


Golang development skills: Using Baidu AI interface to implement text review
In today's Internet era, the review of text content has become crucial. In order to keep the online environment healthy and orderly, enterprises and developers generally face huge challenges. However, the development of automated text review technology has provided great help in solving this problem. This article will introduce how to use the Golang programming language and Baidu AI interface to implement the text review function.
First, we need to prepare a Baidu Cloud account and create an application in the Baidu Cloud console. After successfully creating the application, we can obtain a pair of AppKey and AppSecret, which will be used in subsequent interface requests.
Next, we need to introduce Golang’s HTTP request library in order to send HTTP requests and process responses. We can use the third-party library "requests" and run the following command in the terminal to install the library:
go get github.com/levigross/grequests
After the installation is complete, import grequests in the code:
import ( "github.com/levigross/grequests" )
Next, we need to write a Function to call Baidu AI interface and implement text review function. Let's take the "text anti-spam" interface as an example. This interface can perform anti-spam processing on text based on the specified text content, and the result returned is whether the text is compliant and the type of violation. The code is as follows:
func TextCensor(text string) (bool, error) { url := "https://aip.baidubce.com/rest/2.0/solution/v1/text_censor/user_defined?access_token=<YOUR_ACCESS_TOKEN>" payload := map[string]interface{}{ "tasks": []map[string]string{ { "content": text, }, }, } headers := map[string]string{ "Content-Type": "application/json;charset=UTF-8", } resp, err := grequests.Post(url, &grequests.RequestOptions{ Headers: headers, JSON: payload, }) if err != nil { return false, err } if !resp.Ok { return false, fmt.Errorf("Request failed with status code: %d", resp.StatusCode) } type Response struct { Result []struct { Conclusion string `json:"conclusion"` } `json:"result"` } var data Response err = resp.JSON(&data) if err != nil { return false, err } if len(data.Result) == 0 { return false, errors.New("Empty response") } if data.Result[0].Conclusion != "合规" { return false, nil } return true, nil }
In the above code, we first need to replace <your_access_token></your_access_token>
in the URL with our own Baidu Cloud access key. Next, we define a payload that contains the text content to be reviewed. We use the grequests.Post
method to send an HTTP POST request and parse the result into a structure.
Finally, we call the TextCensor
function in the main function for text review:
func main() { text := "这是一段违规内容" result, err := TextCensor(text) if err != nil { log.Fatal(err) } if result { fmt.Println("文本合规") } else { fmt.Println("文本违规") } }
The above example code implements the function of using Baidu AI interface for text review. By calling the TextCensor
function, we can conduct compliance review on the specified text and obtain the review results. This is an important step for enterprises and developers to automate text content review.
Summary:
This article introduces how to use the Golang programming language and Baidu AI interface to implement the text review function. By using the text anti-spam interface provided by Baidu Cloud, we can easily implement text compliance review. This provides a more efficient and convenient solution for keeping the network environment healthy and orderly. I hope this article has provided some help for you to implement text auditing functions in Golang development.
The above is the detailed content of Golang development tips: Using Baidu AI interface to implement text review. For more information, please follow other related articles on the PHP Chinese website!
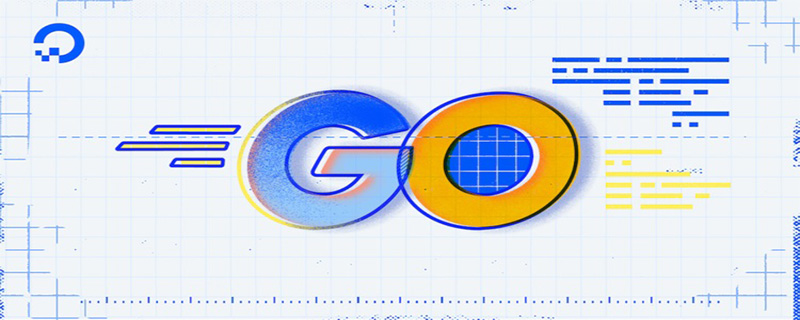
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
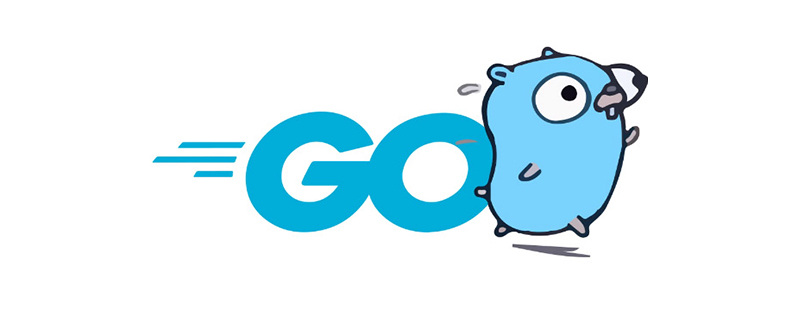
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
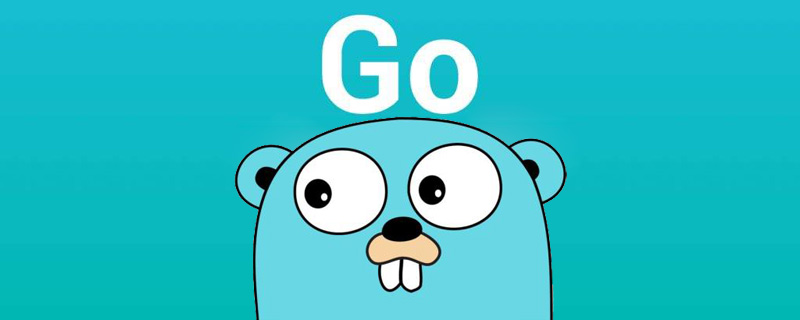
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
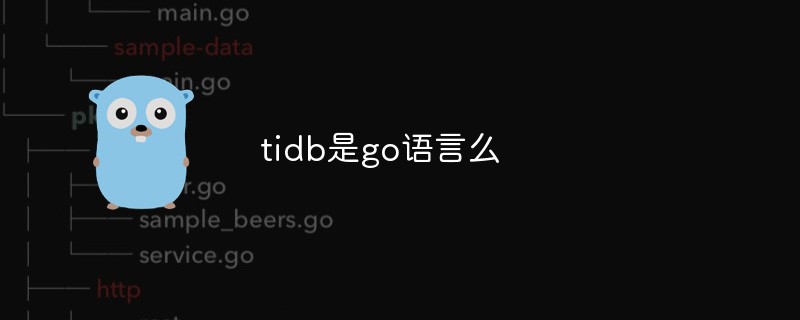
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
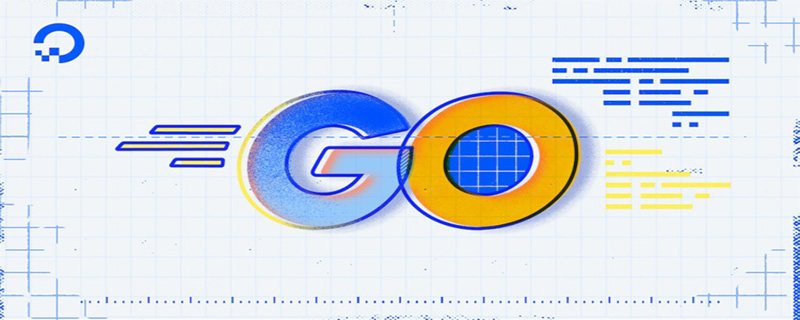
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
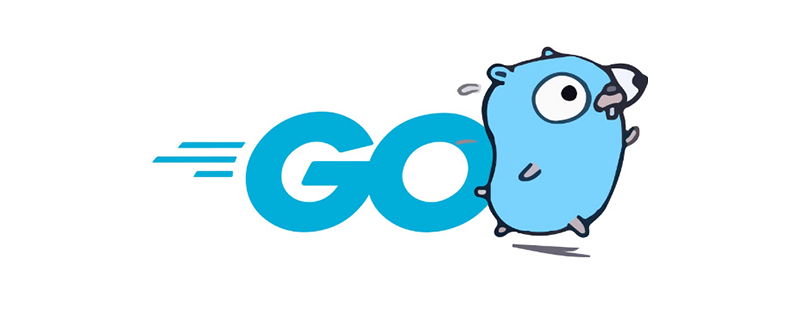
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
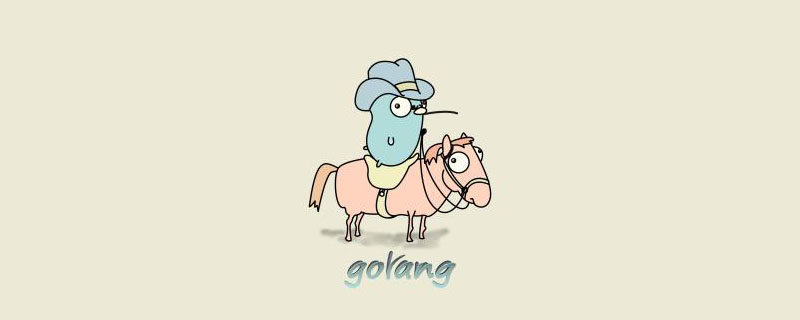
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
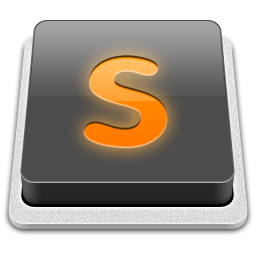
SublimeText3 Mac version
God-level code editing software (SublimeText3)
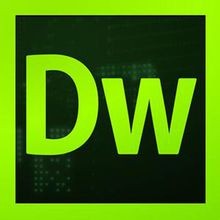
Dreamweaver CS6
Visual web development tools
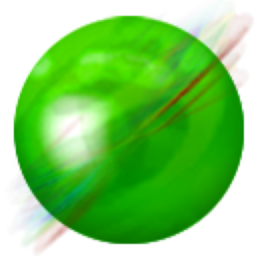
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
