JavaScript developers are always adding new features to the JavaScript language to improve performance and add better functionality. Sometimes, older browser versions don't support new features.
For example, the exponentiation operator was introduced in ES7, and trailing commas in objects are also valid in ES7. Now, while developing the application, we added the exponentiation operator in the application. It will work on newer versions of browsers, but if someone is using a very old version of the browser, they may get errors such as the browser engine not supporting the exponentiation operator.
So, we can use polyfill to avoid this error. Let us break the word polyfill into two parts to understand its meaning. Poly means a lot and fill means filling in the gaps. This means that if a browser does not support the default functionality of JavaScript, a variety of techniques are needed to fill the gaps in browser functionality.
There are two ways to solve the problem that the browser does not support the function. One is a polyfill and the other is a transpiler. A translator converts the code to a lower version so that the browser can support it. For example, we can write code in an ES7 version of JavaScript and then use a transpiler to convert it to ES6 or ES5 so that it is supported by older browsers.
Here we will learn different examples of using polyfill concepts.
grammar
Users can manually implement JavaScript methods using the polyfill concept according to the following syntax.
String.prototype.method_name = function (params) { // implement the code for the method // use this keyword to access the reference object }
We have added methods to the string prototype in the above syntax. method_name represents the method name. We assign a function with multiple parameters to the method.
Example 1 (Includes() method without polyfill)
In the following example, we use the built-in contains() method of the String object. We have defined the string and used the includes() method to check if the string contains a specific word or substring.
<html> <body> <h2 id="Using-the-i-includes-method-without-polyfill-i-in-JavaScript">Using the <i>includes() method without polyfill </i> in JavaScript</h2> <div id = "content"> </div> <script> let content = document.getElementById('content'); let str = "You are welcome on TutorialsPoint's website. Hello users! How are you?"; let isWelcome = str.includes('welcome'); content.innerHTML += "The original string: " + str + "<br>"; content.innerHTML += "The string includes welcome word? " + isWelcome + "<br>"; let isJavaScript = str.includes('javaScript'); content.innerHTML += "The string includes JavaScript word? " + isJavaScript + "<br>"; </script> </body> </html>
Example 2 (Includes() method with polyfill)
In the above example, we used the built-in includes() method. In this example, we will define a polyfill for the includes() method. If any browser does not support the includes() method, it will execute the user-defined includes() method.
Here, we add the includes() method to the prototype of the string object. In the function, if the search string is of type regular expression, we will throw an error. Also, the "pos" parameter is an option, so if the user doesn't pass it, it's treated as nil. Finally, use the indexof() method to check if the string contains the word and return a Boolean value based on the result.
<html> <body> <h2 id="Using-the-i-includes-method-with-polyfill-i-in-JavaScript">Using the <i>includes() method with polyfill </i> in JavaScript</h2> <div id = "content"> </div> <script> let content = document.getElementById('content'); String.prototype.includes = function (str, pos) { // first, check whether the first argument is a regular expression if (str instanceof RegExp) { throw Error("Search string can't be an instance of regular expression"); } // second parameter is optional. So, if it isn't passed as an argument, consider it zero if (pos === undefined) { pos = 0; } content.innerHTML += "The user-defined includes method is invoked! <br>" // check if the index of the string is greater than -1. If yes, string includes the search string. return this.indexOf(str, pos) !== -1; }; let str = "This is a testing string. Use this string to test includes method."; content.innerHTML += `The original string is "` + str +`" <br>`; let isTesting = str.includes('testing'); content.innerHTML += "The string includes testing word? " + isTesting + "<br>"; let isYour = str.includes('your'); content.innerHTML += "The string includes your word? " + isYour + "<br>"; </script> </body> </html>
Example 3 (implementing polyfill for filter() method)
We implemented a polyfill for the filter() method in the example below. We first make sure that the reference array is not empty and that the callback is a function. After that, we iterate over the array and execute the callback function for each array value. If the callback function returns true, we push it to the output array. Finally, we return the output array containing the filtered values
<html> <body> <h2 id="Using-the-i-filter-method-with-polyfill-i-in-JavaScript">Using the <i> filter() method with polyfill </i> in JavaScript</h2> <div id = "content"> </div> <script> let content = document.getElementById('content'); Array.prototype.filter = function (callback) { // check if the reference array is not null if (this === null) throw new Error; // check that callback is a type of function if (typeof callback !== "function") throw new Error; var output = []; // iterate through array for (var k = 0; k < this.length; k++) { // get value from index k var val = this[k]; // call the callback function and, based on a returned boolean value, push the array value in the output array if (callback.call(this, val, k)) { output.push(val); } } return output; }; function getDivisibleBy10(val, k) { // return true if val is divisible by 10. if (val % 10 == 0) { return true; } return false; } let array = [10, 20, 40, 65, 76, 87, 90, 80, 76, 54, 32, 23, 65, 60]; let filtered = array.filter(getDivisibleBy10); content.innerHTML += "The original array is " + JSON.stringify(array) + "<br>"; content.innerHTML += "The filtered array is " + JSON.stringify(filtered) + "<br>"; </script> </body> </html>
This tutorial teaches us how to implement polyfill for the includes() and filter() methods. However, users can use if-else statements to check if the browser supports a specific method. If not, the user-defined method is executed, otherwise the built-in method is executed.
The above is the detailed content of How to use polyfill in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
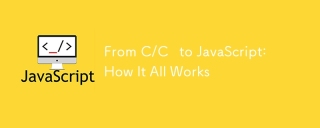
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
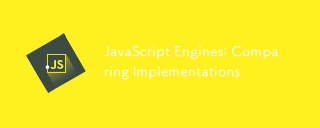
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
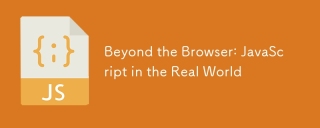
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
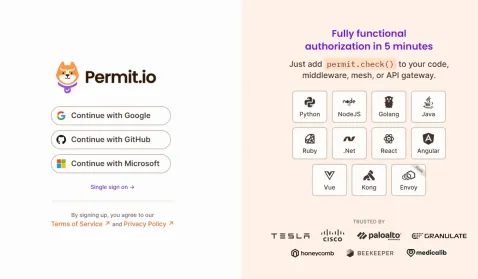
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
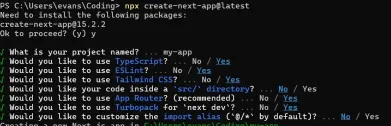
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
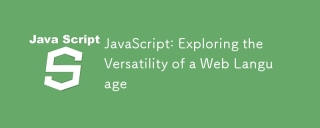
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
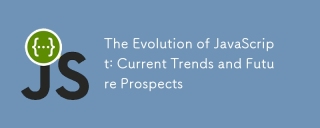
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
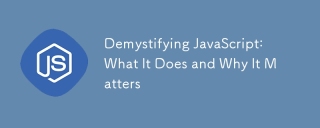
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
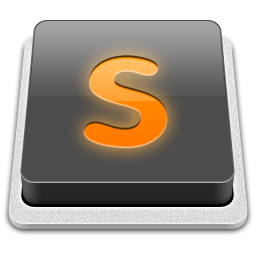
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
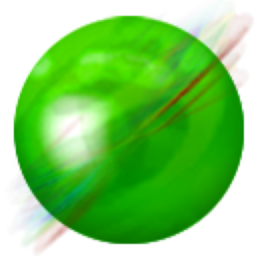
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment