What is a palindrome number?
If given a number (two, three or four digits), reverse the position of each number from front to back, and then vice versa, and then whether the output number after reversing all elements is the same then it It's a palindrome number.
String - String is a storage capsule or a storage method by which we can store a sequence of characters in a Java program.
Array - An array is a collection of similar types of data placed in different locations in a sequential form, making it easier to calculate where the data is in a program by simply adding it to a survey basic data.
Let us understand this through an example -
Given Input: a = 102022220201 Output : Reverse of a = 102022220201 //The Number Is Palindrome = YES//
Given Input: b =20011997 Output : Reverse of b = 79911002 //The Number Is Palimdrome = NO//
Algorithm - Reverse numbers and their palindromes
This is a general algorithm for reversing a number and finding if it is a palindrome -
Step 1 - Check the number of palindromes.
Step 2 - The number should be saved in a non-persistent variable.
Step 3 - Change the numbers.
Step 4 - Now check the number in the temporary variable with the reversed number.
Step 5- If both numbers remain the same or identical, then "it is a palindrome number".
Step 6 - Either/or "This is not".
Syntax: Part 1 - Reverse Numbers
{ int a,res=0,m; Scanner sc=new Scanner(System.in); System.out.println("Enter a number which you want to be reversed"); } m=sc.nextInt(); for( ;m!=0; ) { a=m%10; res=(res*10)+a; m=m/10; }
Syntax: Part 2 - Finding the Palindrome of a Number
if (originalNum1 == reversedNum1) { System.out.println(originalNum1 + " is a Palindrome number."); } else { System.out.println(originalNum1 + " is not a Palindrome number."); }
Let us look at the palindrome program in Java. This would be the simplest form of a palindrome program.
The following methods are very useful for checking palindrome numbers -
Check if a number is a palindrome by using strings and arrays.
Find out palindrome number by using string in Java.
By checking whether the array is a palindrome.
Checking numeric palindromes by using strings and arrays
Steps to check if a number is a palindrome -
Initialization of flag not set or flag value is 0.
Set the array size to n/2.
Check the conditions of the loop. Then set the flag value to 1.
Interruption.
The loop ends and the result is obtained.
Example
import java.util.*; public class palindromeTest{ public static void main(String args[]){ int n; System.out.println("Enter a number to test"); Scanner sc = new Scanner (System.in); n=sc.nextInt(); int r=0 ,q=0 ,num=0 ,p=0; num= n; while(n!=0){ r = r%10; q = n/10; p = p*10+r ; n = q; } if (num==p){ System.out.println("the number is a palindrome number"); } else{ System.out.print("the number is not palindrome"); } } }
Output
Enter a number to test 1111 the number is not palindrome
Find the number of palindromes in Java by using a string -
A palindrome number if get reversed, it will return the same number as the input provided. Using the StringBuffer method with the one way reverse(), we can check the palindrome number by using Java. In below exmaple we will check whether the input string is palindrome or not.
Example
public class Main { public static void main(String[] args) { String str = "Radar2022", reverseStr2 = ""; int strLength1 = str.length(); for (int i = (strLength1 - 1); i >=0; --i) { reverseStr2 = reverseStr2 + str.charAt(i); } if (str.toLowerCase().equals(reverseStr2.toLowerCase())) { System.out.println(str + " -is a Palindrome String."); } else { System.out.println(str + " -is not a Palindrome String."); } } }
Output
Radar2022 -is not a Palindrome String.
By checking whether the array is a palindrome
There are two procedures to check whether the array is a palindrome.
Returns true if there is only one character in the string.
Otherwise, just compare the first and last characters and put them in a recursive method.
Example
public class findapalindrome { static void palindrome(int arr[], int n){ int flag7 = 0; for (int i = 0; i <= n / 2 && n != 0; i++) { if (arr[i] != arr[n - i - 1]) { flag7 = 1; break; } } if (flag7 == 1) System.out.println("The String Is Not Palindrome"); else System.out.println("The String Is Palindrome"); } public static void main(String[] args){ int arr[] = { 100, 200, 300, 222, 1111 }; int n = arr.length; palindrome(arr, n); } }
Output
The String Is Not Palindrome
in conclusion
After definitions and some theory-based procedures, it can be easily said that a palindrome is a number that remains unchanged even after changing the original number.
All these explanations are properly described with examples and for better understanding we have also provided sample programs based on three different methods.
The above is the detailed content of Java program to reverse a number and check if it is a palindrome. For more information, please follow other related articles on the PHP Chinese website!
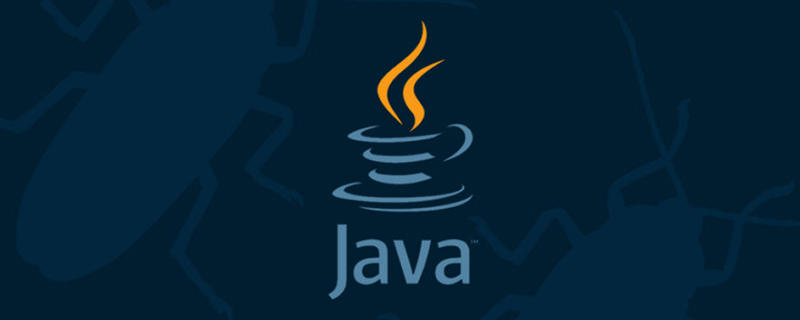
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
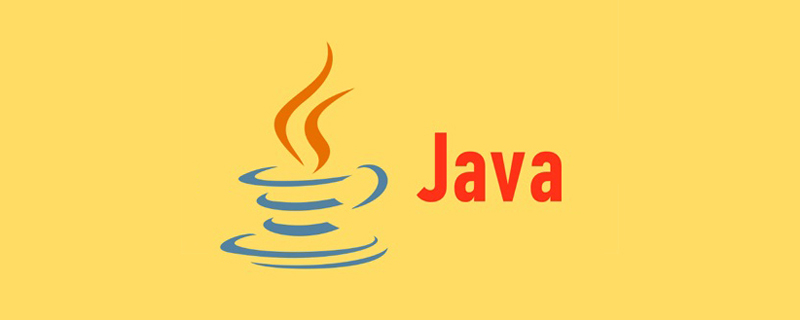
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
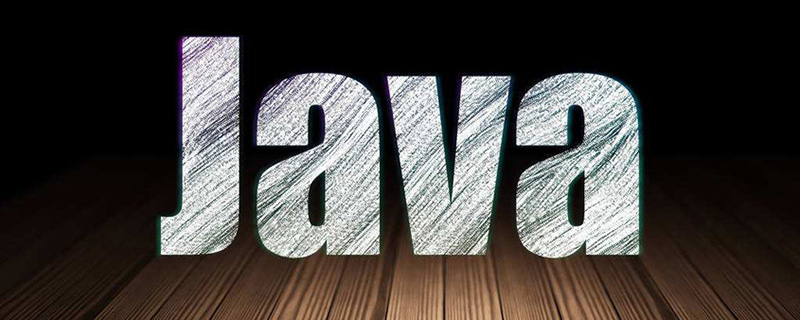
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
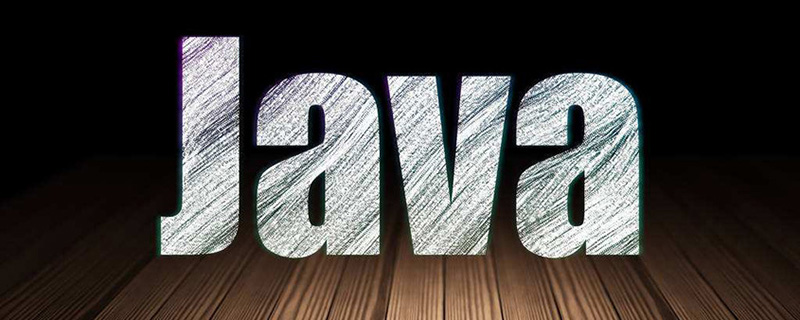
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
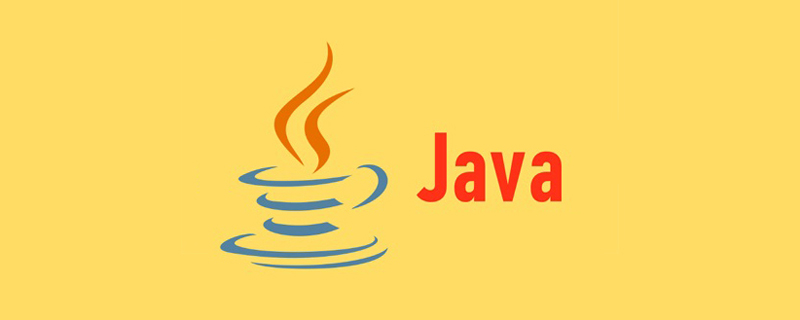
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
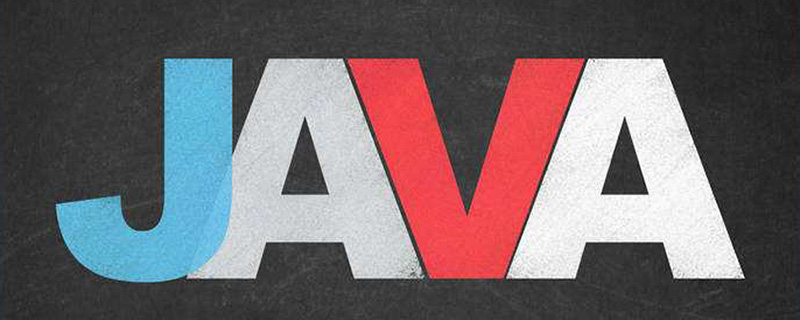
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
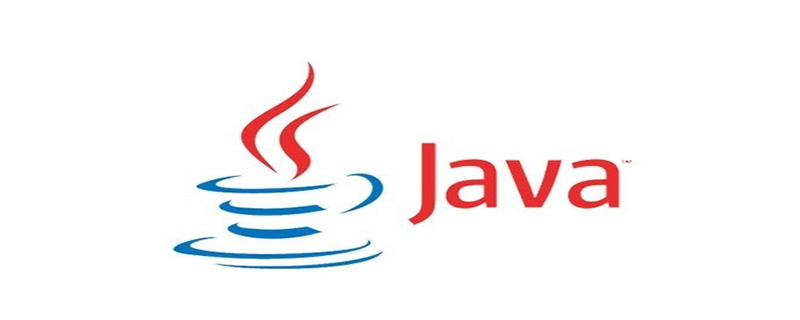
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
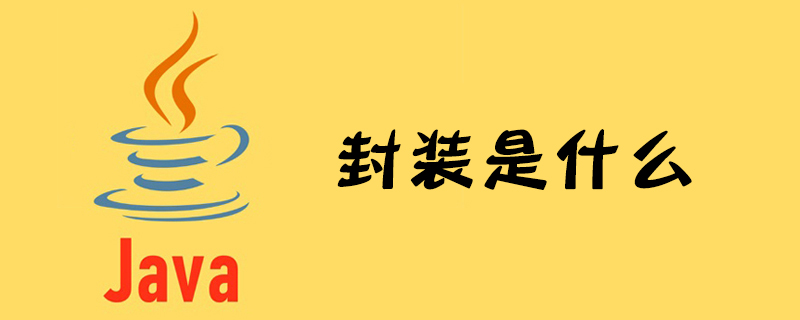
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
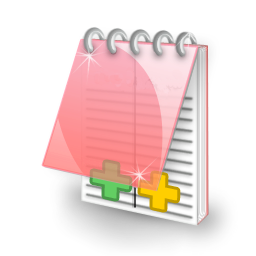
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool