In this tutorial, we will build a project using HTML, CSS, and JavaScript that will generate random references from an API (type.fit).
Steps
We will follow some basic steps -Create HTML elements and templates
Use Add style CSS
JavaScript logic
Create HTML elements and templates
The first step is to create HTML elements and templates. We first add a box that will display the item and then add a button that when clicked it will display a new random quote in the box and then use the span tag to display the quote symbol type font awesome icon.
HTML
<!DOCTYPE html> <html> <head> <title>Random quote generator using HTML, CSS and JavaScript</title> </head> <body> <div class="boxSize"> <h1> <i class="fas fa-quote-left"></i> <span class="QuoteText" id="QuoteText"></span> <i class="fas fa-quote-right"></i> </h1> <p class="QuoteWR" id="author"></p> <hr/> <div class="QuoteBtn"> <button class="GenerateQuote_next" onclick="GenerateQuote()">Next quote</button> </div> </div> </body> </html>
Adding styles using CSS
Now we will add styles to the HTML project we have written. We will add properties like box shadow, padding and margin to the box, for the author we will use the cursive font family and we will also add a background color to the box as well as the body to make it look great.
We will work on internal CSS so as to avoid making extra files, but making external files for CSS and JavaScript is considered a good practice.
We will be adding CDN Font Awesome links in our heads in order to use the font Awesome icons in our applications.
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA==" crossorigin="anonymous" />
CSS
body{ min-height:100vh; transition: 0.5s; display: flex; background-color: #A4E5E0; align-items: center; justify-content: center; } .boxSize { margin: 10px; border-radius: 10px; width: 800px; display: flex; flex-direction: column; align-items: center; padding: 30px; box-shadow: 0 4px 10px rgba(0, 0, 0, 0.6); background-color: rgba(255, 255, 255, 0.3); } .fa-quote-left, .fa-quote-right { font-size: 35px; color: blue; } .QuoteText { text-align: center; font-size: 40px; font-weight: bold; } .author { margin:10px; text-align: right; font-size: 25px; font-style: italic; font-family: cursive; } .QuoteBtn { width: 100%; display: flex; margin-top:10px; } .GenerateQuote_next { font-size:18px; border-radius: 5px; cursor:pointer; padding: 10px; margin-top: 5px; font-weight: bold; color: white; background-color: #2C5E1A } .GenerateQuote_next:hover{ background-color: black; }
JavaScript Logic
Now the logic part appears in the scene, this part will be the JavaScript, we will define which elements will do what work and use the API to Getting and Displaying Data So let's take a closer look at our JavaScript functions.
Steps
We have to follow the following steps to complete our work -
-
Get quote data from type.fit API.
The received data will be stored in the array.
Get a random index variable named "randomIdx".
Then set the "randomIdx" maximum size to the quote list length.
Get the citation and author using the generated random index
Now we assign the obtained value to the project element.
JavaScript
var url="https://type.fit/api/quotes"; const response=await fetch(url); const Quote_list = await response.json(); const randomIdx = Math.floor(Math.random()*Quote_list.length); const quoteText=Quote_list[randomIdx].text; const auth=Quote_list[randomIdx].author; if(!auth) author = "Anonymous"; console.log (quoteText); console.log (auth);
Let’s embed the JavaScript function code to make it work.
Example - Complete Program
Below is the complete program to build a random quote generator.
Ramdom quote generator using HTML, CSS and JavaScript <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css" integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA==" crossorigin="anonymous" /><script> const GenerateQuote = async () =>{ var url="https://type.fit/api/quotes"; const response=await fetch(url); const Quote_list = await response.json(); const randomIdx = Math.floor(Math.random()*Quote_list.length); const quoteText=Quote_list[randomIdx].text; const auth=Quote_list[randomIdx].author; if(!auth) author = "Anonymous"; document.getElementById("QuoteText").innerHTML=quoteText; document.getElementById("author").innerHTML="~ "+auth; } GenerateQuote(); </script>
So we have learned about making a quote generator app, hope it helps.
The above is the detailed content of How to build a random quote generator using HTML, CSS and JavaScript?. For more information, please follow other related articles on the PHP Chinese website!
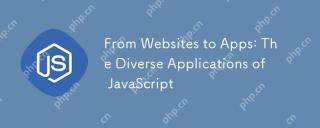
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
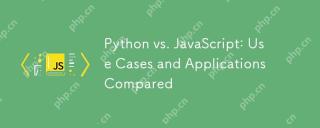
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
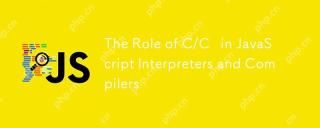
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
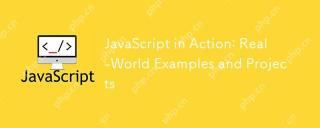
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
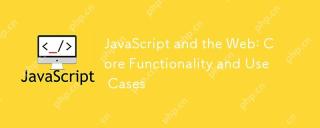
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
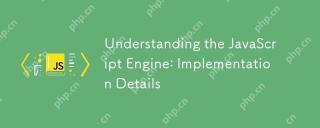
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
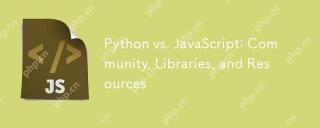
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.