The fluent query builder in Laravel is an interface responsible for creating and running database queries. The query builder works well with all databases supported by Laravel and can be used to perform almost any database operation.
The advantage of using the fluent query builder is that it protects against SQL injection attacks. It utilizes PDO parameter binding and you are free to send strings as needed.
Fluid Query Builder Supports many methods such as count, min, max, avg, sum to get summary values from the table.
Now let’s see how to use the fluent query builder to get the count in a select query. To use the fluent query builder, use the database facade class as shown below
use Illuminate\Support\Facades\DB;
Now let's check a few examples to get counts in select queries. Suppose we create a table named Students using the following query
CREATE TABLE students( id INTEGER NOT NULL PRIMARY KEY, name VARCHAR(15) NOT NULL, email VARCHAR(20) NOT NULL, created_at VARCHAR(27), updated_at VARCHAR(27), address VARCHAR(30) NOT NULL );
and fill it as shown below -
+----+---------------+------------------+-----------------------------+-----------------------------+---------+ | id | name | email | created_at | updated_at | address | +----+---------------+------------------+-----------------------------+-----------------------------+---------+ | 1 | Siya Khan | siya@gmail.com | 2022-05-01T13:45:55.000000Z | 2022-05-01T13:45:55.000000Z | Xyz | | 2 | Rehan Khan | rehan@gmail.com | 2022-05-01T13:49:50.000000Z | 2022-05-01T13:49:50.000000Z | Xyz | | 3 | Rehan Khan | rehan@gmail.com | NULL | NULL | testing | | 4 | Rehan | rehan@gmail.com | NULL | NULL | abcd | +----+---------------+------------------+-----------------------------+-----------------------------+---------+
The number of records in the table is 4.
Example 1
In the following example, we use students in DB::table. The count() method is responsible for returning the total records present in the table.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\DB; class StudentController extends Controller{ public function index() { $count = DB::table('students')->count(); echo "The count of students table is :".$count; } }
Output
The output of the above example is -
The count of students table is :4
Example 2
In this example, selectRaw() will be used to get the total number of records present in the table.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\DB; class StudentController extends Controller { public function index() { $count = DB::table('students')->selectRaw('count(id) as cnt')->pluck('cnt'); echo "The count of students table is :".$count; } }
The column ID is used inside the count() method of the selectRaw() method, and uses pluck to get the count.
Output
The output of the above code is -
The count of students table is :[4]
Example 3
This example will use the selectRaw() method. Suppose you want to count the number of names like Rehan Khan. Let's see how to use selectRaw() with count() method
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\DB; class StudentController extends Controller { public function index() { $count = DB::table('students')-> where('name', 'Rehan Khan')-> selectRaw('count(id) as cnt')->pluck('cnt'); echo "The count of name:Rehan Khan in students table is :".$count; } }
In the above example, we want to find the table: Student named Rehan Khan的人数b>. So write the query Just to get it.
DB::table('students')->where('name', 'Rehan Khan')->selectRaw('count(id) as cnt')->pluck('cnt');
We used the selectRaw() method to count records from the where filter. Finally, use the pluck() method to obtain the count value.
Output
The output of the above code is -
The count of name:Rehan Khan in students table is :[2]
Example 4
If you are planning to use the count() method to check if any record exists in the table, alternatively you can use exists() or doesntExist() method as shown below -
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\DB; class StudentController extends Controller{ public function index() { if (DB::table('students')->where('name', 'Rehan Khan')->exists()) { echo "Record with name Rehan Khan Exists in the table :students"; } } }
Output
The output of the above code is -
Record with name Rehan Khan Exists in the table :students
Example 5
Use the doesntExist() method to check if there are records available in the given table.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\DB; class StudentController extends Controller{ public function index() { if (DB::table('students')->where('name', 'Neha Khan')->doesntExist()) { echo "Record with name Rehan Khan Does not Exists in the table :students"; } else { echo "Record with name Rehan Khan Exists in the table :students"; } } }
Output
The output of the above code is -
Record with name Rehan Khan Does not Exists in the table :students
The above is the detailed content of How to select count using Laravel's fluent query builder?. For more information, please follow other related articles on the PHP Chinese website!
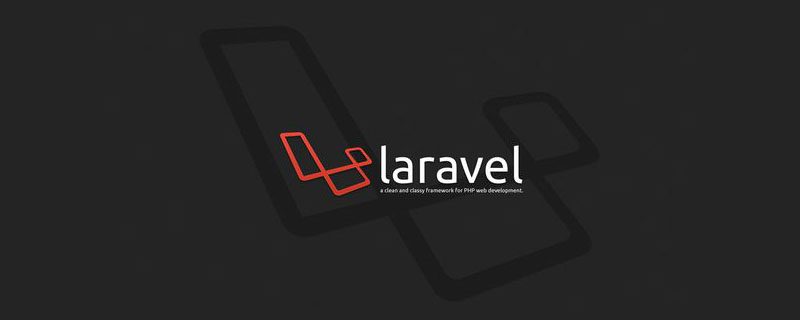
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于单点登录的相关问题,单点登录是指在多个应用系统中,用户只需要登录一次就可以访问所有相互信任的应用系统,下面一起来看一下,希望对大家有帮助。
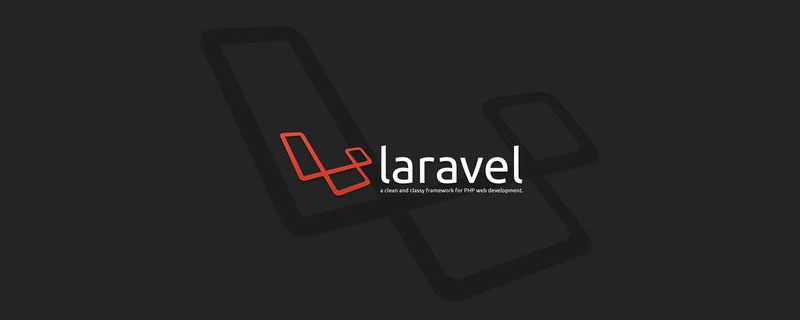
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于Laravel的生命周期相关问题,Laravel 的生命周期从public\index.php开始,从public\index.php结束,希望对大家有帮助。
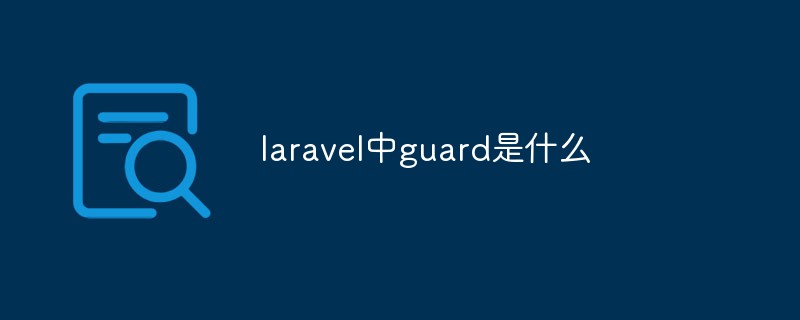
在laravel中,guard是一个用于用户认证的插件;guard的作用就是处理认证判断每一个请求,从数据库中读取数据和用户输入的对比,调用是否登录过或者允许通过的,并且Guard能非常灵活的构建一套自己的认证体系。
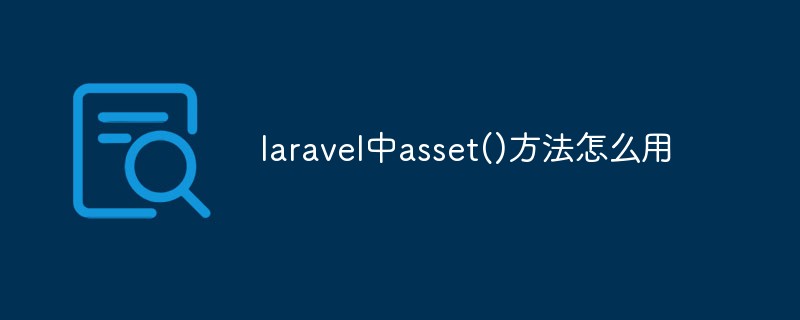
laravel中asset()方法的用法:1、用于引入静态文件,语法为“src="{{asset(‘需要引入的文件路径’)}}"”;2、用于给当前请求的scheme前端资源生成一个url,语法为“$url = asset('前端资源')”。
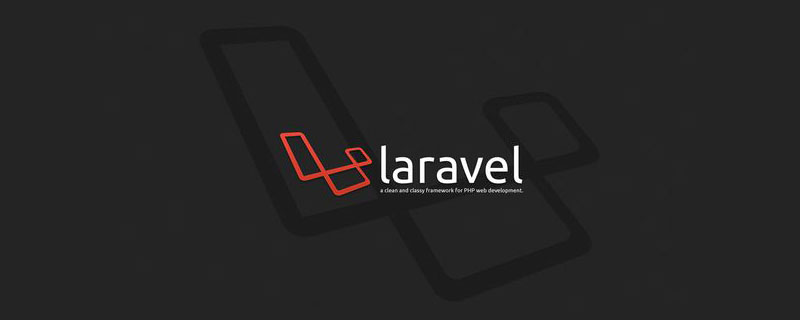
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于使用中间件记录用户请求日志的相关问题,包括了创建中间件、注册中间件、记录用户访问等等内容,下面一起来看一下,希望对大家有帮助。
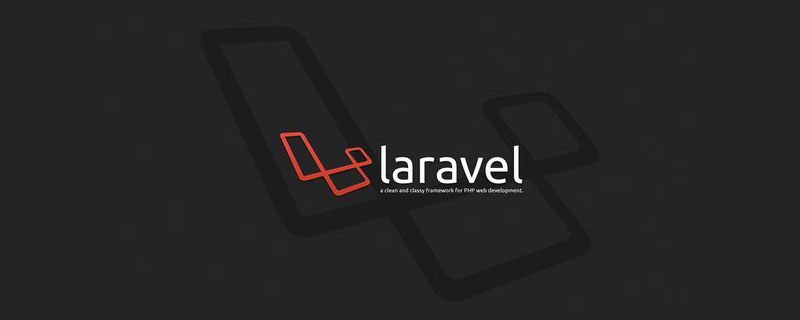
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于中间件的相关问题,包括了什么是中间件、自定义中间件等等,中间件为过滤进入应用的 HTTP 请求提供了一套便利的机制,下面一起来看一下,希望对大家有帮助。
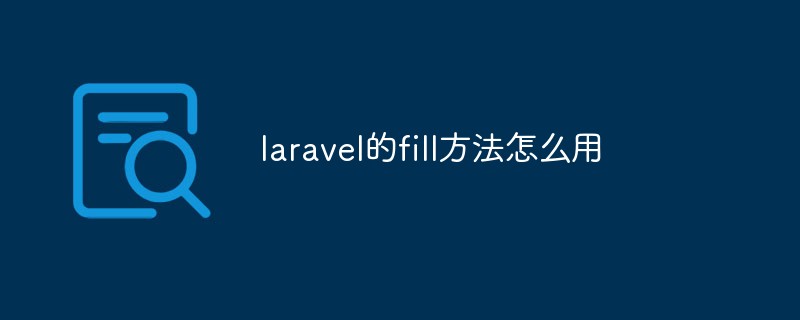
在laravel中,fill方法是一个给Eloquent实例赋值属性的方法,该方法可以理解为用于过滤前端传输过来的与模型中对应的多余字段;当调用该方法时,会先去检测当前Model的状态,根据fillable数组的设置,Model会处于不同的状态。
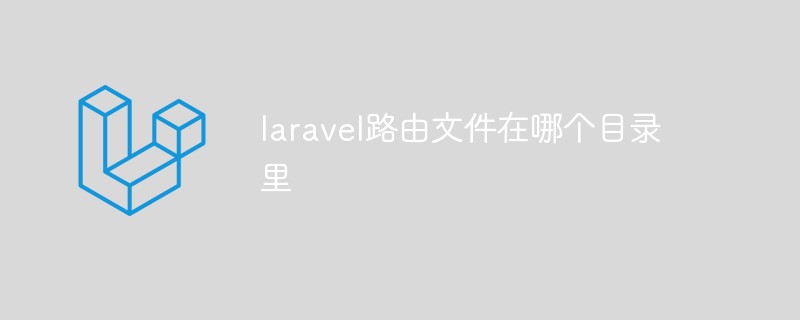
laravel路由文件在“routes”目录里。Laravel中所有的路由文件定义在routes目录下,它里面的内容会自动被框架加载;该目录下默认有四个路由文件用于给不同的入口使用:web.php、api.php、console.php等。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
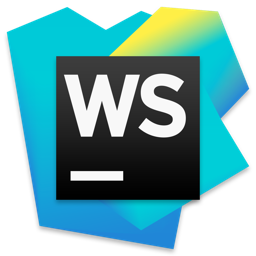
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
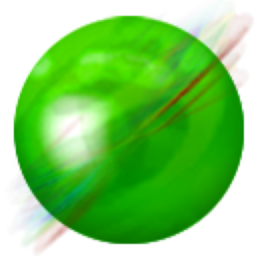
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
