In this tutorial, we will learn how to get the scaled width of text using FabricJS. We can display text on the canvas by adding an instance of Fabric.Text. Not only does it allow us to move, scale and change the dimensions of text, but it also provides additional features such as text alignment, text decoration, line height, which are available through the properties textAlign, underline and lineHeight respectively. We can also find the scaled width of an object using the getScaledWidth method.
grammar
getScaledWidth()
Example 1
Use getScaledWidth method
Let's look at a code example to see the output logged when using the getScaledWidth method. In this case, the width of the object is returned.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2 id="Using-the-getScaledWidth-method">Using the getScaledWidth method</h2> <p>You can open console from dev tools and see that the width value is being displayed in the console</p> <canvas id="canvas"></canvas> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiate a text object var text = new fabric.Text("Add sampletext here", { width: 300, fill: "green", fontWeight: "bold", }); // Add it to the canvas canvas.add(text); // Using getScaledWidth method console.log("The scaled width is", text.getScaledWidth()); </script> </body> </html>
Example 2
Use getScaledWidth method and pass scaleX property
Let's look at a code example to see the output logged when the getScaledWidth method is used in conjunction with the scaleY property. In this case, the final scaled width will be displayed in the console.
<!DOCTYPE html> <html> <head> <!-- Adding the Fabric JS Library--> <script src="https://cdnjs.cloudflare.com/ajax/libs/fabric.js/510/fabric.min.js"></script> </head> <body> <h2 id="Using-the-getScaledWidth-method-and-passing-the-scaleX-property">Using the getScaledWidth method and passing the scaleX property</h2> <p>You can open console from dev tools and see that the width value is being displayed in the console has increased </p> <canvas id="canvas"></canvas> <script> // Initiate a canvas instance var canvas = new fabric.Canvas("canvas"); canvas.setWidth(document.body.scrollWidth); canvas.setHeight(250); // Initiate a text object var text = new fabric.Text("Add sampletext here", { width: 300, fill: "green", fontWeight: "bold", scaleX: 2, }); // Add it to the canvas canvas.add(text); // Using getScaledWidth method console.log("The scaled width is", text.getScaledWidth()); </script> </body> </html>
The above is the detailed content of How to get the scaled width of text using FabricJS. For more information, please follow other related articles on the PHP Chinese website!
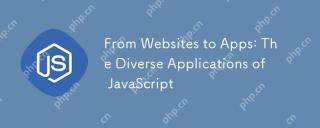
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
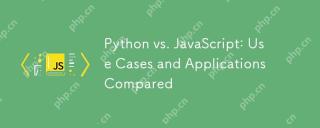
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
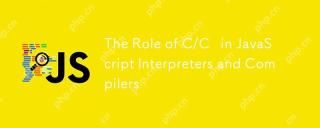
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
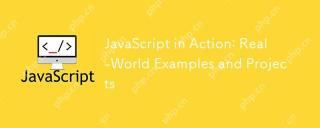
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
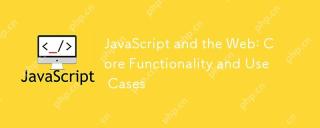
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
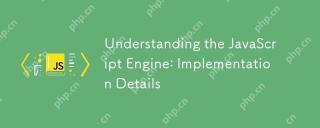
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
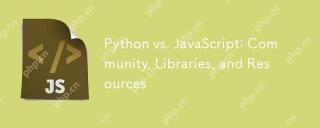
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
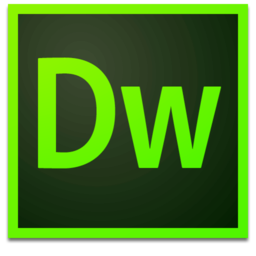
Dreamweaver Mac version
Visual web development tools
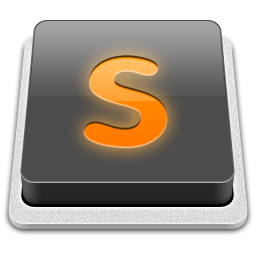
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
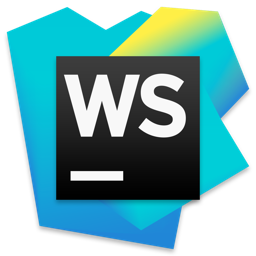
WebStorm Mac version
Useful JavaScript development tools