How to solve: Java graphical interface error: Component display error
How to solve: Java graphical interface error: Component display error
Introduction: Java, as a powerful programming language, is widely popular in graphical interface development. However, in the daily development process, we sometimes encounter the problem of component display errors. This article describes some common error conditions and provides solutions and code examples.
Error situation 1: The component is not displayed
Sometimes, we add a component in the code, but after running the program we find that the component is not displayed. This may be caused by the component not being added to the parent container.
Solution:
Make sure to add the component correctly to the parent container using an appropriate layout manager. Also, remember to use the setVisible(true)
method to make the component visible.
Sample code:
import javax.swing.*; public class ComponentNotDisplayedExample { public static void main(String[] args) { JFrame frame = new JFrame("组件未显示示例"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JButton button = new JButton("点击我"); frame.add(button); // 将按钮添加到父容器中 frame.pack(); frame.setVisible(true); } }
Error situation two: component display misalignment
Sometimes, we may find that the position of the component display is incorrect and deviates from our expected position. This can be caused by using an incorrect layout manager or setting the wrong component position.
Solution:
Make sure to use an appropriate layout manager and set the component's position and bounds correctly. You can use the setBounds(int x, int y, int width, int height)
method to set the position and size of the component.
Sample code:
import javax.swing.*; public class ComponentMisplacedExample { public static void main(String[] args) { JFrame frame = new JFrame("组件显示错位示例"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JButton button = new JButton("点击我"); button.setBounds(100, 100, 100, 50); // 设置按钮的位置和大小 frame.setLayout(null); // 使用绝对布局管理器 frame.add(button); // 将按钮添加到父容器中 frame.setSize(300, 200); frame.setVisible(true); } }
Error situation three: component display overlap
Sometimes, we will find that there is overlap between components, that is, one component covers the display area of other components. This can be caused by using an incorrect layout manager or setting the same component position.
Solution:
Make sure to use an appropriate layout manager and set a different position and bounds for each component to avoid overlapping them.
Sample code:
import javax.swing.*; public class ComponentOverlapExample { public static void main(String[] args) { JFrame frame = new JFrame("组件显示重叠示例"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); JButton button1 = new JButton("按钮1"); button1.setBounds(100, 100, 100, 50); JButton button2 = new JButton("按钮2"); button2.setBounds(200, 100, 100, 50); frame.setLayout(null); frame.add(button1); frame.add(button2); frame.setSize(400, 300); frame.setVisible(true); } }
Conclusion:
In Java graphical interface development, it is common to encounter component display errors. By using layout managers correctly, setting component positions and boundaries, and using appropriate visibility methods, we can effectively solve and prevent these problems. I hope that the solutions and sample codes provided in this article can help readers successfully solve component display errors in Java graphical interfaces.
The above is the detailed content of How to solve: Java graphical interface error: Component display error. For more information, please follow other related articles on the PHP Chinese website!
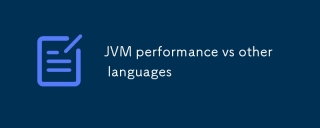
JVM'sperformanceiscompetitivewithotherruntimes,offeringabalanceofspeed,safety,andproductivity.1)JVMusesJITcompilationfordynamicoptimizations.2)C offersnativeperformancebutlacksJVM'ssafetyfeatures.3)Pythonisslowerbuteasiertouse.4)JavaScript'sJITisles

JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunonanyplatformwithaJVM.1)Codeiscompiledintobytecode,notmachine-specificcode.2)BytecodeisinterpretedbytheJVM,enablingcross-platformexecution.3)Developersshouldtestacross
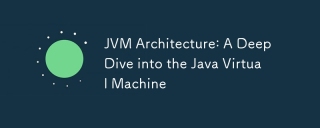
TheJVMisanabstractcomputingmachinecrucialforrunningJavaprogramsduetoitsplatform-independentarchitecture.Itincludes:1)ClassLoaderforloadingclasses,2)RuntimeDataAreafordatastorage,3)ExecutionEnginewithInterpreter,JITCompiler,andGarbageCollectorforbytec
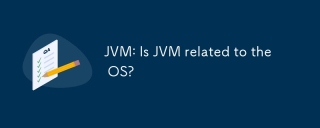
JVMhasacloserelationshipwiththeOSasittranslatesJavabytecodeintomachine-specificinstructions,managesmemory,andhandlesgarbagecollection.ThisrelationshipallowsJavatorunonvariousOSenvironments,butitalsopresentschallengeslikedifferentJVMbehaviorsandOS-spe
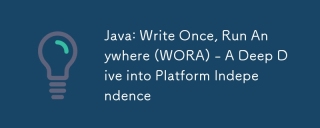
Java implementation "write once, run everywhere" is compiled into bytecode and run on a Java virtual machine (JVM). 1) Write Java code and compile it into bytecode. 2) Bytecode runs on any platform with JVM installed. 3) Use Java native interface (JNI) to handle platform-specific functions. Despite challenges such as JVM consistency and the use of platform-specific libraries, WORA greatly improves development efficiency and deployment flexibility.
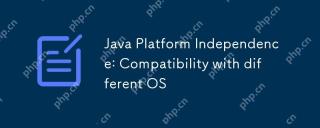
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
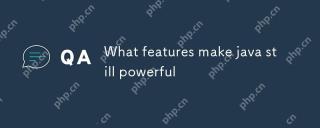
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
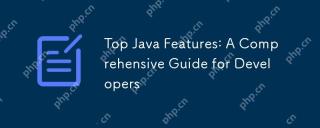
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
