Problem Statement
We are given a string 'str', containing uppercase or lowercase alphabetic characters. We need to check if the usage of uppercase characters in the string is correct.
Here's how to use uppercase letters correctly in strings.
If only the first character is uppercase, the other characters are lowercase.
If all characters of the string are lowercase.
If all characters of the string are uppercase.
Example
enter
"Hello"
Output
"valid"The Chinese translation of
Explanation
is:Explanation
In "Hello", only the first character is uppercase and the other characters are lowercase, so it is a valid string.
enter
'hello'
Output
'valid'The Chinese translation of
Explanation
is:Explanation
In the "hello" string, all characters are lowercase, so it is a valid string.
enter
‘heLLO’
Output
‘Not Valid’The Chinese translation of
Explanation
is:Explanation
In the string 'heLLO', the first character is lowercase, but the last 3 characters are uppercase, so this string is invalid.
method one
In this method, if the first character is a lowercase letter, we check if all the characters of the string are lowercase letters and return a boolean value. If the first character is an uppercase letter, we check if all other characters are uppercase or lowercase letters and return a boolean value.
algorithm
Step 1 - Define the isLower() function, which takes a single character as a parameter and returns a Boolean value whether the character is lowercase. If 'character-A' is greater than or equal to 32, the character is lowercase.
Step 2 - Define the isUpper() function just like the isLower() function and return a boolean value based on whether the character is uppercase or not.
Step 3 - Define the isValidUpper() function, which checks whether a string contains all valid uppercase characters.
Step 4 - In isValidUpper() function, use isLower() function and check if the first character is lowercase. If yes, use a loop and isUpper() function to check for all other characters. Returns false if any characters are uppercase. Otherwise, returns true if all characters are lowercase.
Step 5 - If the first character is a capital letter, you need to check two cases. The first case is that all characters can be uppercase, or all but the first character can be lowercase.
Step 5.1 - Define the variable 'totalUpper' and initialize it to 1.
Step 5.2 − Count the total number of uppercase characters in the string.
Step 5.3 - Return true if the total number of uppercase characters is equal to 1 or the length of the string, indicating that the string contains valid uppercase characters. Otherwise, returns false.
Example
#include <bits/stdc++.h> using namespace std; // Check if character c is in lowercase or not bool isLower(char c){ return c - 'A' >= 32; } // Check if character c is in uppercase or not bool isUpper(char c){ return c - 'A' < 32; } bool isValidUpperCase(string str){ int len = str.size(); // If the first character is in lowercase, check whether all the other characters are in lowercase or not. // If not, return false. Otherwise, return true. if (isLower(str[0])) { for (int i = 1; i < len; i++) { if (isUpper(str[i])) return false; } return true; } else { // If the first character is in uppercase, find the total number of uppercase characters int totalUpper = 1; for (int i = 1; i < len; i++){ if (isUpper(str[i])) totalUpper++; } // if the total number of uppercase characters is equal to the length of the string or 1, return true. Otherwise, return false. if (totalUpper == len || totalUpper == 1) return true; else return false; } } int main(){ string str1 = "TutorialsPoint"; string str2 = "tutorialspoint"; string str3 = "Tutorialspoint"; string str4 = "TUTORIALSPOINT"; cout << str1 << " : " << (isValidUpperCase(str1) ? "Valid" : "Not valid") << endl; cout << str2 << " : " << (isValidUpperCase(str2) ? "Valid" : "Not valid") << endl; cout << str3 << " : " << (isValidUpperCase(str3) ? "Valid" : "Not valid") << endl; cout << str4 << " : " << (isValidUpperCase(str4) ? "Valid" : "Not valid") << endl; return 0; }
Output
TutorialsPoint : Not valid tutorialspoint : Valid Tutorialspoint : Valid TUTORIALSPOINT : Valid
Time complexity − O(N), because it requires using a loop to traverse the string. The time complexity of isLower() and isUpper() functions is O(1).
Space Complexity − O(1) because it does not use any extra space.
Method 2
In the following method, we optimize the code of the first method. Here we check if the string contains valid uppercase characters by checking if two adjacent elements in the string except the first two characters have the same case.
algorithm
Step 1 − Use a for loop to iterate from the first index to the last index of the string.
Step 2 - In the for loop, if the current character is an uppercase letter and the previous character is a lowercase letter, return false because it is not a valid string.
Step 3 − If the current character is a lowercase letter and the previous character is an uppercase letter, follow the steps below.
Step 3.1 - Check if the previous character is at the 0th index or the first character in the string and continue in the for loop.
Step 3.2 − If the previous character is not the first character, return false.
Example
#include <bits/stdc++.h> using namespace std; bool isValidUpperCase(string str){ for (int i = 1; i < str.length(); i++){ // If str[i] is in lower case and str[i-1] is in upper case, handle the case if (str[i] - 'A' >= 32 && str[i - 1] - 'A' < 32) { // If the str[i-1] is the first character, continue the loop. Otherwise, return false. if (i - 1 == 0) continue; return false; } // If str[i] is in upper case and str[i-1] is in lower case, return false. else if (str[i] - 'A' < 32 && str[i - 1] - 'A' >= 32) { return false; } } // Return true return true; } int main(){ string str1 = "TutorialsPoint"; string str2 = "tutorialspoint"; string str3 = "Tutorialspoint"; string str4 = "TUTORIALSPOINT"; cout << str1 << " : " << (isValidUpperCase(str1) ? "Valid" : "Not valid") << endl; cout << str2 << " : " << (isValidUpperCase(str2) ? "Valid" : "Not valid") << endl; cout << str3 << " : " << (isValidUpperCase(str3) ? "Valid" : "Not valid") << endl; cout << str4 << " : " << (isValidUpperCase(str4) ? "Valid" : "Not valid") << endl; return 0; }
Output
TutorialsPoint : Not valid tutorialspoint : Valid Tutorialspoint : Valid TUTORIALSPOINT : Valid
Time complexity - O(N) because it requires using a loop to traverse the string.
Space Complexity − O(1) because it does not use any extra space.
in conclusion
In this tutorial, the user learned to check whether a string contains valid uppercase characters. We learned two different methods. In the first approach, we break the problem into three parts, and in the second approach, we check the character case of adjacent elements. However, the time and space complexity of both codes are similar, but the code of the second method is more readable.
The above is the detailed content of Check if uppercase characters in a string are used correctly. For more information, please follow other related articles on the PHP Chinese website!
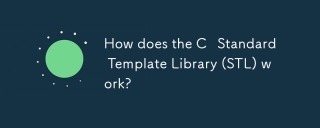
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
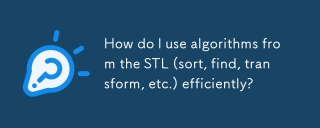
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
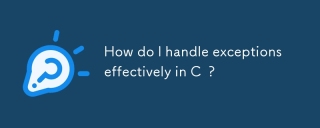
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
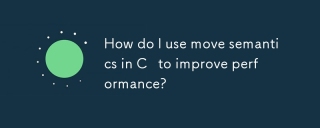
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
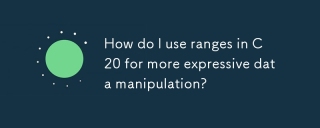
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
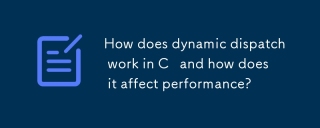
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
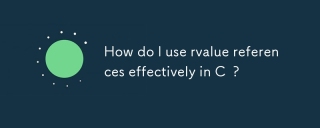
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
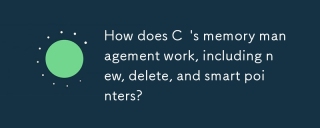
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
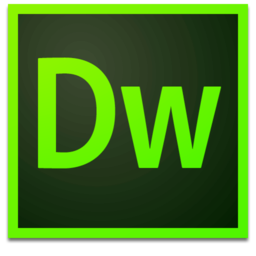
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),