optimal solution, and its performance can be significantly slow for large arrays.
Dynamic Programming - Dynamic programming is another algorithm used to solve this problem. It breaks the problem into smaller sub-problems and solves each sub-problem in one go, using the solution to the smaller sub-problem to determine the solution to the larger sub-problem. This approach saves a lot of time and space. Although dynamic programming can guarantee an optimal solution, its implementation may be more complex than a greedy algorithm.
Branch and Bound Algorithm - Another way to identify the minimum product subset of an array is the branch and bound algorithm. It requires exploring multiple possibilities by branching and limiting the search to consider only valid solutions. This algorithm guarantees an optimal solution and can be faster than other algorithms for specific scenarios. Nonetheless, its implementation may be more complex and may require more time and space resources than other algorithms.
In summary, a simple approach requires generating all subsets, calculating the product of each subset, and then returning the minimum product.
A better solution needs to consider the following facts.
-
Step 1 - In the case where there are no zeros and the negative numbers are even, the product of all elements except the largest negative number will yield the result.
Step 2 - In case there are no zeros and the negative numbers are odd, the product of all elements will give the result.
Step 3 - If zero exists and is completely positive, the result is 0. However, in the special case where there are no negative numbers and all other elements are positive, the answer should be the smallest positive number.
Now let us try to understand the above approach with an example of implementing the problem statement using JavaScript.
Example
The program first calculates the count of negative numbers, zero, the maximum negative number, the minimum positive number, and the product of non-zero numbers. It then applies rules based on counting of negative numbers and zeros to return the minimum product subset of the array. The program time complexity is O(n) and the auxiliary space is O(1).
Input 1: a[] = { -1, -1, -2, 4, 3 }; n = 5
Expected output: Minimum subset is [-2, 4, 3], minimum product is -24.
Input 2: a[] = { -1, 0 }; n = 2
Expected output: Minimum subset is [ -1 ], minimum product is -1.
function minProductSubset(a, n) { if (n === 1) { return [a[0], a[0]]; } let negmax = Number.NEGATIVE_INFINITY; let posmin = Number.POSITIVE_INFINITY; let count_neg = 0, count_zero = 0; let subsets = [[]]; for (let i = 0; i < n; i++) { if (a[i] === 0) { count_zero++; continue; } if (a[i] < 0) { count_neg++; negmax = Math.max(negmax, a[i]); } if (a[i] > 0 && a[i] < posmin) { posmin = a[i]; } const subsetsLength = subsets.length; for(let j = 0; j < subsetsLength; j++){ const subset = [...subsets[j], a[i]]; subsets.push(subset); } } if (count_zero === n || (count_neg === 0 && count_zero > 0)) { return [0, 0]; } if (count_neg === 0) { return [posmin, posmin]; } const negativeSubsets = subsets.filter(subset => subset.reduce((acc, cur) => acc * cur, 1) < 0); let minSubset = negativeSubsets[0]; let minProduct = minSubset.reduce((acc, cur) => acc * cur, 1); for (let i = 1; i < negativeSubsets.length; i++) { const product = negativeSubsets[i].reduce((acc, cur) => acc * cur, 1); if (product < minProduct) { minSubset = negativeSubsets[i]; minProduct = product; } } return [minSubset, minProduct]; } let a = [-1, -1, -2, 4, 3]; let n = 5; const [minSubset, minProduct] = minProductSubset(a, n); console.log(`The minimum subset is [ ${minSubset.join(', ')} ] and the minimum product is ${minProduct}.`);
in conclusion
So, in this tutorial, we learned how to find the minimum product subset of an array by following a simple algorithm using JavaScript. The solution involves various criteria such as the number of negative numbers, positive numbers, and zeros present in the array. It uses simple if-else conditions to check these conditions and return the minimum subset of products accordingly. The program time complexity is O(n), and the auxiliary space required is O(1).
The above is the detailed content of JavaScript program for minimum product subset of an array. For more information, please follow other related articles on the PHP Chinese website!
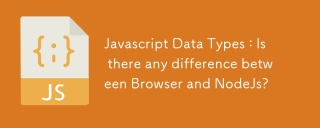
JavaScript core data types are consistent in browsers and Node.js, but are handled differently from the extra types. 1) The global object is window in the browser and global in Node.js. 2) Node.js' unique Buffer object, used to process binary data. 3) There are also differences in performance and time processing, and the code needs to be adjusted according to the environment.
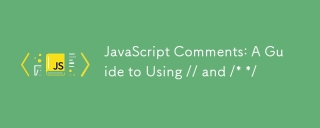
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
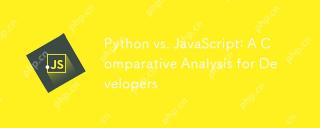
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
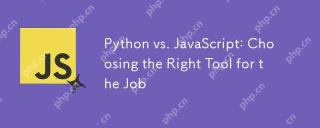
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
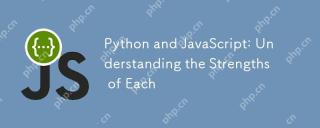
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
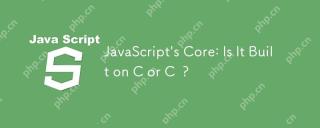
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
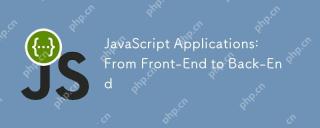
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
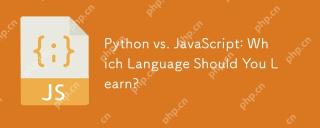
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
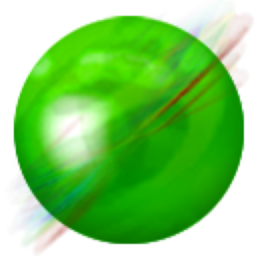
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
