How to use Python for target tracking on pictures
How to use Python to track objects in pictures
Object tracking is one of the important applications in the field of computer vision. It can track specific objects in consecutive frames of image data. Target. Python is a powerful programming language that provides many libraries and tools that make goal tracking relatively easy. In this article, we will introduce how to use Python and the OpenCV library to perform object tracking on images.
First, before we start writing code, we need to install the OpenCV library. It can be installed using the following command:
pip install opencv-python
Next, we will write code in Python to implement target tracking. Below is a simple code example that shows how to use OpenCV to track blue objects in an image.
import cv2 import numpy as np # 定义蓝色的HSV范围 lower_blue = np.array([90, 50, 50]) upper_blue = np.array([130, 255, 255]) # 初始化摄像头 cap = cv2.VideoCapture(0) while True: # 读取摄像头捕获的图像 ret, frame = cap.read() # 将图像从BGR转换为HSV颜色空间 hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV) # 创建一个掩膜,将满足蓝色范围内的像素点设置为白色(255),其余设置为黑色(0) mask = cv2.inRange(hsv, lower_blue, upper_blue) # 对掩膜进行模糊处理,以去除噪声 mask = cv2.blur(mask, (5, 5)) # 找到图像中的轮廓 contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE) if len(contours) > 0: # 找到最大的轮廓 max_contour = max(contours, key=cv2.contourArea) # 计算最小外接矩形 x, y, w, h = cv2.boundingRect(max_contour) # 在图像上绘制矩形 cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2) # 显示图像 cv2.imshow("Tracking", frame) # 按下ESC键退出循环 if cv2.waitKey(1) == 27: break # 释放摄像头和窗口 cap.release() cv2.destroyAllWindows()
In the above code, we first define the blue HSV range. We then initialize the camera and read the images in an infinite loop. In each loop iteration, we convert the image from BGR to HSV, create a mask, and blur the mask to remove noise. Next, we find the contours in the image and find the largest contour. We then calculate the minimum enclosing rectangle and draw that rectangle on the image. Finally, we display the trace results and exit the loop when the ESC key is pressed. Finally, we release the camera and close the window.
Using the above code, we can track the blue objects in the images captured by the camera. Of course, if we want to track objects of other colors, just change the HSV range for blue.
To sum up, Python and OpenCV libraries provide many convenient methods for image processing and target tracking. By writing corresponding code, we can easily track objects of interest in images. I hope this article can help you get started with goal tracking and implement this interesting task in Python.
The above is the detailed content of How to use Python for target tracking on pictures. For more information, please follow other related articles on the PHP Chinese website!
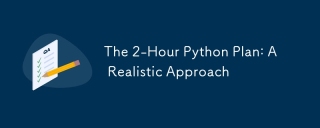
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
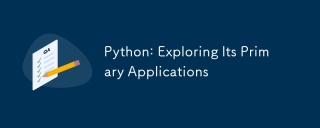
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
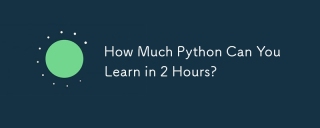
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
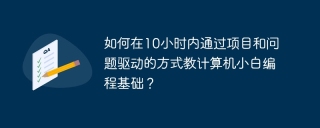
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
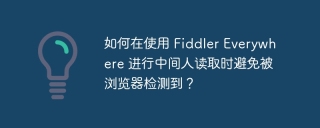
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
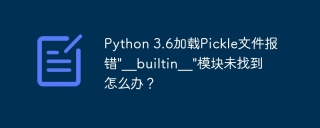
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
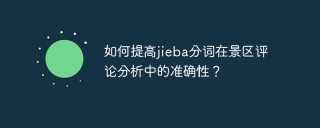
How to solve the problem of Jieba word segmentation in scenic spot comment analysis? When we are conducting scenic spot comments and analysis, we often use the jieba word segmentation tool to process the text...

How to use regular expression to match the first closed tag and stop? When dealing with HTML or other markup languages, regular expressions are often required to...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
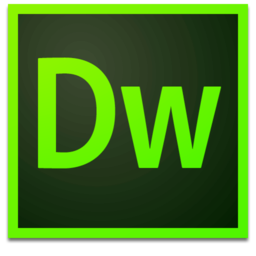
Dreamweaver Mac version
Visual web development tools
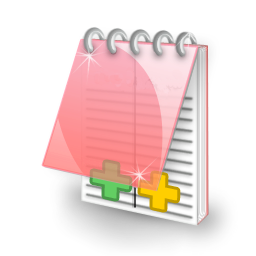
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
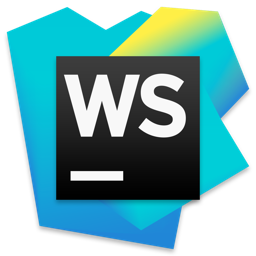
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
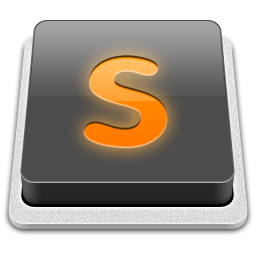
SublimeText3 Mac version
God-level code editing software (SublimeText3)