You probably already know that JavaScript is a single-threaded programming language. This means that JavaScript runs on a single main thread in a web browser or Node.js. Running on a single main thread means running only one piece of JavaScript code at a time.
The event loop in JavaScript plays an important role in determining how code is executed on the main thread. The event loop is responsible for things like the execution of code and the collection and processing of events. It also handles the execution of any queued subtasks.
In this tutorial, you will learn the basics of event loops in JavaScript.
How the event loop works
In order to understand how the event loop works, you need to understand three important terms.
Stack
The call stack is simply a stack of function calls that tracks the execution context of a function. The stack follows the last-in-first-out (LIFO) principle, which means that the most recently called function will be the first to be executed.
queue
A queue contains a series of tasks executed by JavaScript. Tasks in this queue may cause functions to be called, which are then placed on the stack. Processing of the queue begins only when the stack is empty. Items in the queue follow a first-in, first-out (FIFO) principle. This means that the oldest tasks will be completed first.
heap
The heap is basically a large area of memory where objects are stored and allocated. Its main purpose is to store data that may be used by functions on the stack.
Basically, JavaScript is single-threaded and executes one function at a time. This single function is placed on the stack. The function can also contain other nested functions, which will be placed higher in the stack. The stack follows the LIFO principle, so the most recently called nested function will be executed first.
Asynchronous tasks such as API requests or timers will be added to the queue for later execution. The JavaScript engine starts executing tasks in the queue when it is idle.
Consider the following example:
function helloWorld() { console.log("Hello, World!"); } function helloPerson(name) { console.log(`Hello, ${name}!`); } function helloTeam() { console.log("Hello, Team!"); helloPerson("Monty"); } function byeWorld() { console.log("Bye, World!"); } helloWorld(); helloTeam(); byeWorld(); /* Outputs: Hello, World! Hello, Team! Hello, Monty! Bye, World! */
Let's see what the stack and queue would look like if we ran the code above.
Call the helloWorld()
function and put it on the stack. It logs that Hello, World! has completed its execution, so it is taken off the stack. Next the helloTeam()
function is called and placed on the stack. During execution, we log Hello, Team! and call helloPerson()
. The execution of helloTeam()
has not finished yet, so it stays on the stack while helloPerson()
is placed on top of it.
The last-in-first-out principle stipulates that helloPerson()
is executed now. This will log Hello, Monty! to the console, thus completing its execution, and helloPerson()
will be popped off the stack. After that, the helloTeam()
function will pop off the stack, and we finally reach byeWorld()
. It will record Goodbye, world! and then disappear from the stack.
The queue is always empty.
Now, consider a slight variation of the above code:
function helloWorld() { console.log("Hello, World!"); } function helloPerson(name) { console.log(`Hello, ${name}!`); } function helloTeam() { console.log("Hello, Team!"); setTimeout(() => { helloPerson("Monty"); }, 0); } function byeWorld() { console.log("Bye, World!"); } helloWorld(); helloTeam(); byeWorld(); /* Outputs: Hello, World! Hello, Team! Bye, World! Hello, Monty! */
The only change we made here is to use setTimeout()
. However, the timeout has been set to zero. Therefore, we expect Hello, Monty! to be output before Bye, World!. If you understand how the event loop works, you'll understand why this doesn't happen.
When helloTeam()
is pushed onto the stack, the setTimeout()
method is encountered. However, the call to helloPerson()
in setTimeout()
will be put into the queue and will be executed once there are no synchronization tasks to be executed.
Once the call to byeWorld()
completes, the event loop checks the queue for any pending tasks and finds a call to helloPerson()
. At this point, it executes the function and logs Hello, Monty! to the console.
This indicates that the timeout duration you provide to setTimeout()
is not a guaranteed time for the callback to execute. This is the minimum time for the callback to be executed.
Keep our web pages responsive
An interesting feature of JavaScript is that it runs a function until it completes. This means that as long as the function is on the stack, the event loop cannot process any other tasks in the queue or execute other functions.
This may cause the web page to "hang" because it is unable to perform other operations, such as processing user input or making DOM-related changes. Consider the following example, where we find the number of prime numbers in a given range:
function isPrime(num) { if (num <= 1) { return false; } for (let i = 2; i <= Math.sqrt(num); i++) { if (num % i === 0) { return false; } } return true; } function listPrimesInRange(start, end) { const primes = []; for (let num = start; num <= end; num++) { if (isPrime(num)) { primes.push(num); } } return primes; }
In our listPrimesInRange()
function, we iterate over the numbers from start
to end
. For each number, we call the isPrime()
function to see if it is prime. isPrime()
The function itself has a for
loop that goes from 2
to Math.sqrt(num)
to determine if the number is Prime number.
查找给定范围内的所有素数可能需要一段时间,具体取决于您使用的值。当浏览器进行此计算时,它无法执行任何其他操作。这是因为 listPrimesInRange()
函数将保留在堆栈中,浏览器将无法执行队列中的任何其他任务。
现在,看一下以下函数:
function listPrimesInRangeResponsively(start) { let next = start + 100,000; if (next > end) { next = end; } for (let num = start; num <= next; num++) { if (isPrime(num)) { primeNumbers.push(num); } if (num == next) { percentage = ((num - begin) * 100) / (end - begin); percentage = Math.floor(percentage); progress.innerText = `Progress ${percentage}%`; if (num != end) { setTimeout(() => { listPrimesInRangeResponsively(next + 1); }); } } if (num == end) { percentage = ((num - begin) * 100) / (end - begin); percentage = Math.floor(percentage); progress.innerText = `Progress ${percentage}%`; heading.innerText = `${primeNumbers.length - 1} Primes Found!`; console.log(primeNumbers); return primeNumbers; } } }
这一次,我们的函数仅在批量处理范围时尝试查找素数。它通过遍历所有数字但一次仅处理其中的 100,000 个来实现这一点。之后,它使用 setTimeout()
触发对同一函数的下一次调用。
setTimeout()
被调用而没有指定延迟时,它会立即将回调函数添加到事件队列中。
下一个调用将被放入队列中,暂时清空堆栈以处理任何其他任务。之后,JavaScript 引擎开始在下一批 100,000 个数字中查找素数。
尝试单击此页面上的计算(卡住)按钮,您可能会收到一条消息,指出该网页正在减慢您的浏览器速度,并建议您停止该脚本。 p>
另一方面,单击计算(响应式)按钮仍将使网页保持响应式。
最终想法
在本教程中,我们了解了 JavaScript 中的事件循环以及它如何有效地执行同步和异步代码。事件循环使用队列来跟踪它必须执行的任务。
由于 JavaScript 不断执行函数直至完成,因此进行大量计算有时会“挂起”浏览器窗口。根据我们对事件循环的理解,我们可以重写我们的函数,以便它们批量进行计算。这允许浏览器保持窗口对用户的响应。它还使我们能够定期向用户更新我们在计算中取得的进展。
The above is the detailed content of Understanding the event loop in JavaScript. For more information, please follow other related articles on the PHP Chinese website!
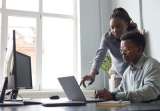
This tutorial demonstrates building a WordPress plugin using object-oriented programming (OOP) principles, leveraging the Dribbble API. Let's refine the text for clarity and conciseness while preserving the original meaning and structure. Object-Ori
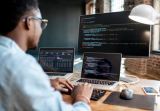
Best Practices for Passing PHP Data to JavaScript: A Comparison of wp_localize_script and wp_add_inline_script Storing data within static strings in your PHP files is a recommended practice. If this data is needed in your JavaScript code, incorporat
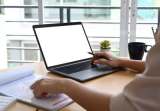
This guide demonstrates how to embed and protect PDF files within WordPress posts and pages using a WordPress PDF plugin. PDFs offer a user-friendly, universally accessible format for various content, from catalogs to presentations. This method ens

WordPress is easy for beginners to get started. 1. After logging into the background, the user interface is intuitive and the simple dashboard provides all the necessary function links. 2. Basic operations include creating and editing content. The WYSIWYG editor simplifies content creation. 3. Beginners can expand website functions through plug-ins and themes, and the learning curve exists but can be mastered through practice.

People choose to use WordPress because of its power and flexibility. 1) WordPress is an open source CMS with strong ease of use and scalability, suitable for various website needs. 2) It has rich themes and plugins, a huge ecosystem and strong community support. 3) The working principle of WordPress is based on themes, plug-ins and core functions, and uses PHP and MySQL to process data, and supports performance optimization.

The core version of WordPress is free, but other fees may be incurred during use. 1. Domain names and hosting services require payment. 2. Advanced themes and plug-ins may be charged. 3. Professional services and advanced features may be charged.

WordPress itself is free, but it costs extra to use: 1. WordPress.com offers a package ranging from free to paid, with prices ranging from a few dollars per month to dozens of dollars; 2. WordPress.org requires purchasing a domain name (10-20 US dollars per year) and hosting services (5-50 US dollars per month); 3. Most plug-ins and themes are free, and the paid price ranges from tens to hundreds of dollars; by choosing the right hosting service, using plug-ins and themes reasonably, and regularly maintaining and optimizing, the cost of WordPress can be effectively controlled and optimized.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Atom editor mac version download
The most popular open source editor
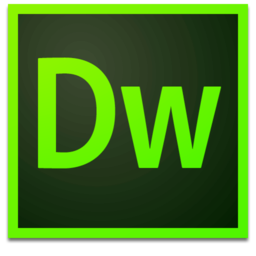
Dreamweaver Mac version
Visual web development tools
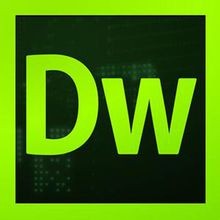
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
