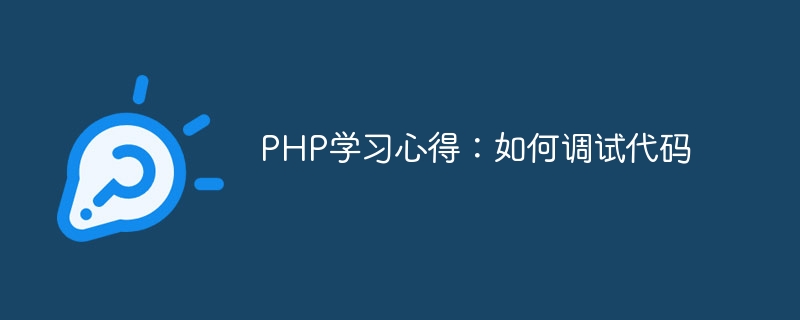
PHP learning experience: How to debug code
As a scripting language widely used in web development, PHP will inevitably encounter various problems in practical applications. When we encounter code problems, a good debugging tool and method is extremely important. This article will introduce some common PHP debugging techniques and tools to help learners find and solve code problems more efficiently.
1. Debugging skills
- Use the var_dump() function: The var_dump() function can output the value and type of the variable to help quickly locate the problem. The following is an example:
<?php
$name = "John";
$age = 25;
var_dump($name);
var_dump($age);
?>
Executing the above code will output the value and type of $name, and the value and type of $age.
- Use the echo() function in conjunction with the exit() function: When we need to output a variable or intermediate result in the code, we can use the echo() function. At the same time, we can also use the exit() function to stop execution at a specific location in the code to view the status of variables under certain circumstances. For example:
<?php
$number1 = 10;
$number2 = 5;
$result = $number1 + $number2;
echo "结果是:" . $result;
exit();
?>
- Utilize logging: Using logging is a good choice when encountering complex problems. We can use file operation functions, such as file_put_contents(), to write debugging information to the log file. Here is an example:
<?php
$number1 = 10;
$number2 = 0;
if($number2 == 0){
file_put_contents("error.log", "除数不能为0");
}
$result = $number1 / $number2;
?>
In the above example, if $number2 is 0, an error message will be written to the error.log file.
- Use try-catch statements for exception handling: When we encounter errors or exceptions in the code, we can use try-catch statements to capture and handle them. The following is an example:
<?php
try {
$number1 = 10;
$number2 = 0;
if($number2 == 0){
throw new Exception("除数不能为0");
}
$result = $number1 / $number2;
echo "结果是:" . $result;
} catch (Exception $e) {
echo "发生错误:" . $e->getMessage();
}
?>
In the above example, if $number2 is 0, an exception will be thrown and caught and handled using catch.
2. Debugging Tools
- Use xdebug: xdebug is a powerful PHP debugging extension that can help us find code problems more quickly and accurately. We can use functions such as step over, step into, and step out to debug the code line by line. At the same time, xdebug also provides some useful functions and variables, such as the xdebug_break() function for setting breakpoints, and the xdebug_var_dump() function for more friendly output of variable information.
- Use PHPStorm: PHPStorm is a powerful PHP development environment that provides a wealth of debugging tools, smart prompts, code navigation and other functions. We can use the debugging function of PHPStorm to single-step debug the code, set breakpoints, view variables and call stacks, etc.
- Use Chrome Developer Tools: Chrome Developer Tools is a powerful web development tool that provides debugging support for PHP scripts. We can set breakpoints in the source code, debug line by line, view variables and call stacks, etc. by opening the debugging panel of the developer tools.
Summary
By learning the PHP debugging skills and tools introduced in this article, we can find and solve code problems more efficiently. Different problems may require the use of different debugging methods and tools, so we need to choose the appropriate method according to the specific situation. At the same time, continuing to learn and practice debugging skills is also very important to improve your development capabilities and problem-solving abilities. I hope this article is helpful for learning PHP debugging!
The above is the detailed content of PHP learning experience: How to debug code. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn