


How to use C to build safe and reliable embedded system power management functions
Power management of embedded systems is an important task, which can extend the battery life of the system , to ensure the stability and reliability of the system. In this article, we explore how to use C to build a safe and reliable power management function for embedded systems and provide code examples.
- System architecture design
Before building the embedded system power management function, the system architecture design needs to be carried out first. This includes defining the individual components and modules of the system, as well as how they relate to and communicate with each other. The following is a simple system architecture diagram:
+-----------------+ | | | Power Manager | | | +-----------------+ | +-----------------+ | | | Power Supply | | | +-----------------+
In this example, there is a Power Manager module responsible for controlling the power management of the system. It communicates with the Power Supply module to monitor and regulate the power supply.
- Design of C classes
In C, we can use classes to represent various components and modules of the system. Below is an example of a Power Manager class:
class PowerManager { public: PowerManager() { // 初始化变量和其他必要的操作 } void monitorPowerSupply() { // 监测电源供应的电压和电流 } void adjustPowerConsumption() { // 调节功耗,例如降低系统的亮度或关闭一些无关的模块 } void handlePowerFailure() { // 处理电源故障,例如保存数据并进入休眠模式 } private: // 私有变量,用于保存相关的数据和状态信息 };
In this example, the PowerManager class has some public functions to perform different power management tasks. It also has some private variables for saving related data and status information.
- Implementing code logic
After the design of the C class is completed, we can start to implement the specific code logic. Here is some sample code:
#include <iostream> #include <thread> class PowerManager { public: PowerManager() { // 初始化变量和其他必要的操作 } void monitorPowerSupply() { std::thread t([this]() { while (true) { // 监测电源供应的电压和电流 if (powerSupplyVoltage <= minVoltage) { handlePowerFailure(); } std::this_thread::sleep_for(std::chrono::seconds(1)); } }); t.detach(); } void adjustPowerConsumption() { // 调节功耗,例如降低系统的亮度或关闭一些无关的模块 } void handlePowerFailure() { // 处理电源故障,例如保存数据并进入休眠模式 } private: float powerSupplyVoltage; // 电源供应的电压 const float minVoltage = 3.0; // 最低电压阈值 }; int main() { PowerManager powerManager; powerManager.monitorPowerSupply(); while (true) { // 执行其他任务 powerManager.adjustPowerConsumption(); } return 0; }
In this example, we use the multi-threading capabilities of C++11 to monitor the voltage and current of the power supply. If the power supply voltage is below the minimum threshold, the handlePowerFailure() function is called.
- Functional testing and debugging
After completing the code implementation, functional testing and debugging need to be performed to ensure that the power management function of the system works normally. During testing, simulated power supplies and other related equipment can be used to simulate the actual operating environment.
- Performance Optimization and Code Maintenance
After the system runs stably and passes the functional test, performance optimization and code maintenance can be performed. Based on actual needs, the code can be optimized to improve the system's response speed and power consumption efficiency. At the same time, the code also needs to be maintained to ensure the stability and reliability of the system in long-term operation.
Summary
This article introduces how to use C to build safe and reliable embedded system power management functions. Through reasonable system architecture design and the use of C classes to represent various components and modules of the system, we can easily implement a powerful power management system. At the same time, we provide some code examples to help readers better understand and apply these concepts. I hope this article helps you build power management functions in embedded systems!
The above is the detailed content of How to use C++ to build safe and reliable embedded system power management functions. For more information, please follow other related articles on the PHP Chinese website!
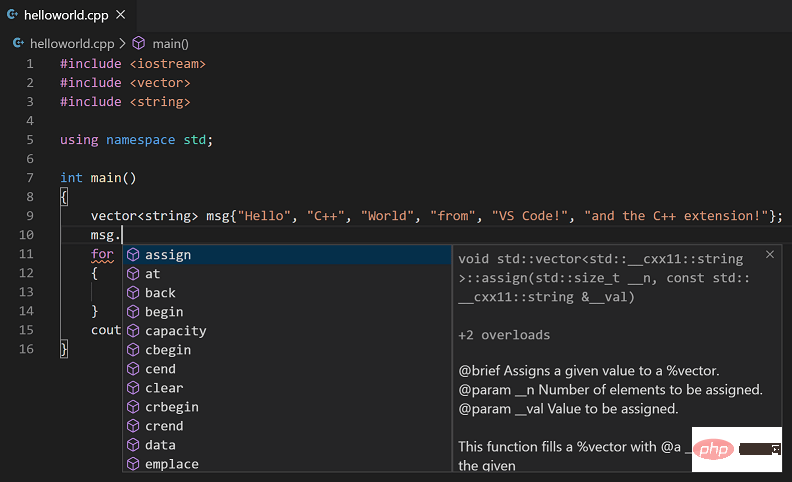
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
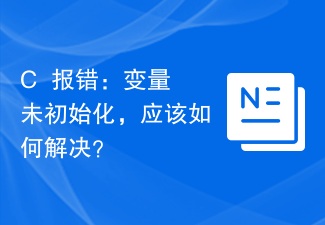
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
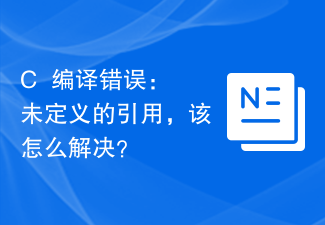
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
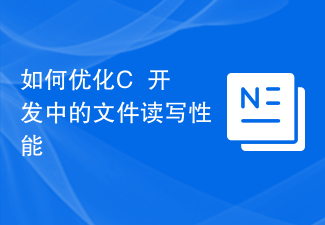
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
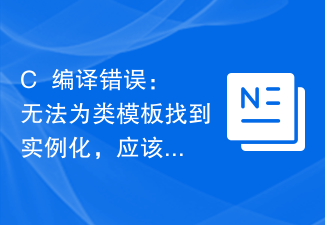
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
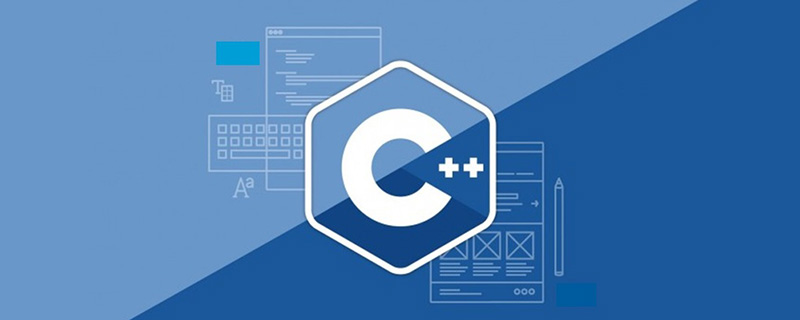
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
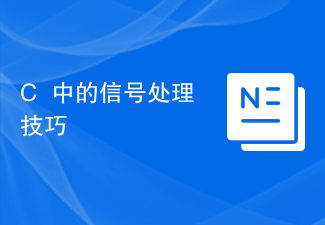
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以
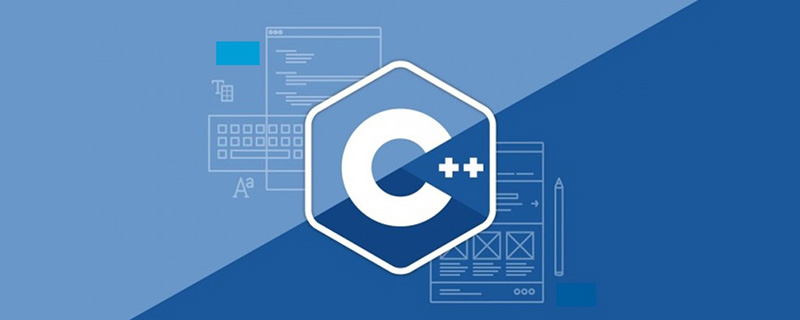
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
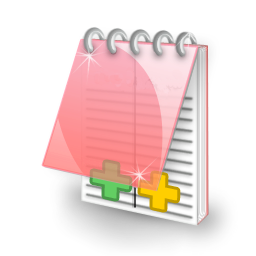
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
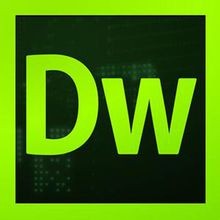
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
