


Use Python's range() function to generate a sequence of numbers in a specified range
Use Python's range() function to generate a number sequence in a specified range
In Python programming, a very common requirement is to generate a series of number sequences. These numbers can be used for various operations such as iteration, looping, indexing, etc. To meet this need, Python provides a very convenient built-in function range().
The syntax of the range() function is as follows:
range(start, stop, step)
Among them, start represents the starting value (optional, default is 0), stop represents termination Value (required), step represents the step size (optional, default is 1).
Here are some examples of using the range() function to generate a number sequence:
Example 1: Generate a number sequence from 0 to 4 (excluding 4)
for num in range(4): print(num)
Output :
0 1 2 3
Example 2: Generate a sequence of numbers from 2 to 8 (excluding 8) with a step size of 2
for num in range(2, 8, 2): print(num)
Output:
2 4 6
Example 3: Generate from A sequence of numbers from 10 to 1 (excluding 1) with a step size of -1
for num in range(10, 1, -1): print(num)
Output:
10 9 8 7 6 5 4 3 2
Example 4: Generate a list containing a sequence of numbers
num_list = list(range(5)) print(num_list)
Output:
[0, 1, 2, 3, 4]
In addition to generating numerical sequences, the range() function can also be used with other built-in functions (such as len()) to facilitate iteration and indexing operations.
Example 5: Use the range() function to generate a sequence of numbers, and pair it with the len() function for iteration and indexing
my_list = ['apple', 'banana', 'orange', 'grape', 'watermelon'] for index in range(len(my_list)): print(f"index: {index}, value: {my_list[index]}")
Output:
index: 0, value: apple index: 1, value: banana index: 2, value: orange index: 3, value: grape index: 4, value: watermelon
Summary:
The range() function is a very commonly used function in Python, which can generate a sequence of numbers in a specified range. By setting the start value, end value and step size, you can flexibly generate a variety of different number sequences. When writing Python programs, using the range() function can make the code more concise and efficient. We hope that the introduction and examples in this article can help readers better understand and apply the range() function.
The above is the detailed content of Use Python's range() function to generate a sequence of numbers in a specified range. For more information, please follow other related articles on the PHP Chinese website!
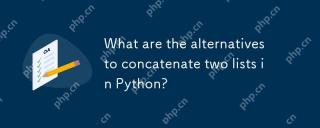
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
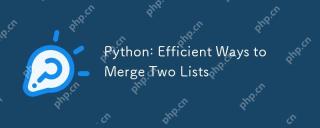
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
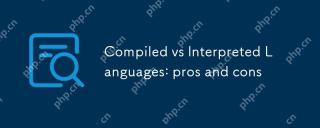
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
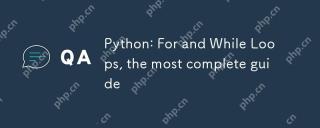
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
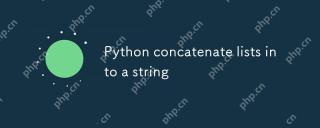
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
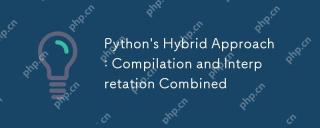
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
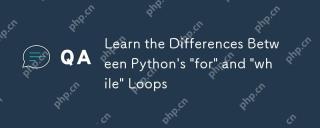
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
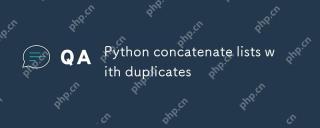
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
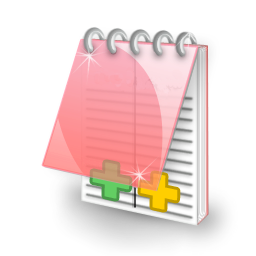
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
