PHP learning experience: How to handle errors
PHP Learning Experience: How to Handle Errors
When developing PHP applications, handling errors is a very important aspect. Good error handling can improve the stability and reliability of the code, and can also better help us debug the code and solve problems. This article will introduce some common error types and how to handle them, with corresponding code examples.
- Grammar errors
Grammar errors are the most common and easiest to find errors in the code writing process. It usually causes the PHP parser to not understand the code correctly, resulting in a parse error (Parse Error). This kind of error will usually be displayed in the editor or IDE, and the error message will indicate the specific error location.
For example, the following code is missing a closing bracket:
<?php function sum($a, $b { return $a + $b; } ?>
When we try to run this code, we get the following error message:
Parse error: syntax error, unexpected '{' in path/to/file.php on line 2
To solve this The error is as simple as double checking the code and fixing the bad syntax.
- Run-time errors
Run-time errors refer to errors that occur during code execution, and they may cause the program to fail to run properly. Methods of handling this error include using conditional statements and exception handling mechanisms.
The use of conditional statements is one of the simplest error handling methods. We can use if statements to check whether certain conditions are met, and then perform different operations based on the conditions.
For example, in the following code, we will check whether the divisor is zero to avoid dividing by zero:
<?php function divide($a, $b) { if($b == 0){ echo "除数不能为零"; }else{ return $a / $b; } } echo divide(10, 0); ?>
When we try to run this code, we will get the following error message:
除数不能为零
In addition to conditional statements, PHP also provides an exception handling mechanism to handle runtime errors. We can use the try-catch statement to catch and handle exceptions.
In the following code, we will try to open a file that does not exist. If an exception occurs, the corresponding error message will be captured and displayed:
<?php try { $file = fopen("nonexistentfile.txt", "r"); } catch (Exception $e) { echo "发生错误:" . $e->getMessage(); } ?>
When we try to run this code, You will get the following error message:
发生错误:fopen(nonexistentfile.txt): failed to open stream: No such file or directory
- Hide Error
Sometimes, we may want to hide error messages in a production environment to prevent the leakage of sensitive information. PHP provides the error_reporting()
function and the display_errors
configuration item to control the display of error messages.
We can set the error reporting level to 0
to turn off the display of error messages:
<?php error_reporting(0); ini_set('display_errors', 0); ?>
Additionally, we can also output error messages to a log file, Instead of displaying it on the page:
<?php ini_set('log_errors', 1); ini_set('error_log', '/path/to/error.log'); ?>
With the above method, we can ensure that the error message is only displayed when we need it, thereby improving the security and stability of the application.
Summary
Good error handling is an integral part of PHP development. With proper error handling, we can better debug the code and solve problems, improving the stability and reliability of the application. This article introduces some common error types and error handling methods, and provides relevant code examples. I hope this knowledge will be helpful to your PHP learning and development.
The above is the detailed content of PHP learning experience: How to handle errors. For more information, please follow other related articles on the PHP Chinese website!

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
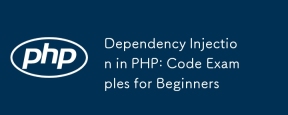
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
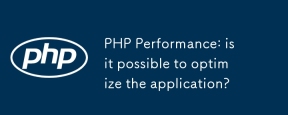
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
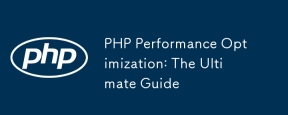
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
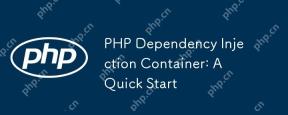
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
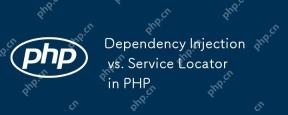
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
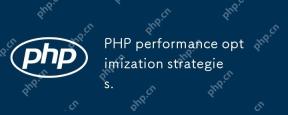
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
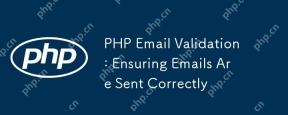
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
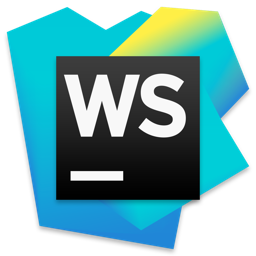
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
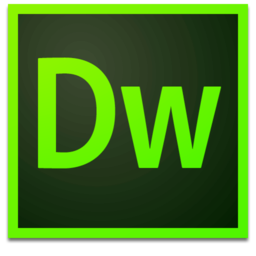
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
