


Let's see how to count the number of triangles on a plane given n points, and limit the collinear points to no more than two.
Calculating the number of triangles in a plane with no more than two collinear points is a typical problem in computational geometry, which is applied in computer graphics, image processing, and other fields of computer science.
For example, when creating a 2D image from a 3D scene in 3D graphics, the problem may arise of calculating triangles in a plane with no more than two collinear points. In this case, the triangle counting process can be used to determine how many triangles are present in the final 2D image after projecting the 3D scene onto a plane. This allows you to determine the complexity of the scene and increase rendering speed.
In image processing, we may want to count the number of unique objects or shapes in an image, this problem is helpful. In this case, we can represent the image as a collection of points on a plane, and then we can count the number of triangles that can be created between these points by applying triangle counting techniques. We can determine the approximate number of different items or shapes in an image by counting the number of triangles formed.
illustrate
Let us understand this problem through a few examples and try to solve it.
The purpose is to determine how many triangles are formed on a plane with n points such that no more than two points are collinear.
Example -
Assume N is the number of points on the plane.
N = 3
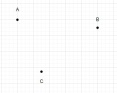
Using these points we can only draw a triangle.
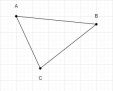
Therefore, the total number of triangles formed using 3 points is 1.
Let N = 4
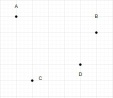
Let's draw a triangle using these four points.
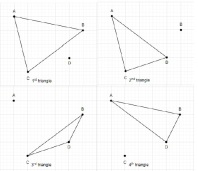
The total number of triangles formed using 4 points is 4.
Let's look at some of the math involved in calculating the number of triangles. This can be obtained using permutations and combinations. To build a triangle, you need 3 points out of the total at a time.
Thus, if a plane contains n points and no more than two of them are collinear, the number of triangles in the plane is given by the following formula.
$$\mathrm{n_{C_{3}}\:=\:\frac{n(n-1)\:(n-2)}{6}}$$
method
The program finds the number of triangles in the plane if no more than two points are collinear, using the following algorithm.
Take the number of points on the plane as input and limit it to no more than two collinear points.
Use the above formula to calculate the total number of triangles.
Print the total number of triangles as output.
Example
C program to calculate the number of triangles in a plane if no more than two points are collinear.
#include <iostream> using namespace std; int main() { int number_of_points = 4; int number_of_triangle; number_of_triangle = number_of_points * (number_of_points - 1) * (number_of_points - 2) / 6; cout << "Total number of triangles formed using " << number_of_points<< " points = " << number_of_triangle << endl; return 0; }
Output
Total number of triangles formed using 4 points = 4
Complexity
