As mentioned in the first article in this series, one of the main problems with custom database tables is that they are not handled by existing import and export handlers. This article aims to address this problem, but it should be noted that there is currently no fully satisfactory solution.
Let us consider two situations:
- Custom tables reference native WordPress tables
- Custom tables are completely independent from native tables
The "worst case scenario" is the first scenario. Take a custom table that saves user activity logs as an example. It references the user ID, object ID, and object type - all of which reference data stored in native WordPress tables. Now imagine that someone wants to import all the data from their WordPress website into a second website. For example, it's entirely possible that when importing a post, WordPress has to assign it a new ID because a post with that ID might already exist in the second site.
In this case, it is necessary to track such changes and update the IDs referenced in the table. This in itself is not that difficult. Unfortunately, the WordPress Importer plugin for handling importing data from other WordPress sites lacks the necessary hooks to achieve this. As suggested in this comment, a potential workaround would be to store the data in metadata as well. Unfortunately, this results in duplicate data and violates database normalization—generally not a good idea. In the end, it's only really feasible in a few use cases.
The second case avoids this complexity, but still requires custom import and export handlers. We will demonstrate this situation in the next two articles. However, to be consistent with the rest of this series, we will stick with the activity log table, even though it is an example of case (1).
Determine the format
First we need to decide the format of the exported file. The best format depends on the nature (or "structure") of the data and how it will be used. In my opinion, XML is generally better because it can handle one-to-many relationships. However, sometimes if the data is in tabular form, CSV may be preferable, especially because of its ease of integration with spreadsheet applications. In this example we will use XML.
Price increase
The next step is to create an admin page to allow users to export data from the log table. We will create a class that will add a page below the Tools menu item. The page only contains a button prompting the user to download the export file. The class will also add a handler to listen for form submissions and trigger file downloads.
First let us look at the structure of the class and then fill in the details of its methods.
class WPTuts_Log_Export_Admin_Page{ /** * The page hook suffix */ static $hook_suffix=''; static function load(){ add_action('admin_menu', array(__CLASS__,'add_submenu')); add_action('admin_init', array(__CLASS__,'maybe_download')); } static function add_submenu(){} static function maybe_download(){} static function display(){} } WPTuts_Log_Export_Admin_Page::load();
WPTuts_Log_Export_Admin_Page::load()
Initialize the class and hook callbacks to the appropriate operations:
-
add_submenu
– Method responsible for adding pages under the "Tools" menu. -
maybe_download
– This method will listen to check whether the download request has been submitted. This will also check permissions and nonce.
The export listener needs to be called early before any headers are sent, since we will be setting these headers ourselves. We could hook it to init
, but since we only allow export files to be downloaded in admin, admin_init
is more appropriate here.
Adding pages to your menu is easy. To add a page under Tools, we simply call add_management_page()
.
static function add_submenu(){ self::$hook_suffix = add_management_page( __('Export Logs','wptuts-log'), __('Export Logs','wptuts-log'), 'manage_options', 'wptuts-export', array(__CLASS__,'display') ); }
Here $hook_suffix
is a suffix used for various screen-specific hooks, discussed here. We don't use it here - but if you do, it's better to store its value in a variable rather than hardcoding it.
Above we set the method display()
as the callback of our page, then we define it:
static function display(){ echo '<div class="wrap">'; screen_icon(); echo '<h2 id="Export-Activity-Logs-wptuts-log">' . __( 'Export Activity Logs', 'wptuts-log' ) . '</h2>'; ?> <form id="wptuts-export-log-form" method="post" action=""> <p> <label><?php _e( 'Click to export the activity logs','wptuts-log' ); ?></label> <input type="hidden" name="action" value="export-logs" /> </p> <?php wp_nonce_field('wptuts-export-logs','_wplnonce') ;?> <?php submit_button( __('Download Activity Logs','wptuts-log'), 'button' ); ?> </form> <?php }
Finally, we hope to monitor when the above form is submitted and trigger the export file download.
static function maybe_download(){ /* Listen for form submission */ if( empty($_POST['action']) || 'export-logs' !== $_POST['action'] ) return; /* Check permissions and nonces */ if( !current_user_can('manage_options') ) wp_die(''); check_admin_referer( 'wptuts-export-logs','_wplnonce'); /* Trigger download */ wptuts_export_logs(); }
All that's left is to create the function wptuts_export_logs()
to create and return our .xml file.
Create export file
The first thing we want the function to do is retrieve the log. If any, we need to set the appropriate headers and print them in XML format. Since we want the user to download the XML file, we set the Content-Type to text/xml
and the Content-Description to File Transfer
. We will also generate a suitable name for the downloaded file. Finally, we'll add some comments - these are completely optional, but helpful in guiding the user on what to do with the downloaded file.
Since we created the API for the table in the previous part of this series, our export handler does not need to touch the database directly - nor does it need to clean up the $args
array, as this is done by Processed by wptuts_get_logs()
.
function wptuts_export_logs( $args = array() ) { /* Query logs */ $logs = wptuts_get_logs($args); /* If there are no logs - abort */ if( !$logs ) return false; /* Create a file name */ $sitename = sanitize_key( get_bloginfo( 'name' ) ); if ( ! empty($sitename) ) $sitename .= '.'; $filename = $sitename . 'wptuts-logs.' . date( 'Y-m-d' ) . '.xml'; /* Print header */ header( 'Content-Description: File Transfer' ); header( 'Content-Disposition: attachment; filename=' . $filename ); header( 'Content-Type: text/xml; charset=' . get_option( 'blog_charset' ), true ); /* Print comments */ echo "<!-- This is a export of the wptuts log table -->\n"; echo "<!-- (Demonstration purposes only) -->\n"; echo "<!-- (Optional) Included import steps here... -->\n"; /* Print the logs */ }
您会注意到,我们已将实际查询数组作为参数传递给 wptuts_export_logs()
函数。我们可以对此进行硬编码,但不这样做也是有道理的。虽然这里的目的只是导出表中的所有内容,但将查询作为参数传递允许我们稍后添加在特定时间范围内或针对特定用户导出日志的选项。 p>
创建 XML 文件时,我们需要确保标签之间打印的值不包含字符 &
、 或 <code>>
。为了确保这一点,对于 ID,我们使用 absint
清理数据,并使用 sanitize_key
清理对象类型和活动(因为我们希望这些仅包含小写字母数字、下划线和连字符)。
/* Print logs to file */ echo '<logs>'; foreach ( $logs as $log ) { ?> <item> <log_id><?php echo absint($log->log_id); ?></log_id> <activity_date><?php echo mysql2date( 'Y-m-d H:i:s', $log->activity_date, false ); ?></activity_date> <user_id><?php echo absint($log->user_id); ?></user_id> <object_id><?php echo absint($log->object_id); ?></object_id> <object_type><?php echo sanitize_key($log->object_type); ?></object_type> <activity><?php echo sanitize_key($log->activity); ?></activity> </item> <?php } echo '</logs>';
更一般地,您可以使用以下函数将要打印的值包装在 CDATA
标记内来清理它们:
/** * Wraps the passed string in a XML CDATA tag. * * @param string $string String to wrap in a XML CDATA tag. * @return string */ function wptuts_wrap_cdata( $string ) { if ( seems_utf8( $string ) == false ) $string = utf8_encode( $string ); return '<![CDATA[' . str_replace( ']]>', ']]]]><![CDATA[>', $string ) . ']]>'; }
最后我们 exit()
以防止任何进一步的处理:
/* Finished - now exit */ exit();
导航到我们的导出页面,单击“下载活动日志”应提示下载 XML 文件。
摘要
在本教程中,我们研究了从自定义表中导出数据。不幸的是,当数据引用本机 WordPress 表时,这充其量是有问题的。上述方法仅适用于数据无法做到这一点的情况。使用的示例(我们的活动日志)显然不属于此类,只是为了与本系列的其余部分保持一致而使用。
当数据确实引用本机表时,显然有必要将其与本机表一起导入,并在此过程中跟踪导入期间发生的 ID 任何更改。目前,现有的导入和导出处理程序无法实现这一点,因此唯一可行的选择是创建自己的处理程序。在自定义数据仅引用单个帖子类型的简单情况下,可以设计导入和导出处理程序来处理该帖子类型以及自定义数据,并通知用户不要使用该帖子类型的本机导出器。
在本系列的下一部分中,我们将为导出的 .xml 文件创建一个简单的导入处理程序。
The above is the detailed content of Data export: customized database table. For more information, please follow other related articles on the PHP Chinese website!
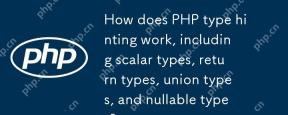
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
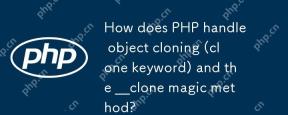
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
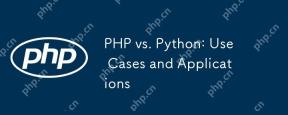
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.
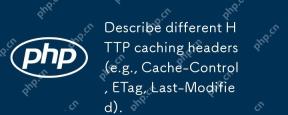
Key players in HTTP cache headers include Cache-Control, ETag, and Last-Modified. 1.Cache-Control is used to control caching policies. Example: Cache-Control:max-age=3600,public. 2. ETag verifies resource changes through unique identifiers, example: ETag: "686897696a7c876b7e". 3.Last-Modified indicates the resource's last modification time, example: Last-Modified:Wed,21Oct201507:28:00GMT.
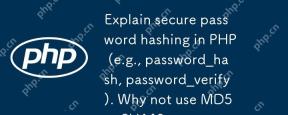
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
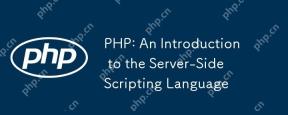
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
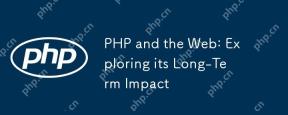
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
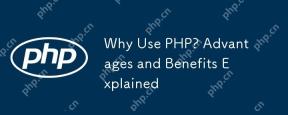
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
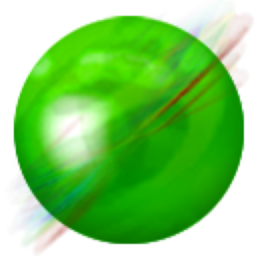
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
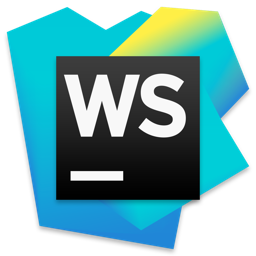
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version