Solve PHP error: circular dependency problem between classes
Solve PHP error: circular dependency problem between classes
In PHP development, dependencies between classes are very common. But sometimes, when there are circular dependencies between two or more classes, it may cause code errors. This article will discuss the causes of circular dependencies and provide some solutions to avoid this problem.
Circular dependency means that two or more classes refer to each other without a clear dependency chain. In this case, when we try to instantiate one of the classes, the PHP engine cannot resolve this complex dependency, causing an error. The following is a simple sample code:
// ClassA.php class ClassA { public function __construct(ClassB $classB) { $this->classB = $classB; } } // ClassB.php class ClassB { public function __construct(ClassA $classA) { $this->classA = $classA; } } // index.php require_once 'ClassA.php'; require_once 'ClassB.php'; $classA = new ClassA(new ClassB);
In the above sample code, ClassA and ClassB depend on each other's instances through the constructor. When we try to instantiate ClassA, since the constructor of ClassA requires an instance of ClassB, and the constructor of ClassB requires an instance of ClassA, this creates a circular dependency.
In order to solve this problem, we can use the following methods:
- Refactor the code: If possible, we should try to avoid circular dependencies. You can refactor the code to change the dependency between two classes to a one-way dependency, or extract the co-dependent code into an independent class.
- Lazy loading: You can use lazy loading to solve circular dependencies. We use a dependency injection container in the constructor of the class to delay the execution of the instantiation work of the class that needs to be instantiated. For example, we can use the
set
method to inject dependencies instead of using them directly in the constructor.
class ClassA { public function setClassB(ClassB $classB) { $this->classB = $classB; } } class ClassB { public function setClassA(ClassA $classA) { $this->classA = $classA; } } $classA = new ClassA; $classB = new ClassB; $classA->setClassB($classB); $classB->setClassA($classA);
- Interfaces and abstract classes: Using interfaces and abstract classes can reduce the coupling between classes, thereby avoiding circular dependencies. Define dependencies between classes by defining interfaces or abstract classes instead of directly relying on concrete classes.
interface InterfaceA { public function doSomething(); } class ClassA implements InterfaceA { public function __construct(InterfaceB $classB) { $this->classB = $classB; } public function doSomething() { // do something } } interface InterfaceB { public function doSomething(); } class ClassB implements InterfaceB { public function __construct(InterfaceA $classA) { $this->classA = $classA; } public function doSomething() { // do something } } $classA = new ClassA(new ClassB);
To summarize, circular dependency is a common problem that will cause code errors in PHP development. In order to solve the circular dependency, we can avoid this problem by refactoring the code, lazy loading, and using interfaces and abstract classes. I hope the solutions provided in this article can help you solve the circular dependency problem in PHP errors.
The above is the detailed content of Solve PHP error: circular dependency problem between classes. For more information, please follow other related articles on the PHP Chinese website!
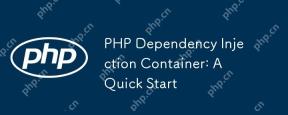
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
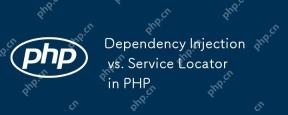
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
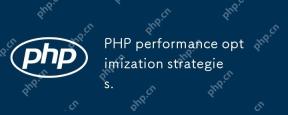
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
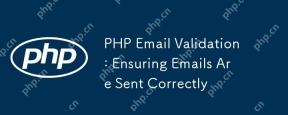
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
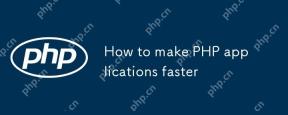
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
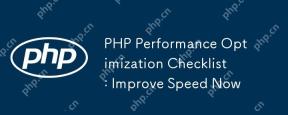
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
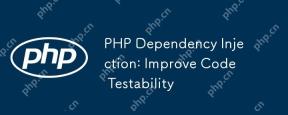
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
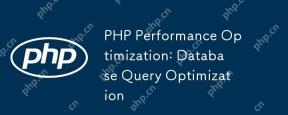
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
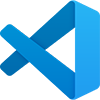
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
