


Solve Python error: TypeError: unsupported operand type(s) for +: 'str' and 'int'
Solution to Python error: TypeError: unsupported operand type(s) for : 'str' and 'int'
We often encounter this when writing programs in Python All kinds of errors. One of the common errors is "TypeError: unsupported operand type(s) for : 'str' and 'int'". This error is usually caused by incorrect operations between string types and integer types.
The reason for this error is that in Python, strings (str) and integers (int) are different data types, and their operations cannot be mixed. When we try to add a string and an integer, Python will throw a type error, indicating that operations between string and integer types are not supported.
In order to better understand this error, let's look at a code example:
name = "Alice" age = 25 message = "My name is " + name + " and I am " + age + " years old." print(message)
When you run this code, "TypeError: unsupported operand type(s) for : 'str' will appear and 'int'" error.
To solve this error, we need to convert the integer type variable to the string type and then perform the addition operation. In Python, there are several ways to convert an integer to a string. The following are some commonly used methods:
- Use str() function for type conversion
name = "Alice" age = 25 message = "My name is " + name + " and I am " + str(age) + " years old." print(message)
This code uses the str() function to convert the integer type variable age Convert it to a string. Then the string is added to other strings and finally the correct result is obtained.
- Use the format() method for formatting
name = "Alice" age = 25 message = "My name is {} and I am {} years old.".format(name, age) print(message)
Using the format() method can more conveniently perform string formatting operations. In this example, we use {} as placeholder, and then pass in the variables name and age in the format() method, which will replace the placeholder in order to get the final string.
- Use f-string for formatting (Python 3.6 and above)
name = "Alice" age = 25 message = f"My name is {name} and I am {age} years old." print(message)
f-string is a new character introduced in Python 3.6 and above String formatting method. In f-string, we can use curly braces {} directly in the string to refer to variables and add an f character before the variable. In this way, the variable will be automatically converted to a string and replaced into the corresponding curly braces.
Through the above three methods, we can solve the "TypeError: unsupported operand type(s) for : 'str' and 'int'" error. Because in this error we involve the addition operation of strings and integers, we need to convert the integer type variable to the string type so that the operation can be performed.
The above is the detailed content of Solve Python error: TypeError: unsupported operand type(s) for +: 'str' and 'int'. For more information, please follow other related articles on the PHP Chinese website!
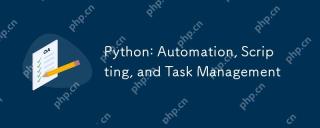
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
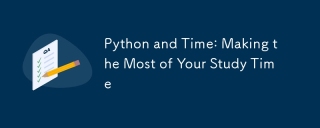
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
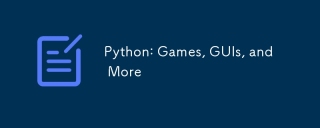
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
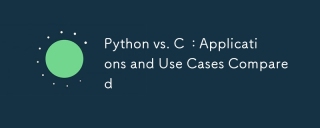
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
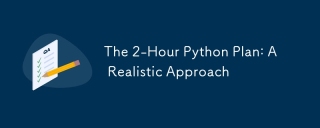
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
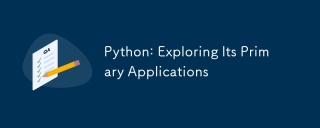
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
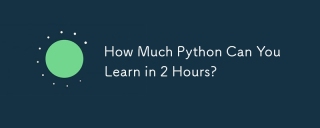
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
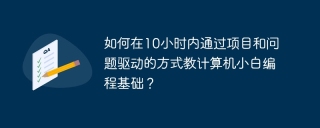
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.