Time complexity: O(1) because this code performs a fixed number of calculations regardless of the input size.
Space Complexity: O(1) because the code uses a fixed number of variables to store input values and results regardless of the size of the input.
in conclusion
In this article, we try to explain the method to find the total number of possible triangles with n given points, with the constraint that no two points are collinear. I hope this article helps you learn this concept better.
The above is the detailed content of If no more than two points in the plane are collinear, what is the number of triangles?. For more information, please follow other related articles on the PHP Chinese website!
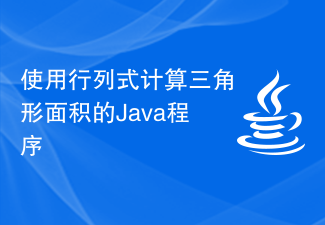
简介使用行列式计算三角形面积的Java程序是一个简洁高效的程序,可以根据给定三个顶点的坐标来计算三角形的面积。该程序对于学习或使用几何的任何人都非常有用,因为它演示了如何在Java中使用基本算术和代数计算,以及如何使用Scanner类读取用户输入。程序提示用户输入三角形三个点的坐标,然后将其读入并用于计算坐标矩阵的行列式。使用行列式的绝对值来确保面积始终为正,然后使用公式计算三角形的面积并显示给用户。该程序可以轻松修改以接受不同格式的输入或执行附加计算,使其成为几何计算的多功能工具。决定因素行列
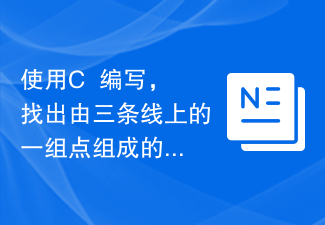
现在我们得到了3行中存在的几个点;例如,我们需要找出这些点可以形成多少个三角形Input:m=3,n=4,k=5Output:205Input:m=2,n=2,k=1Output:10我们将应用一些组合数学来解决这个问题,并制定一些公式来解决这个问题。寻找解决方案的方法在这种方法中,我们将设计一个公式:将组合学应用于当前情况,这个公式将为我们提供结果。上述方法的C++代码这是我们可以用作求解的输入的C++语法给定的问题-示例#include<bits/stdc++.h>#define
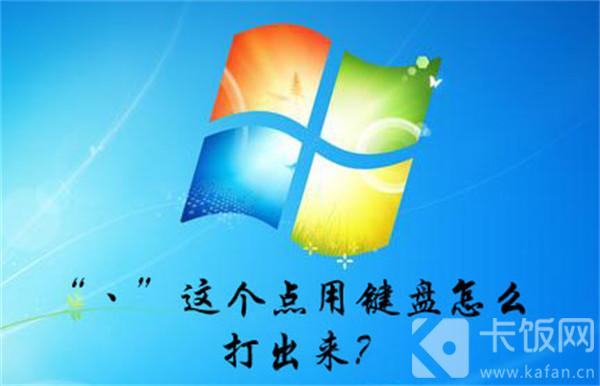
在使用键盘的打字的时候会有很多的用户比较好奇“丶”这个点用键盘是怎么打出来的?那么下面就来看一下小编给大家带来的键盘上打出这个“丶”符号的方法吧。一、“丶”点用键盘打出打出直接输入【dian】在选择栏上就会看到【丶】的标点符号。二、特殊符号在搜狗拼音输入法中,当切换至中文状态后,按下v键会出现一些特殊的符号。这些符号包括数字(如:v123)、日期(如:v2013/1/1)、算式(如:v1+1)和函数(如:v2~3)。这些符号可以方便地输入各种不同的信息。2.接着再按下数字键,0到9随便一个都可以
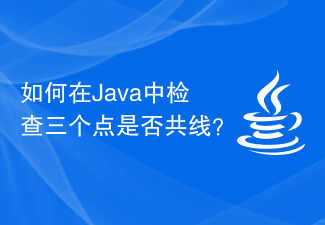
如果三个点都位于一条直线上,则称这三个点共线。如果这些点不在同一条直线上,则它们不是共线点。这意味着如果三个点(x1,y1),(x2,y2),(x3,y3)在同一条直线上,则它们是共线的。其中,x1、y1、x2、y2、x3、y3是x轴和y轴上的点,(x1,y1)、(x2,y2)、(x3,y3)是坐标。数学上,有两种方法可以确定三个点是否共线。通过使用点求三角形的面积,如果三角形的面积为零,则三个点共线。Formulatofindareaoftriangle=0。5*[x1*(y2-y3)+x2*
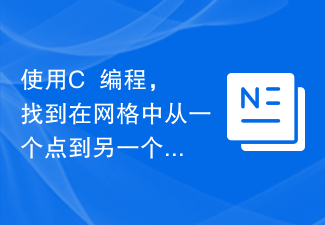
在本文中,我们给出了一个问题,我们需要找到从点A到点B的总路径数,其中A和B是固定点,即A是网格中的左上角点,B是网格中的右下角点,例如−Input:N=5Output:252Input:N=4Output:70Input:N=3Output:20在给定的问题中,我们可以通过简单的观察来形式化答案并得出结果。寻找解决方案的方法在这种方法中,我们通过观察得出一个公式,即从A到B穿过网格时,我们需要向右行进n次,向下行进n次,这意味着我们需要找到所有可能的路径组合,因此我们得到了
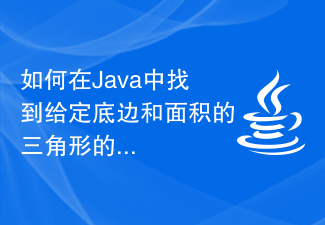
我们有三角形的面积'a'和底边'b'。根据问题陈述,我们需要使用Java编程语言来找到最小高度'h'。正如我们所知,当给定底边和高度时,三角形的面积为−$$\mathrm{面积\:=\:\frac{1}{2}\:*\:底边\:*\:高度}$$通过使用上述公式,我们可以从中得到高度-height=(2*area)/base然后通过使用内置的ceil()方法,我们可以得到最小高度。展示一些实例给你看Instance-1的中文翻译为:实例-1假设给定面积=12和底边=6然后使用公式
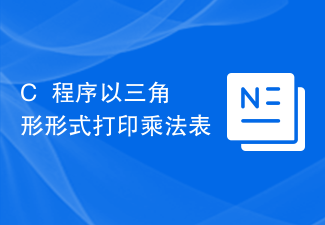
要以表格或图形形式记住一些基本乘法结果,请使用乘法表。本文将介绍如何用C++生成一个看起来像直角三角形的乘法表。在少数可以轻松记住大量结果的情况下,三角形表示法是有效的。在这种格式中,表格逐行、逐列显示,每行仅包含填充该列的条目。为了解决这个问题,我们需要C++中的基本循环语句。为了以三角形方式显示数字,我们需要嵌套循环来逐行打印每一行。我们将看到解决这个问题的方法。让我们看看算法和实现以便更好地理解。算法取我们想要的乘法表的行数,假设为n。对于从1到n的i,执行以下操作。对于范围从1到i的j,
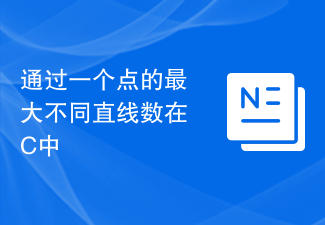
我们得到每条线的数字N和两个点(x1,y1)和(x2,y2)的坐标。目标是从给定的直线中找到可以穿过单个点的最大直线数,使得没有两条直线相互覆盖,并且不执行旋转。我们将把直线表示为(对)m,c)其中y=mx+c,m是斜率m=y2-y1/x2-x1给定c1!=c2,具有相同m的线是平行的。我们将计算不同的坡度(米)。对于垂直线,如果x1=x2,则斜率=INT_MAX,否则为m。让我们通过示例来理解。输入 Line1(x1,y1)=(4,10)(x2,y2)=(2,2)Line2(x1,y1)=(2


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
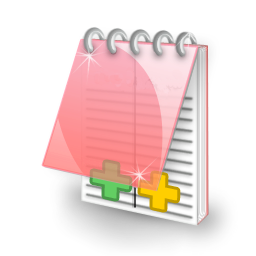
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool