How to select random elements in a PHP array
Randomly selecting elements in an array is a common requirement in programming. In PHP we have many ways to achieve this goal. This article will introduce several commonly used methods and sample code.
Method 1: Use array_rand() function
array_rand() function is a function in PHP used to randomly select key names from an array. This function returns a random key name, which we can use to get the corresponding element.
The following is a sample code:
$array = array("apple", "banana", "orange", "grape", "kiwi"); $randomKey = array_rand($array); $randomElement = $array[$randomKey]; echo $randomElement;
In the above code, we define an array containing five fruits. Then, we use the array_rand() function to randomly select a key and get the corresponding element through that key. Finally, we print out the randomly selected fruits.
Method 2: Use the shuffle() function
The shuffle() function is a function in PHP used to disrupt the order of array elements. By shuffling the order of the array, we can randomly select elements in the array.
The following is a sample code:
$array = array("apple", "banana", "orange", "grape", "kiwi"); shuffle($array); $randomElement = $array[0]; echo $randomElement;
In the above code, we first define an array containing five fruits. Then, we use the shuffle() function to shuffle the order of the array elements. Next, we get the first element in the shuffled array, which is the randomly selected fruit, and print it out.
Method 3: Use mt_rand() function and count() function
mt_rand() function is a function used to generate random numbers in PHP. We can combine the count() function to generate a random index, and then use this index to get the corresponding array element.
The following is a sample code:
$array = array("apple", "banana", "orange", "grape", "kiwi"); $randomIndex = mt_rand(0, count($array)-1); $randomElement = $array[$randomIndex]; echo $randomElement;
In the above code, we first define an array containing five fruits. We then use the count() function to get the length of the array and the mt_rand() function to generate a random index from 0 to the array length minus one. Finally, we obtain the corresponding array element by random index and print the output.
Summary:
This article introduces three common methods for selecting random elements in PHP arrays. Use the array_rand() function to directly obtain the element corresponding to the random key name, and use the shuffle() function to shuffle the order of the array elements, and then select the first element as the randomly selected element. In addition, combining the mt_rand() function and the count() function can generate a random index, and then use this index to obtain the corresponding array element. Which method to use depends on specific scene needs and personal preference.
The above is the detailed content of How to select random elements in PHP array. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
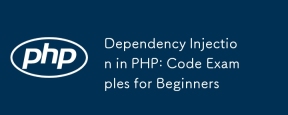
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
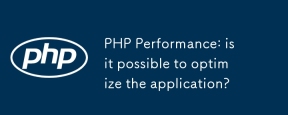
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
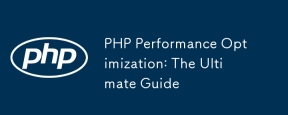
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
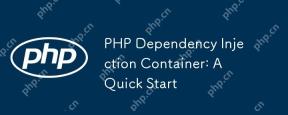
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
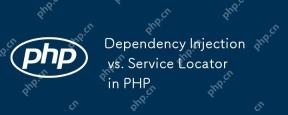
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
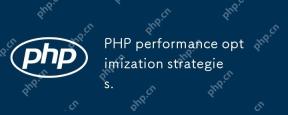
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
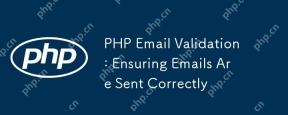
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
