Python program: Trim string from right side of string
In Python, we have a predefined function rstrip() to remove the characters on the right side. This means it will remove spaces on the right side of the string.
Let us take an example to understand how to trim from the left side of a string.
Remove the right string LESS from the given string "WIRELESS" and get the resulting value as "WIRE".
In the given string "kingdom", delete the string dom on the right side, and the result value is "king".
grammar
The syntax used in the following examples is −
isspace()
This is a predefined method in Python for allowing whitespace, newlines, or spaces in characters.
rstrip("parameter as a string")
This is a predefined method used in Python that accepts a character as argument and removes that character from the right side of the string.
endswith()
This is a built-in method in Python that returns true if the string ends with a specific value.
Example 1
In this program, we store the input string in the variable ‘str’. The variable 'i' is then initialized to the value 5, and characters after the 5th index will be trimmed later. Next, the variable ‘str’ is iterated over the variable ‘char’ using a for loop. Then use the if statement to search for spaces using the isspace() method. If a space is not found in the string, it breaks the loop and the variable "i" is decremented for each white space character. Now we trim the characters using str[:i] and store the value in variable 'trim_str'. Finally, we use the variable 'trim_str' to print the result.
#trim the string from the right str = "UNIVERSITY" i = 5 for char in str: if not char.isspace(): break i -= 1 trim_str = str[:i] #The use before slicing removes the right string. print("Trim the string of", i," characters from right:", trim_str)
Output
Trim the string of 5 characters from right: UNIVE
Example 2
In this program, we store the input string in the variable ‘my_str’. We then remove the character "a" from the right side of the string and store it in the variable 'trim_str'. Finally, we use the variable 'trim_str' to print the result.
#Trim the string from right my_str = "aaaaa!King!aaaaa" trim_str = my_str.rstrip("a") print(trim_str)
Output
aaaaa!King!The Chinese translation of
Example 3
is:Example 3
In this program, we will store the input string in the variable str_name. The correct deletion string is then stored in the variable del_suffix. Then use an if statement to check the condition for removing the right side of the string using the built-in method endswith(). Next, use the replace() method to remove the given string and store it in the variable str_name. Finally, we use the str_name variable to print the output.
str_name = "abcdefghi" del_suffix = "ghi" if str_name.endswith(del_suffix): str_name = str_name.replace(del_suffix, "") print("After deleting the suffix from left side:",str_name)
Output
After deleting the suffix from left side: abcdefThe Chinese translation of
Example 4
is:Example 4
In the following program, we store the input string in the variable s. Then use the built-in method removesuffix() to set a string named 'iop', remove the string from the right and in the print() function.
s = 'qwertyuiop' print(s.removesuffix('iop'))
Output
qwertyu
in conclusion
We understand the difference between these two examples by trimming the string from the left. We see several different methods used in the examples, including isspace(), rstrip(), endswith(), and slicing techniques. Slicing techniques are often used to trim strings from the right side.
The above is the detailed content of Python program: Trim string from right side of string. For more information, please follow other related articles on the PHP Chinese website!
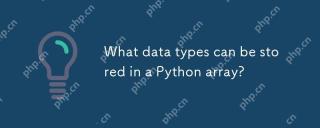
Pythonlistscanstoreanydatatype,arraymodulearraysstoreonetype,andNumPyarraysarefornumericalcomputations.1)Listsareversatilebutlessmemory-efficient.2)Arraymodulearraysarememory-efficientforhomogeneousdata.3)NumPyarraysareoptimizedforperformanceinscient
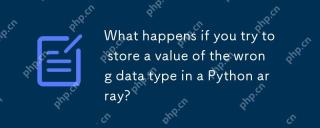
WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
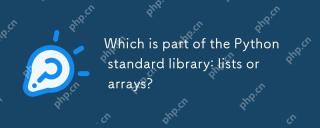
Pythonlistsarepartofthestandardlibrary,whilearraysarenot.Listsarebuilt-in,versatile,andusedforstoringcollections,whereasarraysareprovidedbythearraymoduleandlesscommonlyusedduetolimitedfunctionality.
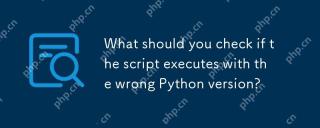
ThescriptisrunningwiththewrongPythonversionduetoincorrectdefaultinterpretersettings.Tofixthis:1)CheckthedefaultPythonversionusingpython--versionorpython3--version.2)Usevirtualenvironmentsbycreatingonewithpython3.9-mvenvmyenv,activatingit,andverifying
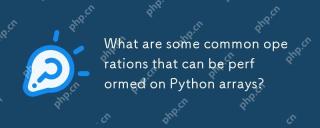
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
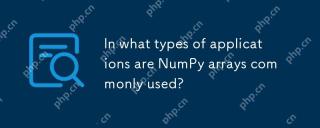
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
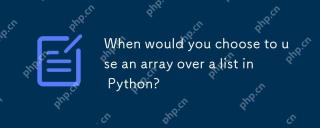
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf
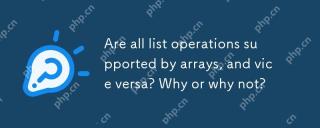
No,notalllistoperationsaresupportedbyarrays,andviceversa.1)Arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,whichimpactsperformance.2)Listsdonotguaranteeconstanttimecomplexityfordirectaccesslikearraysdo.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
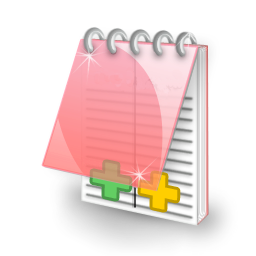
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
