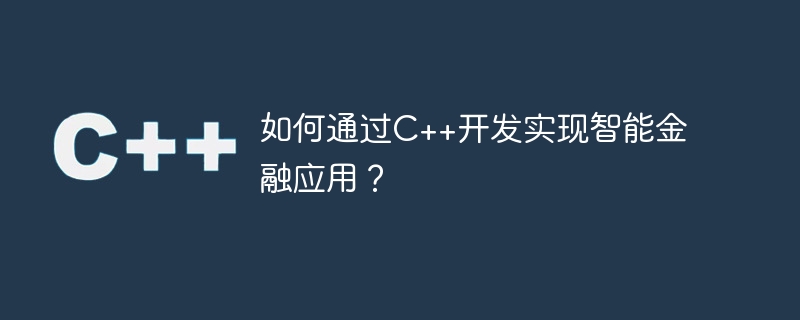
How to implement intelligent financial applications through C development?
Introduction:
With the rapid development of technology, intelligent financial applications have become a hot topic in today's financial industry. By integrating technologies such as artificial intelligence and machine learning with financial operations, the efficiency and accuracy of financial services can be improved. This article will introduce how to use C language to develop intelligent financial applications and provide code examples.
- Determine requirements:
Before starting development, we need to clarify the specific requirements of smart financial applications. For example, we can develop a stock prediction system based on machine learning algorithms, or develop a chatbot based on natural language processing for customer service, etc. Based on specific needs, we can clarify the direction and goals of development.
- Data preparation:
For smart financial applications, data is very important. We need to obtain and prepare enough data to analyze and train algorithms. For example, for a stock prediction system, we need to obtain historical stock market data and clean and organize the data.
- Algorithm selection:
When developing smart financial applications, we need to choose appropriate algorithms to process data and make predictions or decisions. C provides a wealth of data processing and algorithm libraries, and we can choose the appropriate library to use according to our needs. For example, we can use OpenCV for image processing, or use TensorFlow to build a deep learning model.
- Code implementation:
Next, we will use C language to implement the core functions of smart financial applications. Suppose we want to develop a stock prediction system based on machine learning algorithms. We can implement it according to the following process:
#include <iostream>
#include <vector>
// 定义机器学习模型类
class MLModel {
public:
void train(std::vector<double> data) {
// 训练模型的代码实现
}
double predict(std::vector<double> data) {
// 预测结果的代码实现
}
};
int main() {
std::vector<double> trainingData = {1.0, 2.0, 3.0, 4.0, 5.0};
std::vector<double> testingData = {6.0, 7.0, 8.0, 9.0, 10.0};
// 创建机器学习模型对象
MLModel model;
// 训练模型
model.train(trainingData);
// 预测结果
double result = model.predict(testingData);
std::cout << "预测结果:" << result << std::endl;
return 0;
}
In the above example code, we define a MLModel class to train and predict results . We used std::vector to store data, train the model through the train() function, and make predictions through the predict() function.
- Result evaluation:
After the development is completed, we need to evaluate the results of the smart financial application. For example, in a stock prediction system, we can use historical data to verify the accuracy of the prediction results.
- Performance Optimization:
Finally, we can optimize the performance of smart financial applications. For example, we can use multi-threading or parallel computing to speed up calculations, or optimize algorithms to improve accuracy.
Conclusion:
Implementing intelligent financial applications through C language development is a complex and challenging task. However, through reasonable planning, selecting appropriate algorithms and tools, and implementing and optimizing code, we can develop efficient and accurate smart financial applications.
Reference materials:
- C Official documentation: https://en.cppreference.com/
- OpenCV official website: https://opencv.org/
- TensorFlow official website: https://www.tensorflow.org/
The above is the detailed content of How to implement smart financial applications through C++ development?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn