The character that appears most often in the linked list
We are given a singly linked list of characters, and our task is to print the character that appears most often in the linked list. If multiple characters occur the same number of times, the last occurrence of the character is printed.
Singly linked list is a linear data structure composed of nodes. Each node contains data and a pointer to the next node, which contains the memory address of the next node because the memory allocated to each node is not contiguous.
Example
Assume we have been given a list of character links
Example 1
Input: LL = a -> b -> c -> c -> c
Output: The most common character is c.
Explanation: In the given linked list LL, a appears once, b appears once, and c appears 3 times. Therefore, the output is c.
Example 2
enter:
LL = x -> x -> y -> y -> z -> z
Output: The largest occurring character is z.
Explanation: In the given linked list LL, x appears 2 times, y appears 2 times, and z appears 2 times. All occurrences are the same because z appears last, so the output is z.
Here we will discuss two methods. Let’s look at the following parts -
Method 1: Iterative calculation frequency
The idea of this method is that we will traverse the linked list and calculate the frequency of each character, then find the character with the highest frequency, and if multiple characters have the same frequency, print the character and return the last character.
Example
#include <iostream> using namespace std; // creating a class to have a structure for linked list nodes class Node{ public: char data; // variable to store the characters Node* next = NULL; // variable to store the address of the next node Node(char cur){ data = cur; } }; // function to print the elements of the linked list void printLL(Node* head){ // creating a temporary pointer Node* temp = head; while(temp != nullptr){ cout<<temp->data<<" -> "; temp = temp->next; } cout<<"NULL"<<endl; } // function to find the max frequency void maxFreq(Node* head){ // traversing over the linked list for each character // starting from the first character to the last character int ans = 0; // variable to store the maximum frequency char char_ans; Node* temp_first = head; // variable to store the current first node while(temp_first != nullptr){ int cur = 0; // variable to store the frequency of the current character Node* temp = temp_first; while(temp != nullptr){ if(temp->data == temp_first->data){ cur++; } temp = temp->next; } if(ans < cur){ ans = cur; char_ans = temp_first->data; } temp_first = temp_first->next; } cout<<"The last character in the given linked list is '"<<char_ans<<"' with the frequency of "<< ans<<endl; } // main function int main(){ // defining the linked list Node* head = new Node('a'); head->next = new Node('b'); head->next->next = new Node('b'); head->next->next->next = new Node('c'); head->next->next->next->next = new Node('d'); head->next->next->next->next->next = new Node('d'); head->next->next->next->next->next->next = new Node('d'); cout<<"The given linked list is: "<<endl; printLL(head); maxFreq(head); return 0; }
Output
The given linked list is: a -> b -> b -> c -> d -> d -> d -> NULL The last character in the given linked list is 'd' with the frequency of 3
Time complexity
: O(N*N), where N is the size of the linked list.
Space complexity: O(1)
Method 2: Use counting array
The idea of this approach is that we will maintain an array of counts where we store the frequency of each character and then iterate through the array and find the highest frequency character. If multiple characters have the same frequency, print that character and then return the last character.
Example
#include <iostream> using namespace std; // creating a class to have a structure for linked list nodes class Node{ public: char data; // variable to store the characters Node* next = NULL; // variable to store the address of the next node Node(char cur){ data = cur; } }; // function to print the elements of the linked list void printLL(Node* head){ // creating a temporary pointer Node* temp = head; while(temp != nullptr){ cout<<temp->data<<" -> "; temp = temp->next; } cout<<"NULL"<<endl; } // function to find the max frequency void maxFreq(Node* head){ int ans = 0; // variable to store the maximum frequency char char_ans; // traversing over the linked list for each lowercase character for(char i = 'a'; i<= 'z'; i++){ Node* temp = head; int cur = 0; // variable to store the frequency of the current character while(temp != nullptr){ if(temp->data == i){ cur++; } temp = temp->next; } if(ans <= cur){ ans = cur; char_ans = i; } } cout<<"The last character in the given linked list is '"<<char_ans<<"' with the frequency of "<< ans<<endl; } int main(){ // defining the linked list Node* head = new Node('a'); head->next = new Node('b'); head->next->next = new Node('b'); head->next->next->next = new Node('c'); head->next->next->next->next = new Node('e'); head->next->next->next->next->next = new Node('d'); head->next->next->next->next->next->next = new Node('d'); cout<<"The given linked list is: "<<endl; printLL(head); maxFreq(head); return 0; }
Output
The given linked list is: a -> b -> b -> c -> e -> d -> d -> NULL The last character in the given linked list is 'd' with the frequency of 2
Time complexity
O(N), where N is the size of the linked list.
Space complexity: O(N), where N is the size of the linked list.
in conclusion
Here we discuss how to find the characters that appear most in the linked list. To find the maximum occurrence of characters, we discussed two methods. The first method uses a while loop for each character of the given linked list and the second method uses a for loop for each lower case character and maintains the count.
The above is the detailed content of The character that appears most often in the linked list. For more information, please follow other related articles on the PHP Chinese website!
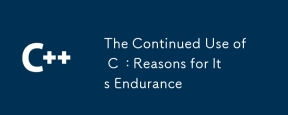
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
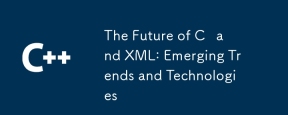
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
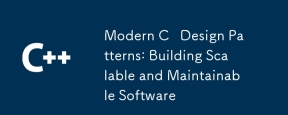
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
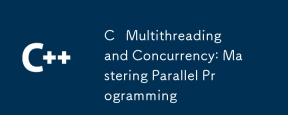
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
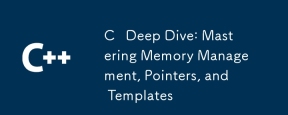
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
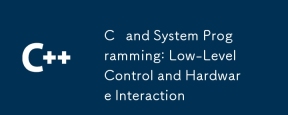
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
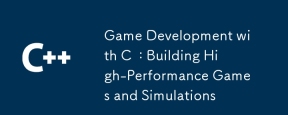
C is suitable for building high-performance gaming and simulation systems because it provides close to hardware control and efficient performance. 1) Memory management: Manual control reduces fragmentation and improves performance. 2) Compilation-time optimization: Inline functions and loop expansion improve running speed. 3) Low-level operations: Direct access to hardware, optimize graphics and physical computing.
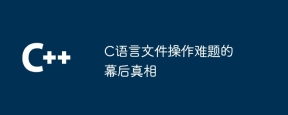
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Zend Studio 13.0.1
Powerful PHP integrated development environment
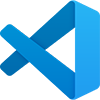
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
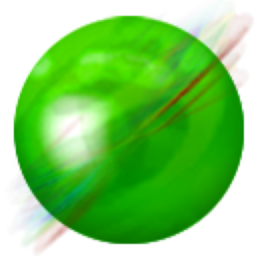
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment