The "final" keyword in Java can be used to define constant values and prevent variables, methods, or classes from being changed or overwritten. Immutability, on the other hand, describes the characteristic of an object remaining in a constant state throughout its existence. Once an object is formed, its value does not change.
Variables, methods, and classes are restricted by the "final" keyword, but immutability goes a step further and ensures that the entire state of the object is preserved.
Let us understand the main differences between final and immutable in this article.
Java Final Version
The final keyword in Java has several characteristics:
Final variable: Its initial value cannot be modified after initialization. They are often used to declare unchangeable or immutable values.
Final methods: They cannot be modified by subclasses, ensuring that they behave consistently. They help maintain the effectiveness of important procedures.
Final classes: They cannot be extended by other classes and their implementation is guaranteed not to be changed. Final classes are often used to build security or utility classes.
Initialization: To ensure that the final variable has a known value, it must be assigned a value during declaration or in the constructor.
Performance: The use of final allows the compiler to optimize the code more successfully, potentially improving performance.
Security: Final improves the security of Java programs by preventing unauthorized changes to sensitive data or behavior.
Immutability in Java
In Java, an immutable class refers to a class whose contents cannot be changed once created. To create an immutable class, follow these requirements:
Declare the class as Final to prevent inheritance.
Declare the data members of the class as private to restrict direct access.
Declare data members as final to prevent modification after the object is created.
Use parameterized constructors to initialize all fields through deep copy to prevent modification through object references.
Return a copy (deep copy) of the object in the getter method instead of the actual object reference to maintain immutability.
By following these properties, you can create your own immutable classes in Java, similar to the built-in immutable classes like Integer, Boolean, Byte, Short and String.
Difference between final and immutable
When it comes to Java programming, it is crucial to understand the difference between "final" and "immutable".
-
Final: Keep object references and allow state mutations
Let's start with "Final". When an object or variable is marked final in Java, it means that the reference cannot be changed to point to another object or variable after giving it a value. It's important to remember that although the reference is fixed, using the associated setter method still allows you to change the object's state. So even though the reference itself cannot be changed, you can still use accessible methods to change the object's internal properties or properties. In other words, final ensures the stability of an object reference while allowing changes to its internal state.
-
Immutability: Immutable Values and Reference Flexibility
Now let’s turn our attention to “immutability”. In Java, immutability means that the actual value of an object cannot be changed after it is created. However, unlike Final, you can modify the reference itself and assign it to another object or variable. This means that while the value of the object remains the same, you can change its reference to point to a different instance.
-
Application and Scope: Final and Immutability
The modifier "final" applies to variables in Java and not to objects. It emphasizes restrictions on changing references or variables while allowing modification of the object's state. Immutability, on the other hand, applies to objects, indicating that their values cannot be changed once created. It is important to understand the difference between these two concepts to ensure that Java programs behave as expected.
-
Meaning: Object address and state variability
When we declare an object or variable as final, we force the permanence of its address. In other words, the reference remains fixed, preventing any changes to the location it points to. In contrast, immutability emphasizes that once an object is created, its state cannot be modified. This means that the object's internal values cannot be changed, thus maintaining its integrity and consistency throughout program execution.
StringerBuffer()
This code demonstrates the difference between the "final" keyword and immutability in Java. The "final" keyword makes a variable constant and prevents reassignment, while immutability means that the object itself cannot be modified.
algorithm
Step 1: Declare variable "sb" as the final StringBuffer object with an initial value of "Hello".
Step 2: Use the append() method to append "TP" to the StringBuffer object referenced by "sb".
Step 3: Print the updated value of "sb", which is "HelloTP".
Step 4: Attempting to reassign the new StringBuffer object to the variable "sb" will result in a compile-time error.
Step 5: Print the value of "sb", but due to the error in the previous step, this line will not be executed.
Example
// Java program to illustrate difference between final and immutability public class Tutorialspoint { public static void main(String[] args) { final StringBuffer sb = new StringBuffer("Hello"); // We can make changes even though reference variable sb is final sb.append("TP"); System.out.println(sb); // Compile time error will appear here. This is because the final variable cannot be reassigned sb = new StringBuffer("Hello World"); System.out.println(sb); } }
Output
Tutorialspoint.java:16: error: cannot assign a value to final variable sb sb = new StringBuffer("Hello World"); ^ 1 error
in conclusion
In summary, "finality" and immutability have very different characteristics in Java. The "final" keyword restricts the reallocation of object references but allows modification of the object's state.
In contrast, immutability prevents the value of an object from being changed, but allows references to be reassigned. Understanding the application and scope of "final" and immutability is important for designing reliable Java programs. When an object or variable is declared final, its address remains fixed, while immutability ensures that the object's internal values cannot be modified. The sample code demonstrates the difference, where "final" prevents reallocation, causing a compile-time error.
The above is the detailed content of Final vs Immutability Comparison in Java. For more information, please follow other related articles on the PHP Chinese website!
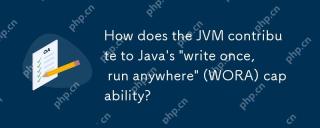
JVM implements the WORA features of Java through bytecode interpretation, platform-independent APIs and dynamic class loading: 1. Bytecode is interpreted as machine code to ensure cross-platform operation; 2. Standard API abstract operating system differences; 3. Classes are loaded dynamically at runtime to ensure consistency.
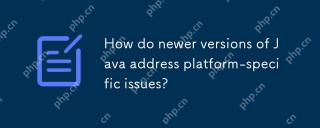
The latest version of Java effectively solves platform-specific problems through JVM optimization, standard library improvements and third-party library support. 1) JVM optimization, such as Java11's ZGC improves garbage collection performance. 2) Standard library improvements, such as Java9's module system reducing platform-related problems. 3) Third-party libraries provide platform-optimized versions, such as OpenCV.
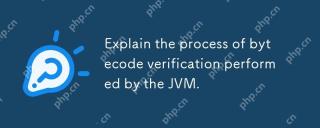
The JVM's bytecode verification process includes four key steps: 1) Check whether the class file format complies with the specifications, 2) Verify the validity and correctness of the bytecode instructions, 3) Perform data flow analysis to ensure type safety, and 4) Balancing the thoroughness and performance of verification. Through these steps, the JVM ensures that only secure, correct bytecode is executed, thereby protecting the integrity and security of the program.
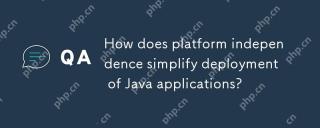
Java'splatformindependenceallowsapplicationstorunonanyoperatingsystemwithaJVM.1)Singlecodebase:writeandcompileonceforallplatforms.2)Easyupdates:updatebytecodeforsimultaneousdeployment.3)Testingefficiency:testononeplatformforuniversalbehavior.4)Scalab
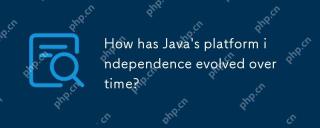
Java's platform independence is continuously enhanced through technologies such as JVM, JIT compilation, standardization, generics, lambda expressions and ProjectPanama. Since the 1990s, Java has evolved from basic JVM to high-performance modern JVM, ensuring consistency and efficiency of code across different platforms.
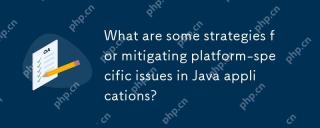
How does Java alleviate platform-specific problems? Java implements platform-independent through JVM and standard libraries. 1) Use bytecode and JVM to abstract the operating system differences; 2) The standard library provides cross-platform APIs, such as Paths class processing file paths, and Charset class processing character encoding; 3) Use configuration files and multi-platform testing in actual projects for optimization and debugging.
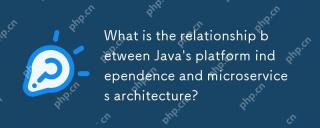
Java'splatformindependenceenhancesmicroservicesarchitecturebyofferingdeploymentflexibility,consistency,scalability,andportability.1)DeploymentflexibilityallowsmicroservicestorunonanyplatformwithaJVM.2)Consistencyacrossservicessimplifiesdevelopmentand
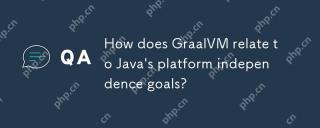
GraalVM enhances Java's platform independence in three ways: 1. Cross-language interoperability, allowing Java to seamlessly interoperate with other languages; 2. Independent runtime environment, compile Java programs into local executable files through GraalVMNativeImage; 3. Performance optimization, Graal compiler generates efficient machine code to improve the performance and consistency of Java programs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
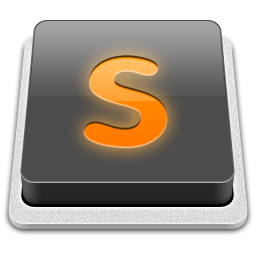
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor
