


In the field of computer science, we have to deal with large data sets, which include query select and update operations. Performing these operations in real-time with low time complexity is a challenging task for developers.
Using Fenwick trees is an effective way to solve these range-based query problems.
Fenwick Tree is a data structure that can efficiently update elements and calculate the prefix sum of numbers in a table. It is also called a binary index tree.
In this article, we will discuss how to use Fenwick tree to find the number of elements greater than K in the range L to R.
Input and output scenarios
Suppose you have an array with N elements, and you want to find the number of elements in the array that are greater than K in the range L to R.
Input: arr = {1, 15, 12, 13, 6, 18, 14, 10, 23, 41, 16, 9} N = 11, K = 17, L = 1, R = 8 Output: 2
What is offline query?
Offline query is a query operation performed on a predetermined data set. In other words, these operations are performed only on those datasets that are not further modified while processing the query.
Use Fenwick Tree
Here we will use a Fenwick tree, which has vectors at each node containing all the elements of the array in order. We then use binary search to answer each query and count the number of elements.
Create two functions, updateTree() and total(), for updating and retrieving the prefix sum in BIT respectively.
Next, create another function that will count elements in the range L to R that are greater than "K". This function accepts the input values "arr", "N", "L", "R" and "K" ".
The count is calculated by subtracting the cumulative sum of K from the cumulative sum of the maximum range N.
To exclude out-of-range elements, subtract the cumulative sum of L-1 (if L is greater than 1).
Example
#include <iostream> #include <vector> using namespace std; // Updating the Fenwick Tree void updateTree(vector<int>& BIT, int index, int value) { while (index < BIT.size()) { BIT[index] += value; index += index & -index; } } int total(vector<int>& BIT, int index) { int result = 0; while (index > 0) { result += BIT[index]; index -= index & -index; } return result; } // Counting the number of elements int elementsGreaterThanK(vector<int>& arr, int N, int K, int L, int R) { vector<int> BIT(N+1, 0); for (int i = L; i <= R; i++) { updateTree(BIT, arr[i], 1); } int count = total(BIT, N) - total(BIT, K); if (L > 1) { count -= total(BIT, L-1); } return count; } int main() { vector<int> arr = {0, 5, 2, 3, 6, 8, 7, 1, 8, 4, 6, 9}; int N = 11; // Total range of values int K = 4; // Highest value int L = 1; // Start index of the range int R = 8; // End index of the range int count = elementsGreaterThanK(arr, N, K, L, R); cout << "Number of elements greater than " << K << " in the range [" << L << ", " << R << "]: " << count << endl; return 0; }
Output
Number of elements greater than 4 in the range [1, 8]: 5
alternative method
Here we will create a separate vector to store the query. Each query consists of two variables, index and val. index is used to store the position in the array, and val is used to store the value of the element at that index. This approach enables developers to perform a variety of query operations. Additionally, we use BIT to count the number of elements greater than K for each query.
Example
#include <iostream> #include <vector> using namespace std; struct Query { int index; int val; }; void updateTree(vector<int>& BIT, int index, int value) { while (index < BIT.size()) { BIT[index] += value; index += index & -index; } } int total(vector<int>& BIT, int index) { int result = 0; while (index > 0) { result += BIT[index]; index -= index & -index; } return result; } vector<int> elementsGreaterThanK(vector<int>& arr, vector<Query>& queries, int K) { int N = arr.size(); vector<int> BIT(N+1, 0); vector<int> result; for (int i = 0; i < N; i++) { updateTree(BIT, i+1, (arr[i] > K) ? 1 : 0); } for (auto query : queries) { if (query.val > K) { result.push_back(total(BIT, query.index)); } else { result.push_back(0); } } return result; } int main() { vector<int> arr = {3, 14, 32, 7, 11, 5, 8, 6, 16, 2, 15}; vector<Query> queries = {{2, 4}, {5, 3}, {3, 6}, {0, 3}}; int K = 2; vector<int> counts = elementsGreaterThanK(arr, queries, K); for (int count : counts) { cout << "Number of elements greater than " << K << ": " << count << endl; } return 0; }
Output
Number of elements greater than 2: 2 Number of elements greater than 2: 5 Number of elements greater than 2: 3 Number of elements greater than 2: 0
in conclusion
We can simply iterate the array from index L to R and increment by 1 every time the array element is greater than K in order to find the answer to each query. However, in order to reduce the time complexity, we use Fenwick tree to perform such query operations. We can also use Line Segment Tree and Sparse Table instead of Fenwick tree to perform such query operations.
The above is the detailed content of Using a tree array (offline query), calculate the number of elements greater than K in the range L to R. For more information, please follow other related articles on the PHP Chinese website!
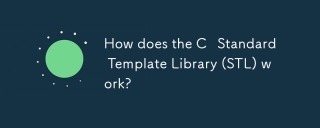
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
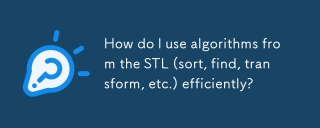
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
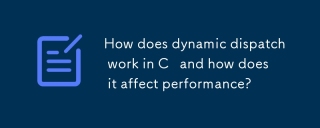
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
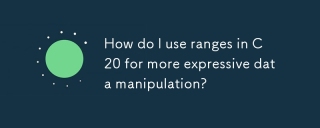
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
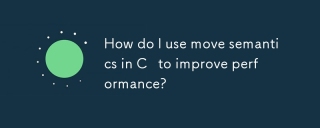
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
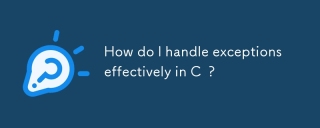
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
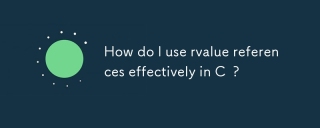
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
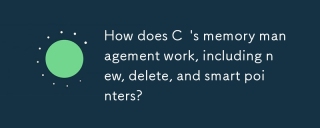
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
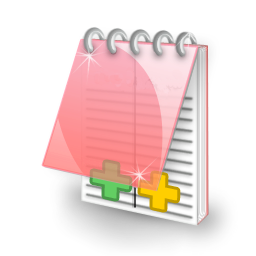
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 English version
Recommended: Win version, supports code prompts!
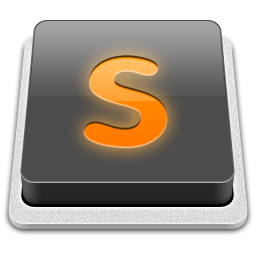
SublimeText3 Mac version
God-level code editing software (SublimeText3)
