How to solve the '[Vue warn]: Invalid handler for event' error
How to solve the "[Vue warn]: Invalid handler for event" error
When developing applications using Vue.js, you may encounter error messages : "[Vue warn]: Invalid handler for event". This error usually occurs when we use an invalid event handler in the component. This article describes some ways to resolve this error and provides corresponding code examples.
- Check event handlers for naming errors
Event handlers in Vue.js should be bound to a component's method or computed property. When we bind event handlers in templates, we need to use the correct method name. If the event handler has the wrong name or does not exist, Vue.js will not be able to find the corresponding handler. In this case, you may receive "[Vue warn]: Invalid handler for event" error. Therefore, make sure that the methods you use actually exist and are properly bound to the event.
Example:
<template> <div> <button @click="handleClick">点击我</button> </div> </template> <script> export default { methods: { handleClick() { // 处理点击事件的逻辑 } } } </script>
In the above example, we used the correct method name handleClick
to bind to the click
event. If we use a non-existent or wrong method name, we will encounter the "[Vue warn]: Invalid handler for event" error.
- Check for syntax errors in event handlers
When we define methods or computed properties in components, make sure their syntax is correct. If the syntax of a method or computed property is wrong, Vue.js will not recognize them and throw a "[Vue warn]: Invalid handler for event" error.
Example:
<template> <div> <button @click="handleClick()">点击我</button> </div> </template> <script> export default { methods: { handleClick() { // 处理点击事件的逻辑 } } } </script>
In the above example, we added a pair of brackets ()
to the @click
event handler in the template . This is a common syntax error and is not allowed in Vue.js. The correct way to write it is to bind the reference to the method, not to call the method.
- Check the context of event binding
In Vue.js, each component instance has its own scope. When we bind an event handler in a template, Vue.js automatically binds the event handler to the context of the component instance. However, in some cases, Vue.js cannot correctly bind event handlers to component instances due to scoping issues.
Example:
<template> <div> <button @click="handleClick">点击我</button> </div> </template> <script> export default { mounted() { // 切换上下文 setTimeout(function() { this.handleClick(); }, 1000); }, methods: { handleClick() { // 处理点击事件的逻辑 } } } </script>
In the above example, we used the setTimeout
function in the mounted
hook function of the component and tried to call it in it handleClick
method. Since the setTimeout
function switches context, this
will no longer point to the component instance. Therefore, we need to use arrow functions to ensure that this
points to the correct context.
The solution is to use arrow functions to ensure the correctness of this
.
mounted() { setTimeout(() => { this.handleClick(); }, 1000); }
With the above three methods, you should be able to solve the "[Vue warn]: Invalid handler for event" error. Make sure the event handler has the correct name, correct syntax, and correct context. This way, you can continue to have fun developing applications with Vue.js.
The above is the detailed content of How to solve the '[Vue warn]: Invalid handler for event' error. For more information, please follow other related articles on the PHP Chinese website!

Vue.js and React each have their own advantages in scalability and maintainability. 1) Vue.js is easy to use and is suitable for small projects. The Composition API improves the maintainability of large projects. 2) React is suitable for large and complex projects, with Hooks and virtual DOM improving performance and maintainability, but the learning curve is steeper.

The future trends and forecasts of Vue.js and React are: 1) Vue.js will be widely used in enterprise-level applications and have made breakthroughs in server-side rendering and static site generation; 2) React will innovate in server components and data acquisition, and further optimize the concurrency model.

Netflix's front-end technology stack is mainly based on React and Redux. 1.React is used to build high-performance single-page applications, and improves code reusability and maintenance through component development. 2. Redux is used for state management to ensure that state changes are predictable and traceable. 3. The toolchain includes Webpack, Babel, Jest and Enzyme to ensure code quality and performance. 4. Performance optimization is achieved through code segmentation, lazy loading and server-side rendering to improve user experience.

Vue.js is a progressive framework suitable for building highly interactive user interfaces. Its core functions include responsive systems, component development and routing management. 1) The responsive system realizes data monitoring through Object.defineProperty or Proxy, and automatically updates the interface. 2) Component development allows the interface to be split into reusable modules. 3) VueRouter supports single-page applications to improve user experience.

The main disadvantages of Vue.js include: 1. The ecosystem is relatively new, and third-party libraries and tools are not as rich as other frameworks; 2. The learning curve becomes steep in complex functions; 3. Community support and resources are not as extensive as React and Angular; 4. Performance problems may be encountered in large applications; 5. Version upgrades and compatibility challenges are greater.

Netflix uses React as its front-end framework. 1.React's component development and virtual DOM mechanism improve performance and development efficiency. 2. Use Webpack and Babel to optimize code construction and deployment. 3. Use code segmentation, server-side rendering and caching strategies for performance optimization.

Reasons for Vue.js' popularity include simplicity and easy learning, flexibility and high performance. 1) Its progressive framework design is suitable for beginners to learn step by step. 2) Component-based development improves code maintainability and team collaboration efficiency. 3) Responsive systems and virtual DOM improve rendering performance.

Vue.js is easier to use and has a smooth learning curve, which is suitable for beginners; React has a steeper learning curve, but has strong flexibility, which is suitable for experienced developers. 1.Vue.js is easy to get started with through simple data binding and progressive design. 2.React requires understanding of virtual DOM and JSX, but provides higher flexibility and performance advantages.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
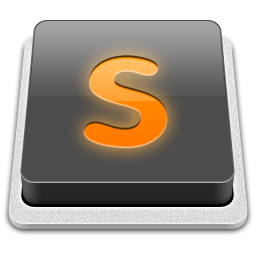
SublimeText3 Mac version
God-level code editing software (SublimeText3)
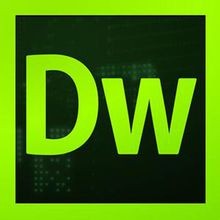
Dreamweaver CS6
Visual web development tools
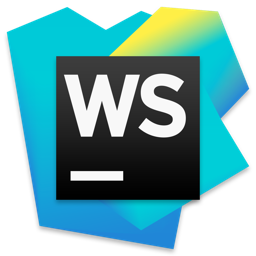
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
