How to implement user rights management through PHP and database
User rights management is a very important function when developing a website or application. Through permission management, you can control the access and operation permissions of different users to the system to ensure the security and stability of the system. In this article, we will introduce how to implement user rights management through PHP and database.
1. Database design
First, we need to design a database to store user information and permission information. Common user rights management usually involves the following tables:
- User table (users): used to store basic user information, such as user name, password, email, etc.
- Role table (roles): used to store basic information of roles, such as role name, role description, etc.
- Permissions table: used to store various permissions of the system, such as accessing pages, performing specific operations, etc.
- User role association table (user_roles): Use this table to establish the relationship between users and roles.
- Role permission association table (role_permissions): Use this table to establish the relationship between roles and permissions.
The following is a simple database design example:
CREATE TABLE users ( id INT(11) PRIMARY KEY AUTO_INCREMENT, username VARCHAR(255) NOT NULL, password VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL ); CREATE TABLE roles ( id INT(11) PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255) NOT NULL, description TEXT ); CREATE TABLE permissions ( id INT(11) PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255) NOT NULL, description TEXT ); CREATE TABLE user_roles ( user_id INT(11) NOT NULL, role_id INT(11) NOT NULL, PRIMARY KEY (user_id, role_id), FOREIGN KEY (user_id) REFERENCES users(id), FOREIGN KEY (role_id) REFERENCES roles(id) ); CREATE TABLE role_permissions ( role_id INT(11) NOT NULL, permission_id INT(11) NOT NULL, PRIMARY KEY (role_id, permission_id), FOREIGN KEY (role_id) REFERENCES roles(id), FOREIGN KEY (permission_id) REFERENCES permissions(id) );
2. Registration and login functions
Next, we need to implement the user registration and login functions. When a user registers, we insert his or her basic information into the user table:
// 注册用户 function registerUser($username, $password, $email) { // 将用户信息插入到用户表中 $query = "INSERT INTO users (username, password, email) VALUES ('$username', '$password', '$email')"; // 执行插入操作 // ... }
When the user logs in, we need to verify whether the user's username and password are correct:
// 用户登录 function loginUser($username, $password) { // 根据用户名查询用户信息 $query = "SELECT * FROM users WHERE username = '$username' LIMIT 1"; // 执行查询操作 // ... // 验证密码是否正确 if ($user && password_verify($password, $user['password'])) { // 登录成功 // 设置用户登录状态等 } else { // 登录失败 // 提示用户用户名或密码错误 } }
3. User roles Management
Next, we need to implement the association between users and roles. When the user registers successfully, a default role is assigned to the user by default. User roles can also be assigned manually through background management.
// 分配用户角色 function assignUserRole($userId, $roleId) { // 将用户角色关联信息插入到用户角色关联表中 $query = "INSERT INTO user_roles (user_id, role_id) VALUES ('$userId', '$roleId')"; // 执行插入操作 // ... }
4. Permission management
Finally, we need to implement the permission management function. We can create various permissions required in the system and assign permissions to roles. When a user logs in to the system, the user's role can be used to determine whether the user has a certain permission.
// 检查用户权限 function checkPermission($userId, $permission) { // 查询用户拥有的角色 $query = "SELECT * FROM user_roles WHERE user_id = '$userId'"; // 执行查询操作 // ... // 查询角色拥有的权限 $query = "SELECT * FROM role_permissions WHERE role_id IN ($roleIds)"; // 执行查询操作 // ... // 判断用户是否拥有指定权限 foreach ($permissions as $p) { if ($p['name'] === $permission) { return true; } } return false; }
Through the above methods, we can achieve basic user rights management functions. When a user accesses a page or performs an operation, he only needs to call the checkPermission
function to determine whether the user has the corresponding permissions.
Summary
This article introduces how to implement user rights management through PHP and database. Through the design of the database and related operations, we can flexibly assign roles and permissions to users, and control users' access and operation permissions. Using specific business scenarios, we can manage and control permissions according to actual needs. User rights management is very important to the security and stability of the system. I hope the content of this article will be helpful to everyone.
The above is the detailed content of How to implement user rights management through PHP and database. For more information, please follow other related articles on the PHP Chinese website!

本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于索引优化器工作原理的相关内容,其中包括了MySQL Server的组成,MySQL优化器选择索引额原理以及SQL成本分析,最后通过 select 查询总结整个查询过程,下面一起来看一下,希望对大家有帮助。
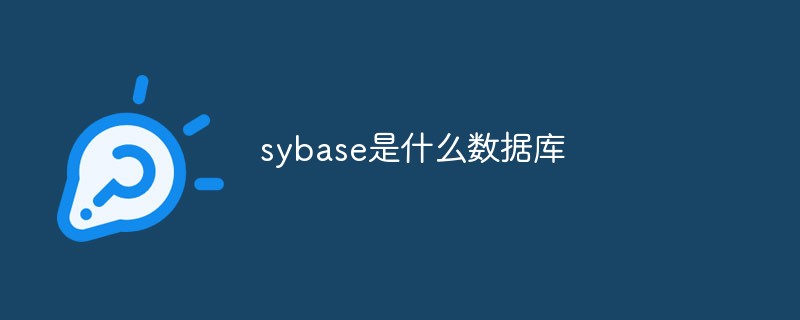
sybase是基于客户/服务器体系结构的数据库,是一个开放的、高性能的、可编程的数据库,可使用事件驱动的触发器、多线索化等来提高性能。
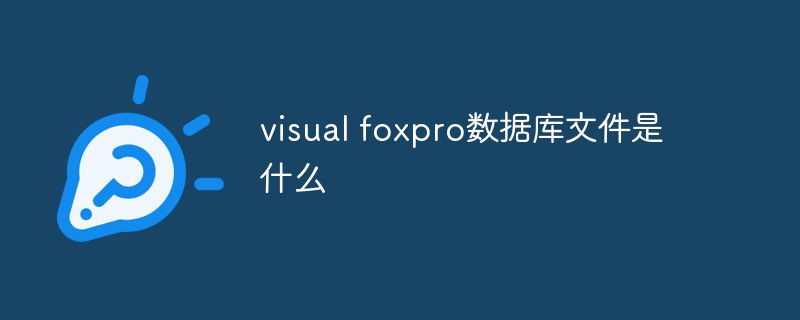
visual foxpro数据库文件是管理数据库对象的系统文件。在VFP中,用户数据是存放在“.DBF”表文件中;VFP的数据库文件(“.DBC”)中不存放用户数据,它只起将属于某一数据库的 数据库表与视图、连接、存储过程等关联起来的作用。
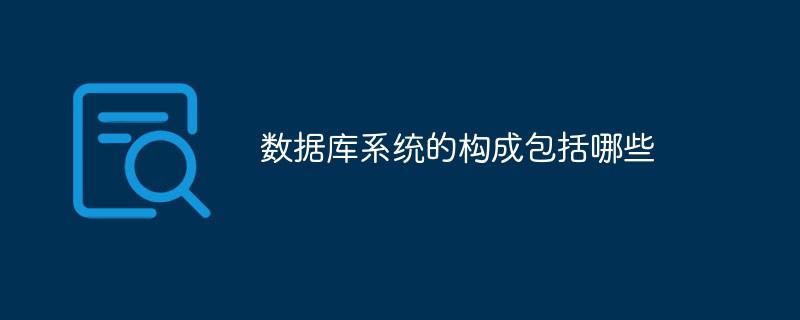
数据库系统由4个部分构成:1、数据库,是指长期存储在计算机内的,有组织,可共享的数据的集合;2、硬件,是指构成计算机系统的各种物理设备,包括存储所需的外部设备;3、软件,包括操作系统、数据库管理系统及应用程序;4、人员,包括系统分析员和数据库设计人员、应用程序员(负责编写使用数据库的应用程序)、最终用户(利用接口或查询语言访问数据库)、数据库管理员(负责数据库的总体信息控制)。
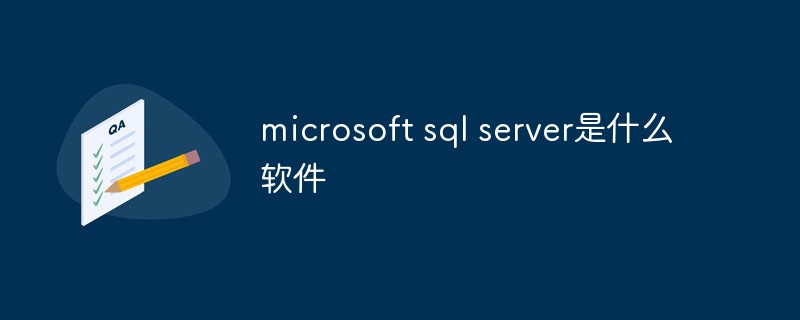
microsoft sql server是Microsoft公司推出的关系型数据库管理系统,是一个全面的数据库平台,使用集成的商业智能(BI)工具提供了企业级的数据管理,具有使用方便可伸缩性好与相关软件集成程度高等优点。SQL Server数据库引擎为关系型数据和结构化数据提供了更安全可靠的存储功能,使用户可以构建和管理用于业务的高可用和高性能的数据应用程序。
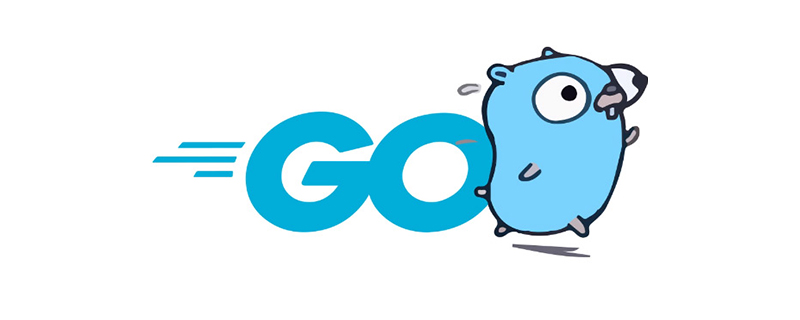
go语言可以写数据库。Go语言和其他语言不同的地方是,Go官方没有提供数据库驱动,而是编写了开发数据库驱动的标准接口,开发者可以根据定义的接口来开发相应的数据库驱动;这样做的好处在于,只要是按照标准接口开发的代码,以后迁移数据库时,不需要做任何修改,极大方便了后期的架构调整。
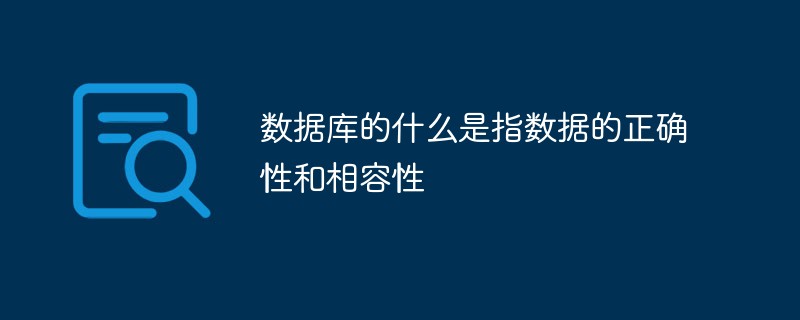
数据库的“完整性”是指数据的正确性和相容性。完整性是指数据库中数据在逻辑上的一致性、正确性、有效性和相容性。完整性对于数据库系统的重要性:1、数据库完整性约束能够防止合法用户使用数据库时向数据库中添加不合语义的数据;2、合理的数据库完整性设计,能够同时兼顾数据库的完整性和系统的效能;3、完善的数据库完整性有助于尽早发现应用软件的错误。
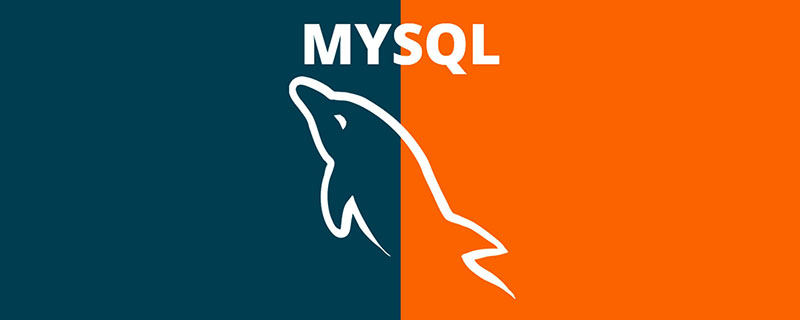
mysql查询为什么会慢,关于这个问题,在实际开发经常会遇到,而面试中,也是个高频题。遇到这种问题,我们一般也会想到是因为索引。那除开索引之外,还有哪些因素会导致数据库查询变慢呢?


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
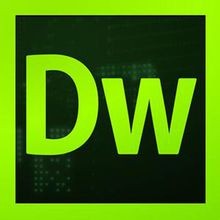
Dreamweaver CS6
Visual web development tools
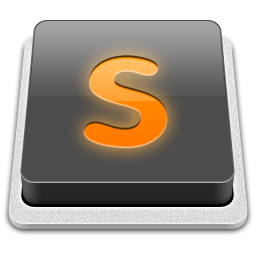
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 English version
Recommended: Win version, supports code prompts!
