How to perform gradient filtering on images using Python
How to use Python to perform gradient filtering on images
Gradient filtering is a technique commonly used in digital image processing and is used to detect edge and contour information in images. In Python, we can use the OpenCV library to implement gradient filtering. This article will introduce how to use Python to perform gradient filtering on images, and attach code examples for reference.
The principle of gradient filtering is to determine the position of the edge by calculating the difference in pixel values around the pixel point. Generally speaking, edges in an image are usually represented as areas where the gray value of the image changes more drastically. Therefore, gradient filtering can find edges by calculating the first or second order differential of the image grayscale.
The following is a code example using Python and OpenCV libraries to implement gradient filtering:
import cv2 import numpy as np # 读取图片 image = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE) # 使用Sobel算子计算图像梯度 gradient_x = cv2.Sobel(image, cv2.CV_64F, 1, 0, ksize=3) gradient_y = cv2.Sobel(image, cv2.CV_64F, 0, 1, ksize=3) # 计算梯度幅值 gradient_magnitude = np.sqrt(np.square(gradient_x) + np.square(gradient_y)) # 将梯度幅值映射到0-255的灰度空间 gradient_magnitude = cv2.normalize(gradient_magnitude, None, 0, 255, cv2.NORM_MINMAX, cv2.CV_8U) # 显示原图和梯度图像 cv2.imshow('original', image) cv2.imshow('gradient', gradient_magnitude) cv2.waitKey(0) cv2.destroyAllWindows()
First, we use the cv2.imread()
function to read a grayscale image. Here you need to specify the path and reading mode of the image: cv2.IMREAD_GRAYSCALE
means reading the image in grayscale mode.
Next, we use the cv2.Sobel()
function to calculate the gradient of the image. The parameters here include the input image, the order in which the gradient is calculated (x direction or y direction), the order of the derivative, and the size of the Sobel operator. The Sobel operator is a commonly used edge detection operator that calculates the gradient by performing first-order differentiation on the image gray value.
Then, we can get the gradient amplitude by performing square and square root operations on the gradient in the x and y directions. This operation uses the functions np.square()
and np.sqrt()
provided by the NumPy library.
Finally, we map the gradient amplitude to the grayscale space of 0-255 and use the cv2.normalize()
function for normalization.
Finally, we use the cv2.imshow()
function to display the original image and gradient image, and use cv2.waitKey()
and cv2.destroyAllWindows( )
The function waits for the user's operation and closes the window.
With the above code, we can perform gradient filtering on the input image and display the results. If you want to implement other gradient filtering algorithms, you can try using the cv2.filter2D()
function, which provides a more flexible convolution operation.
Gradient filtering is a technique commonly used in digital image processing, which can help us extract edge and contour information in images. I hope the content of this article is helpful to you and can lead you to further learn and explore the field of image processing.
The above is the detailed content of How to perform gradient filtering on images using Python. For more information, please follow other related articles on the PHP Chinese website!
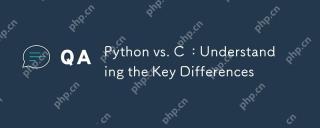
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
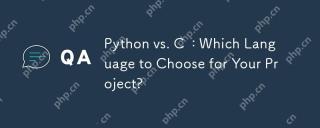
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
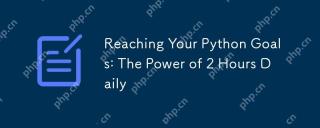
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
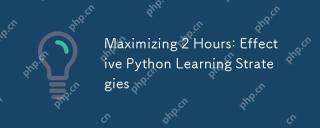
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
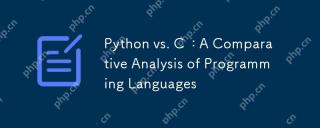
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
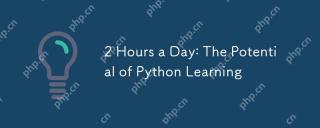
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
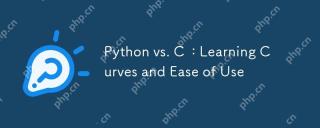
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
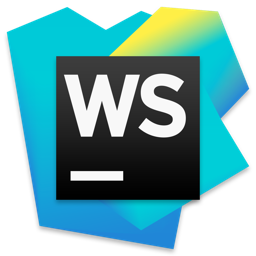
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
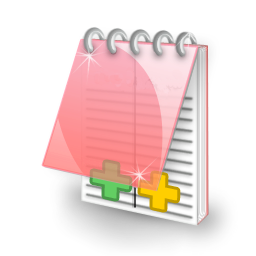
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software