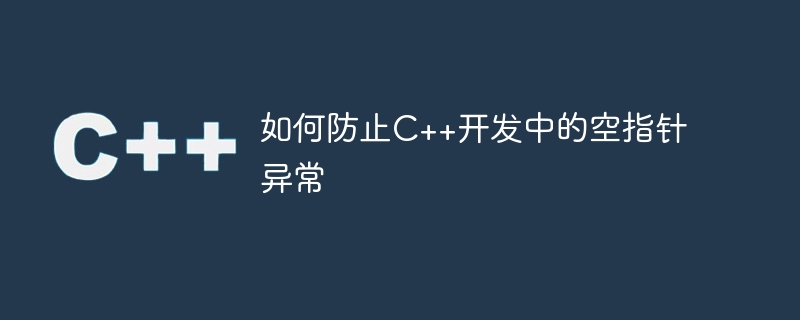
How to prevent null pointer exceptions in C development
Abstract: This article mainly introduces how to prevent null pointer exceptions in C development, including reasonable use of pointers, avoiding Dangling pointers, using smart pointers, etc.
Keywords: C development, null pointer exception, pointer, dangling pointer, smart pointer
Introduction: In C development, null pointer exception is a common and troublesome problem. Null pointer exceptions occur when we do not handle pointers correctly in our code or use dangling pointers. This article will introduce some methods to prevent null pointer exceptions to help readers improve the quality and reliability of their code.
1. Reasonable use of pointers
- Initialize pointers: Before using a pointer, be sure to initialize it to nullptr (standard after C 11) or NULL (early versions). This ensures that the pointer does not contain any garbage values after it is declared.
- Check whether the pointer is null: Before using a pointer, be sure to check whether it is null. You can use conditional statements such as if statements or ternary operators to check whether the pointer is null, and take appropriate processing measures as needed.
- Avoid using uninitialized pointers: Using uninitialized pointers is dangerous and may lead to unpredictable results. When declaring a pointer variable, it is best to immediately initialize it to nullptr or a suitable value.
2. Avoid dangling pointers
- Delete the pointer and then set it empty: When we use the new operator to allocate memory for the pointer, we should set it after using it. Is empty. This avoids problems caused by dangling pointers.
- Avoid multiple releases of pointers: When we use delete to release the memory pointed to by the pointer, we should set the pointer to null to avoid releasing the pointer again in subsequent code.
- Pay attention to the life cycle of pointers: In C, the life cycle of a pointer should be consistent with the object it points to. When an object is destroyed, the pointer to the object should be released.
3. Use smart pointers
- The concept of smart pointers: A smart pointer is a pointer that can automatically manage the life cycle of the object pointed by the pointer. C 11 introduced two types of smart pointers, shared_ptr and unique_ptr.
- shared_ptr: shared_ptr allows multiple smart pointers to share the same object. It manages the release of the object through a counter. When the counter decreases to 0, shared_ptr automatically releases the object.
- unique_ptr: unique_ptr is a smart pointer with exclusive ownership. It guarantees that only one smart pointer has ownership of the object, and that the object will be automatically released after its life cycle is over.
Conclusion: Null pointer exceptions are a common problem in C development, but we can take some preventive measures to reduce their occurrence. This article introduces methods such as rational use of pointers, avoiding dangling pointers, and using smart pointers to help readers better prevent null pointer exceptions and improve the quality and reliability of code.
References:
- https://en.cppreference.com/w/cpp/memory/shared_ptr
- https://en.cppreference.com /w/cpp/memory/unique_ptr
(Total word count: about 500 words)
The above is the detailed content of How to prevent null pointer exceptions in C++ development. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn