在Laravel中,您可以使用Hash外观模块来处理密码。它具有bcrypt函数,可以帮助您安全地存储密码。
Hash门面bcrypt()方法是一种强大的密码哈希方式。它可以防止恶意用户破解使用bcrypt()生成的密码。
The hashing details are available inside config/hashing.php. The default driver has bcrypt() as the hashing to be used.
Hashing Passwords
要使用Hash Facade,您需要包含以下类:
Illuminate\Support\Facades\Hash
Example
要对密码进行哈希处理,您可以使用make()方法。以下是一个哈希密码的示例
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; use Illuminate\Support\Facades\Hash; class StudentController extends Controller { public function index() { echo $hashed = Hash::make('password', [ 'rounds' => 15, ]); } }
Output
The output of the above code is
$2y$15$QKYQhdKcDSsMmIXZmwyF/.sihzQDhxtgF5WNiy4fdocNm6LiVihZi
Verifying if the password matches with a hashed password
要验证明文文本即Hash::make中使用的文本是否与哈希值匹配,可以使用check()方法。
如果纯文本与哈希密码匹配,check()方法返回true,否则返回false。
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; use Illuminate\Support\Facades\Hash; class StudentController extends Controller { public function index() { $hashed = Hash::make('password', [ 'rounds' => 15, ]); if (Hash::check('password', $hashed)) { echo "Password matching"; } else { echo "Password is not matching"; } } }
Output
The output of the above code is
Password matching
使用check()方法
让我们现在通过提供错误的纯文本来测试,并查看 check() 方法的响应。
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; use Illuminate\Support\Facades\Hash; class StudentController extends Controller { public function index() { $hashed = Hash::make('password', [ 'rounds' => 15, ]); if (Hash::check('password123', $hashed)) { echo "Password matching"; } else { echo "Password is not matching"; } } }
我们在哈希中使用的纯文本是“password”。在check方法中,我们使用了“password123”,因为文本与哈希文本不匹配,所以输出为“密码不匹配”。
Output
当您在浏览器中执行时,输出将是 -
Password is not matching
对密码进行两次哈希
Let us now hash the same text twice and compare it in the check() method −
$testhash1 = Hash::make('mypassword'); $testhash2 = Hash::make('mypassword'); if (Hash::check('mypassword', $testhash1) && Hash::check('mypassword', $testhash2)) { echo "Password matching"; } else { echo "Password not matching"; }
You can test the complete code in the browser as shown below −
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; use Illuminate\Support\Facades\Hash; class StudentController extends Controller { public function index() { $testhash1 = Hash::make('mypassword'); $testhash2 = Hash::make('mypassword'); if (Hash::check('mypassword', $testhash1) && Hash::check('mypassword', $testhash2)) { echo "Password matching"; } else { echo "Password not matching"; } } }
Output
上述代码的输出为 −
Password matching
使用bcrypt()方法
You can also try using the bcrypt() method and test the plain text with hashed one using Hash::check().
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; use Illuminate\Support\Facades\Hash; class StudentController extends Controller { public function index() { $hashedtext = bcrypt('mypassword'); if (Hash::check('mypassword', $hashedtext)) { echo 'Password matches'; } else{ echo 'Password not matching'; } } }
Output
上述代码的输出为 -
Password matches
The above is the detailed content of How to compare two encrypted (bcrypt) passwords in Laravel?. For more information, please follow other related articles on the PHP Chinese website!
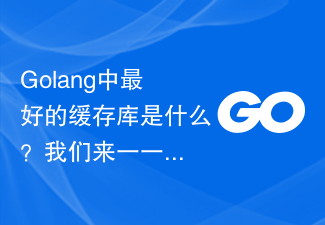
Golang中最好的缓存库是什么?我们来一一比较。在编写Go代码时,经常需要使用缓存,例如存放一些比较耗时的计算结果或者从数据库中读取的数据等,缓存能够大大提高程序的性能。但是,Go语言没有提供原生的缓存库,所以我们需要使用第三方的缓存库。在这篇文章中,我们将一一比较几个比较流行的Go缓存库,找到最适合我们的库。GocacheGocache是一个高效的内存缓
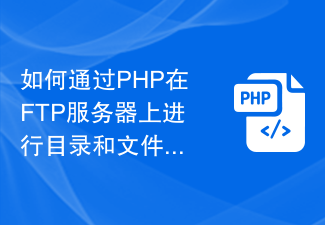
如何通过PHP在FTP服务器上进行目录和文件的比较在web开发中,有时候我们需要比较本地文件与FTP服务器上的文件,以确保两者之间的一致性。PHP提供了一些函数和类来实现这个功能。本文将介绍如何使用PHP在FTP服务器上进行目录和文件的比较,并提供相关的代码示例。首先,我们需要连接到FTP服务器。PHP提供了ftp_connect()函数来建立与FTP服务器
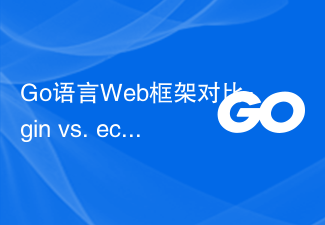
随着Web开发的需求不断增加,各种语言的Web框架也逐渐多样化,Go语言也不例外。在许多Go语言的Web框架中,gin、echo和iris是三个最受欢迎的框架。在这篇文章中,我们将比较这三个框架的优缺点,以帮助您选择适合您的项目的框架。gingin是一个轻量级的Web框架,它具有高性能和灵活性的特点。它支持中间件和路由功能,这使得它非常适合构建RESTful
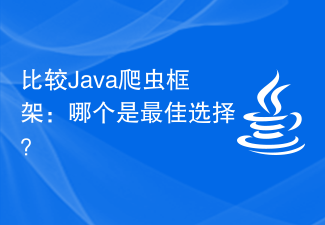
探寻最佳Java爬虫框架:哪个更胜一筹?在当今信息时代,大量的数据在互联网中不断产生和更新。为了从海量数据中提取有用的信息,爬虫技术应运而生。而在爬虫技术中,Java作为一种强大且广泛应用的编程语言,拥有许多优秀的爬虫框架可供选择。本文将探寻几个常见的Java爬虫框架,并分析它们的特点和适用场景,最终找到最佳的一种。JsoupJsoup是一种非常受欢迎的Ja
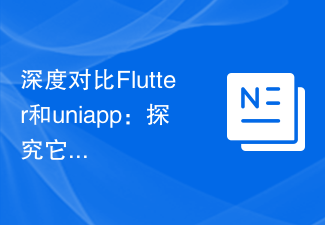
在移动应用开发领域,Flutter和uniapp是两个备受关注的跨平台开发框架。它们的出现使得开发者能够快速且高效地开发同时支持多个平台的应用程序。然而,尽管它们有着相似的目标和用途,但在细节和特性方面存在一些差异。接下来,我们将深入比较Flutter和uniapp,并探讨它们各自的特点。Flutte是由Google推出的开源移动应用开发框架。Flutter
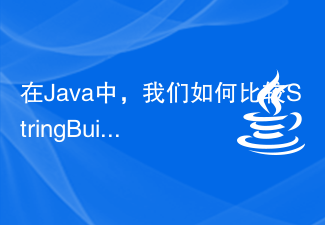
StringBuffer对象通常可以安全地在多线程环境中使用,其中多个线程可能会尝试访问同一个StringBuffer对象同时。StringBuilder是线程安全的StringBuffer类的替代品,它的工作速度要快得多,因为它没有同步>方法。如果我们在单个线程中执行大量字符串操作,则使用此类可以提高性能。示例publicclassCompareBuilderwithBufferTest{ publicstaticvoidmain(String[]a
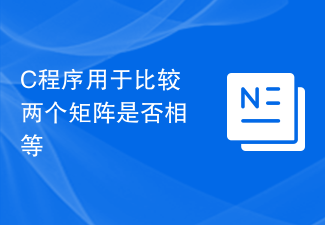
用户必须输入两个矩阵的顺序以及两个矩阵的元素。然后,比较这两个矩阵。如果矩阵元素和大小都相等,则表明两个矩阵相等。如果矩阵大小相等但元素相等不相等,则显示矩阵可以比较,但不相等。如果大小和元素不匹配,则显示矩阵无法比较。程序以下是C程序,用于比较两个矩阵是否相等-#include<stdio.h>#include<conio.h>main(){ intA[10][10],B[10][10]; in
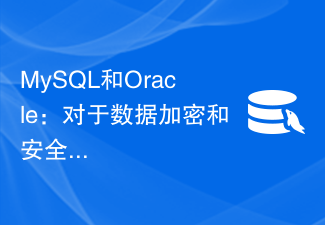
MySQL和Oracle:对于数据加密和安全传输的支持程度比较引言:数据安全在如今的信息时代中变得愈发重要。从个人隐私到商业机密,保持数据的机密性和完整性对于任何组织来说都至关重要。在数据库管理系统(DBMS)中,MySQL和Oracle是两个最受欢迎的选项。在本文中,我们将比较MySQL和Oracle在数据加密和安全传输方面的支持程度,并提供一些代码示例。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
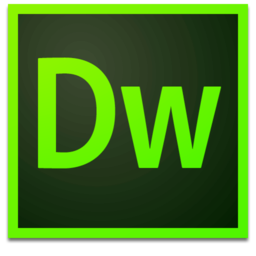
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
