JavaScript functions can be called with or without parameters. When called with arguments, the function will be executed using the arguments passed in as values. When called without arguments, the function will be executed without passing in any arguments.
In some cases, a function needs to be called with some parameters (but not all). This can be done using the Function.prototype.apply method or using the spread operator ( ... ).
Use Function.prototype.apply method
The Function.prototype.apply method can be used to call a function with some parameters, but not all parameters. The first argument to the apply method is the this value, followed by the array of arguments to be passed to the function.
grammar
apply(thisArg) apply(thisArg, argsArray)
parameter
thisArg - The this value provided for the calling function. If the function is not in strict mode, null and undefined will be replaced with global and the original value will be converted to an object.
-
argsArray Optional - x Array-like object specifying the arguments with which the function should be called, or null or undefined if arguments should not be supplied to the function.
Example
For example, consider the following code -
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> function add(a, b) { return a + b; } document.getElementById("result").innerHTML = add.apply(null, [1, 2]) </script> </body> </html>
This value is passed to the apply method as the first parameter, and the parameter array is passed as the second parameter. The function is called using the this value and the arguments passed in as values.
The Function.prototype.apply method is a powerful way to call a function with some arguments (but not all arguments). By using the apply method, you avoid hardcoding the parameters to be passed to the function.
Use spread operator
The spread operator ( ... ) can be used to call a function with some arguments (but not all arguments).
The spread operator expands a parameter array into individual parameters.
For example, consider the following code -
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> function add(a, b) { return a + b; } document.getElementById("result").innerHTML = add(...[1, 2]) </script> </body> </html>
The parameter array is expanded into individual parameters and the function is called with these parameters.
Use bind() method
Function.prototype.bind() method can be used to create a new function that calls the original function and prepends some parameters.
For example, consider the following code -
<!doctype html> <html> <head> <title>Examples</title> </head> <body> <div id="result"></div> <script> function add(a, b) { return a + b; } const add1 = add.bind(null, 1); document.getElementById("result").innerHTML = add1(2) </script> </body> </html>
The first parameter of the bind method is the this value, and the remaining parameters are used as parameters of the function when calling the function. In this example, the this value is passed as null and the value 1 is passed as the first argument to the add function.
The bind method returns a new function that is called with the given parameters. In this example, the new function is called with the value 2, and the add function is called with the values 1 and 2.
JavaScript functions can be called with or without parameters. In some cases, a function needs to be called with some arguments (but not all). This can be done using the Function.prototype.apply method or using the spread operator ( ... ).
The above is the detailed content of How to call a function with partial prefix parameters in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
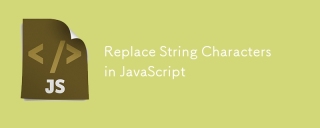
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
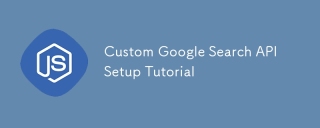
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
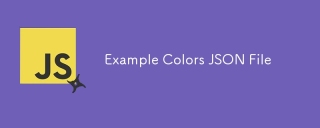
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
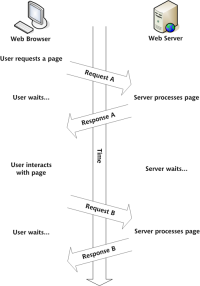
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
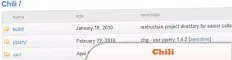
Enhance Your Code Presentation: 10 Syntax Highlighters for Developers Sharing code snippets on your website or blog is a common practice for developers. Choosing the right syntax highlighter can significantly improve readability and visual appeal. T
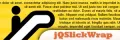
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
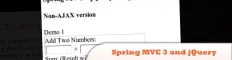
This article presents a curated selection of over 10 tutorials on JavaScript and jQuery Model-View-Controller (MVC) frameworks, perfect for boosting your web development skills in the new year. These tutorials cover a range of topics, from foundatio
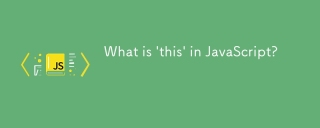
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
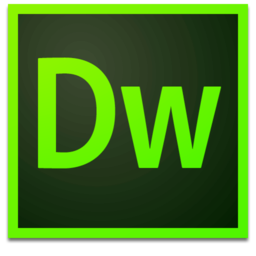
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
