How to deal with string concatenation problems in C++ development
How to deal with string splicing problems in C development
In C development, string splicing is a common task. Whether you are concatenating multiple strings together or concatenating strings with other data types, handling string concatenation correctly is critical to your program's performance and readability. This article will introduce some common methods and techniques to help readers effectively deal with string splicing problems in C development.
1. Use operator overloading for string splicing
String splicing in C can be achieved by overloading the addition operator. By overloading the addition operator, we can concatenate multiple strings together without destroying the original string.
The sample code is as follows:
#include <iostream> #include <string> std::string operator+(const std::string& lhs, const std::string& rhs) { std::string result = lhs; result += rhs; return result; } int main() { std::string str1 = "Hello, "; std::string str2 = "world!"; std::string result = str1 + str2; std::cout << result << std::endl; return 0; }
In the above code, we concatenate two strings together by overloading the addition operator. When running, the output is "Hello, world!".
By using operator overloading to implement string concatenation, we can make the code more concise and readable. However, when multiple strings need to be concatenated, a temporary string object is created each time the addition operation is performed, which may cause a performance loss. Therefore, in scenarios where string concatenation is frequently performed, other more efficient methods need to be considered.
2. Use std::stringstream for string splicing
The C standard library provides a convenient class template std::stringstream, which can be used to convert different types of data into strings and spliced.
The sample code is as follows:
#include <iostream> #include <string> #include <sstream> int main() { std::stringstream ss; int num = 10; std::string str1 = "The number is "; std::string str2 = ss.str(); ss << num; std::string result = str1 + str2; std::cout << result << std::endl; return 0; }
In the above code, we create a std::stringstream object, and then write the integer type variable into the std::stringstream object. Convert to string. Finally, we concatenate different types of strings and output the results.
Using std::stringstream for string concatenation can reduce the creation of temporary variables, thus improving performance. At the same time, std::stringstream also provides flexible control methods, which can more easily handle different types of data for splicing.
3. Use the append function of std::string for string splicing
In addition to using operator overloading and std::stringstream, the std::string class in the C standard library also provides a The append function can be used to append another string to the end of the current string.
The sample code is as follows:
#include <iostream> #include <string> int main() { std::string str1 = "Hello, "; std::string str2 = "world!"; str1.append(str2); std::cout << str1 << std::endl; return 0; }
In the above code, we use the append function of std::string to append the string str2 to the end of the string str1 to realize the splicing of strings. When running, the output is "Hello, world!".
Compared with operator overloading and std::stringstream, the append function of std::string is more intuitive and easier to use. However, when multiple strings need to be spliced, calling the append function multiple times may lead to frequent memory allocation and copy operations, and performance may be degraded.
To sum up, the string splicing problem in C development can be handled through operator overloading, std::stringstream and std::string’s append function. Choosing the appropriate method according to the actual situation can make the code more concise, efficient and readable, and improve the performance and maintainability of the program. However, it should be noted that when string splicing is performed frequently, a method with better performance should be selected to avoid performance loss.
The above is the detailed content of How to deal with string concatenation problems in C++ development. For more information, please follow other related articles on the PHP Chinese website!
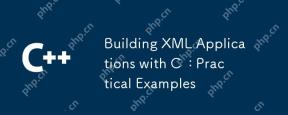
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
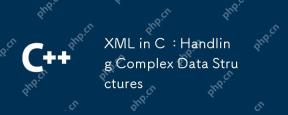
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
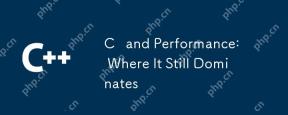
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
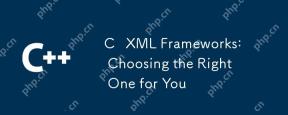
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.
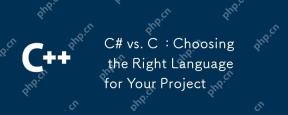
C# is suitable for projects that require development efficiency and type safety, while C is suitable for projects that require high performance and hardware control. 1) C# provides garbage collection and LINQ, suitable for enterprise applications and Windows development. 2)C is known for its high performance and underlying control, and is widely used in gaming and system programming.
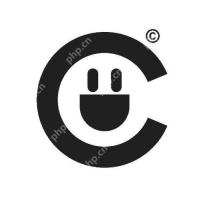
C code optimization can be achieved through the following strategies: 1. Manually manage memory for optimization use; 2. Write code that complies with compiler optimization rules; 3. Select appropriate algorithms and data structures; 4. Use inline functions to reduce call overhead; 5. Apply template metaprogramming to optimize at compile time; 6. Avoid unnecessary copying, use moving semantics and reference parameters; 7. Use const correctly to help compiler optimization; 8. Select appropriate data structures, such as std::vector.
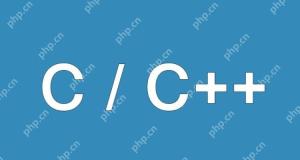
The volatile keyword in C is used to inform the compiler that the value of the variable may be changed outside of code control and therefore cannot be optimized. 1) It is often used to read variables that may be modified by hardware or interrupt service programs, such as sensor state. 2) Volatile cannot guarantee multi-thread safety, and should use mutex locks or atomic operations. 3) Using volatile may cause performance slight to decrease, but ensure program correctness.
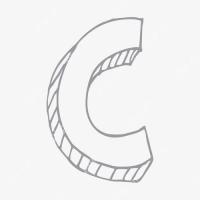
Measuring thread performance in C can use the timing tools, performance analysis tools, and custom timers in the standard library. 1. Use the library to measure execution time. 2. Use gprof for performance analysis. The steps include adding the -pg option during compilation, running the program to generate a gmon.out file, and generating a performance report. 3. Use Valgrind's Callgrind module to perform more detailed analysis. The steps include running the program to generate the callgrind.out file and viewing the results using kcachegrind. 4. Custom timers can flexibly measure the execution time of a specific code segment. These methods help to fully understand thread performance and optimize code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
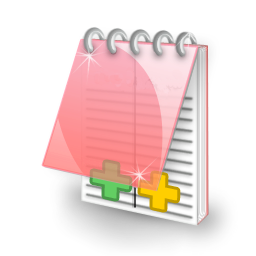
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
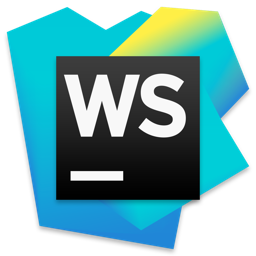
WebStorm Mac version
Useful JavaScript development tools
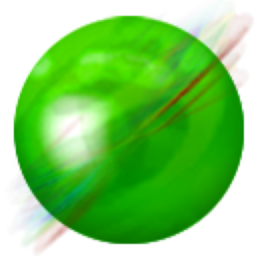
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
