


Use PHP to implement scheduled messages and scheduled tasks for real-time chat function
Use PHP to implement scheduled messages and scheduled tasks for real-time chat function
With the rapid development of the Internet, real-time communication has become an important way for people to communicate. In order to enrich users' interactive experience, many websites and applications have added real-time chat functions. This article will introduce how to use PHP to implement scheduled messages and scheduled tasks in the real-time chat function.
1. Implementation of scheduled messages
Scheduled messages refer to sending messages to specified users at a specified time point. PHP can use timers to achieve this function. The following is a simple sample code:
// 设置定时器 $timer = new Timer(); $timer->setInterval(1000); // 设置定时器间隔为1秒 // 设置定时任务 $timer->onInterval(function() { // 获取待发送的消息 $message = getMessageFromDatabase(); // 获取待发送的用户 $users = getUsersFromDatabase(); // 发送消息给用户 sendMessage($users, $message); }); // 启动定时器 $timer->start();
In the above code, we first create a timer object, and then set the timer interval to 1 second. Next, we use the onInterval
method to set a callback function for the timer, which will be executed when each timer interval is reached. In the callback function, we obtain the message to be sent and the user to be sent from the database, and send the message to the user through the sendMessage
function. Finally, we start the timer through the start
method, which will start triggering the callback function at the set interval.
2. Implementation of scheduled tasks
Scheduled tasks refer to performing certain operations at a specified point in time. PHP can use Cron expressions to achieve this functionality. The following is a simple sample code:
// 检查Cron表达式是否达到触发时间 if (CronExpression::factory('* * * * *')->isDue()) { // 执行定时任务操作 performScheduledTask(); }
In the above code, we use the CronExpression
class to create a Cron expression object that specifies a timer to trigger every minute. Task. Then, we use the isDue
method to check whether the Cron expression reaches the specified time point. If so, execute the performScheduledTask
function, which will perform the specific operations of the scheduled task.
3. Applications combined with real-time chat function
The real-time chat function usually requires operations such as sending system notifications regularly and clearing chat records regularly. We can combine the implementation of scheduled messages and scheduled tasks to write a complete PHP application with real-time chat function. The following is a simple example:
// 设置定时器 $timer = new Timer(); $timer->setInterval(1000); // 设置定时器间隔为1秒 // 设置定时任务 $timer->onInterval(function() { // 检查是否有系统通知应发送 if (CronExpression::factory('* * * * *')->isDue()) { $message = getSystemNotification(); // 获取系统通知消息 $users = getAllUsers(); // 获取所有用户 sendMessage($users, $message); // 发送系统通知消息给所有用户 } // 检查是否需要清理聊天记录 if (CronExpression::factory('0 0 * * *')->isDue()) { deleteExpiredMessages(); // 清理过期聊天记录 } }); // 启动定时器 $timer->start();
In the above code, we added two Cron expressions to the scheduled task. The first Cron expression indicates that a system notification is triggered every minute, and the notification message will be sent to all users through the sendMessage
function. The second Cron expression indicates that the chat record clearing operation is triggered at zero o'clock every day. This operation will clear expired chat records through the deleteExpiredMessages
function.
Through the above code examples, we can see the application of scheduled messages and scheduled tasks in the real-time chat function. In actual development, the trigger time and operation content of scheduled messages and scheduled tasks can be set according to specific needs to meet different business scenarios.
The above is the detailed content of Use PHP to implement scheduled messages and scheduled tasks for real-time chat function. For more information, please follow other related articles on the PHP Chinese website!
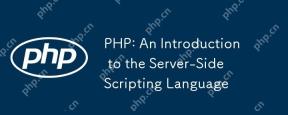
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
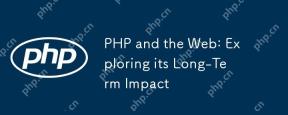
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
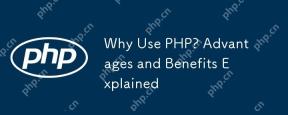
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
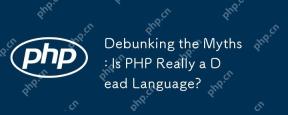
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
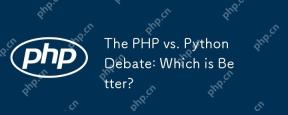
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
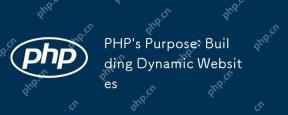
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
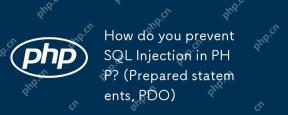
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
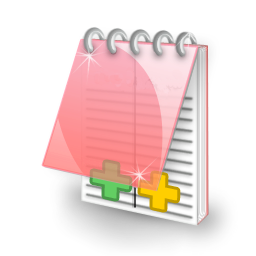
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
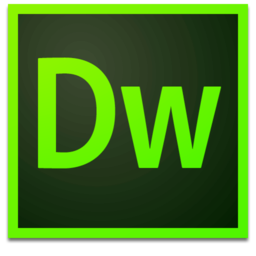
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor