Regression analysis and best fit straight line using Python
In this tutorial, we will use Python programming to implement regression analysis and best-fit lines
Introduction
Regression analysis is the most basic form of predictive analysis.
In statistics, linear regression is a method of modeling the relationship between a scalar value and one or more explanatory variables.
In machine learning, linear regression is a supervised algorithm. This algorithm predicts a target value based on independent variables.
More information about linear regression and regression analysis
In linear regression/analysis, the target is a real or continuous value such as salary, BMI, etc. It is often used to predict the relationship between a dependent variable and a set of independent variables. These models typically fit linear equations, however, there are other types of regression, including higher-order polynomials.
Before fitting a linear model to the data, it is necessary to check whether there is a linear relationship between the data points. This is evident from their scatter plot. The goal of the algorithm/model is to find the line of best fit.
In this article, we will explore linear regression analysis and its implementation using C.
The form of the linear regression equation is Y = c mx, where Y is the target variable and X is the independent variable or explanatory parameter/variable. m is the slope of the regression line and c is the intercept. Since this is a 2D regression task, the model tries to find the line of best fit during training. All points don't have to line up exactly on the same line. Some data points may lie on the line, and some may be scattered across the line. The vertical distance between the line and the data points is the residual. The value can be negative or positive depending on whether the point is below or above the line. The residual is a measure of how well the line fits the data. The algorithm is continuous to minimize the total residual.
The residual for each observation is the difference between the predicted value of y (the dependent variable) and the observed value of y
$$\mathrm{residual\: =\:actual\:y\:value\:−\:forecast\:y\:value}$$
$$\mathrm{ri\:=\:yi\:−\:y'i}$$
The most common metric for evaluating the performance of a linear regression model is called the root mean square error, or RMSE. The basic idea is to measure how bad/wrong the model's predictions are compared to actual observations.
Therefore, high RMSE is "bad" and low RMSE is "good"
RMSE error is
$$\mathrm{RMSE\:=\:\sqrt{\frac{\sum_i^n=1\:(this\:-\:this')^2}{n}}}$$ p>
RMSE is the root of the mean square of all residuals.
Using Python to implement
Example
# Import the libraries import numpy as np import math import matplotlib.pyplot as plt from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error # Generate random data with numpy, and plot it with matplotlib: ranstate = np.random.RandomState(1) x = 10 * ranstate.rand(100) y = 2 * x - 5 + ranstate.randn(100) plt.scatter(x, y); plt.show() # Creating a linear regression model based on the positioning of the data and Intercepting, and predicting a Best Fit: lr_model = LinearRegression(fit_intercept=True) lr_model.fit(x[:70, np.newaxis], y[:70]) y_fit = lr_model.predict(x[70:, np.newaxis]) mse = mean_squared_error(y[70:], y_fit) rmse = math.sqrt(mse) print("Mean Square Error : ",mse) print("Root Mean Square Error : ",rmse) # Plot the estimated linear regression line using matplotlib: plt.scatter(x, y) plt.plot(x[70:], y_fit); plt.show()
Output
Mean Square Error : 1.0859922470998231 Root Mean Square Error : 1.0421095178050257
in conclusion
Regression analysis is a very simple yet powerful technique used for predictive analysis in machine learning and statistics. The idea lies in its simplicity and the underlying linear relationship between the independent and target variables.
The above is the detailed content of Regression analysis and best fit straight line using Python. For more information, please follow other related articles on the PHP Chinese website!
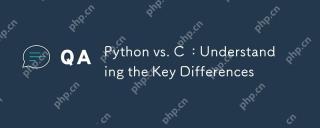
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
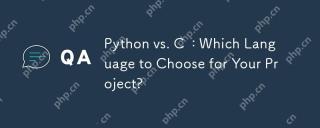
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
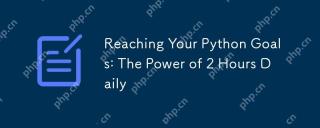
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
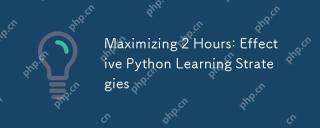
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
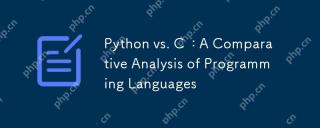
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
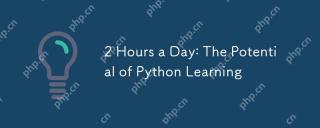
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
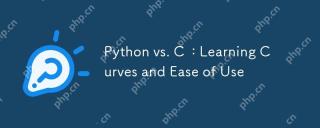
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
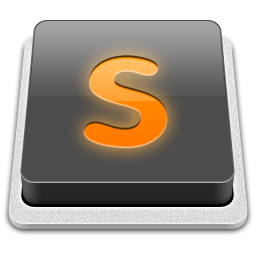
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor