


How to use forced inheritance to proxy final classes in Java to achieve better code maintenance and upgrades?
How to use forced inheritance to proxy final classes in Java to achieve better code maintenance and upgrades?
Introduction:
In Java programming, we often encounter situations where we need to inherit and rewrite some classes. However, sometimes the class we want to inherit is declared final and cannot be inherited, which brings certain troubles to code maintenance and upgrades. This article will introduce a solution to achieve better code maintenance and upgrades by forcing inheritance of proxy final classes.
Text:
In Java, if a class is declared final, it means that the class cannot be inherited by other classes. This limits the flexibility and scalability of the code to a certain extent. However, we can solve this problem by forcing inheritance of the proxy final class. Specifically, we can create a proxy class in which the call to the final class is implemented and perform some additional operations if necessary.
Next, we will use an example to illustrate how to use forced inheritance to proxy final classes to achieve better code maintenance and upgrades.
Suppose we have a final class FinalClass
, in which there is an action()
method that needs to be inherited and overridden. However, due to the limitations of FinalClass
, we cannot directly inherit and override this method. The solution is to create a proxy class ProxyClass
that inherits FinalClass
and overrides the action()
method.
The following is the sample code:
public final class FinalClass { public void action() { System.out.println("FinalClass action"); } } public class ProxyClass extends FinalClass { private FinalClass finalClass; public ProxyClass(FinalClass finalClass) { this.finalClass = finalClass; } @Override public void action() { // 可以在这里进行一些额外的操作 System.out.println("Before action"); // 调用原始类的方法 finalClass.action(); // 可以在这里进行一些额外的操作 System.out.println("After action"); } } public class Main { public static void main(String[] args) { FinalClass finalClass = new FinalClass(); ProxyClass proxyClass = new ProxyClass(finalClass); proxyClass.action(); } }
In the above example, we created a FinalClass
as the proxied final class, which has an action()
method. Then, we created a ProxyClass
as a proxy class, which inherits the FinalClass
and overrides the action()
method. In the action()
method, we implement the call to the final class by calling the method of the original class, and perform some additional operations if necessary.
In the main()
method of the Main
class, we instantiate the FinalClass
and ProxyClass
objects, and The action()
method was called.
Through the above implementation, we have successfully used forced inheritance of proxy final classes to achieve better code maintenance and upgrades. We can override the methods of the original class in the proxy class and perform some additional operations if necessary. This approach makes code maintenance and upgrades more flexible and controllable.
Conclusion:
By using forced inheritance to proxy final classes in Java, we can solve the problem that final classes cannot be inherited and rewritten, and achieve better code maintenance and upgrades. By creating a proxy class and overriding the methods of the original class in it, we can increase the flexibility and scalability of the code to a certain extent. I hope the introduction in this article will be helpful when you encounter similar problems in Java programming.
The above is the detailed content of How to use forced inheritance to proxy final classes in Java to achieve better code maintenance and upgrades?. For more information, please follow other related articles on the PHP Chinese website!
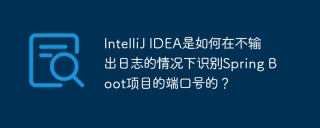
Start Spring using IntelliJIDEAUltimate version...
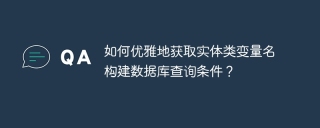
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
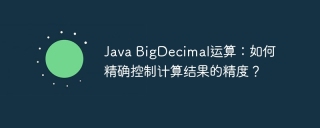
Java...
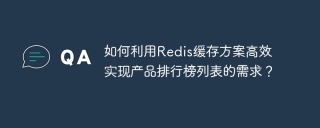
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
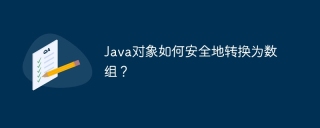
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
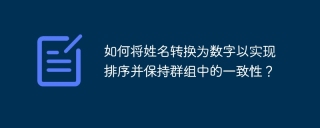
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
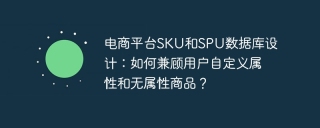
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
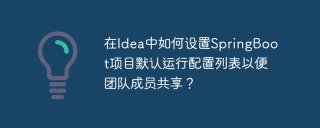
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
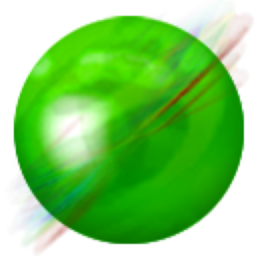
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment